How to convert a string to lower or upper case in Ruby
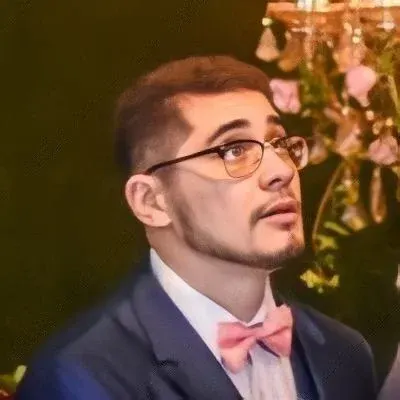

🌟 Conquering Case Conversions in Ruby: Lower or Upper? No Problem! 🌟
Are you feeling puzzled about converting a string to lower or upper case in Ruby? Worry no more, my friend! In this blog post, I'll guide you through the wild world of string case conversions and show you some straightforward solutions to your dilemma. So put on your coding hat and let's dive right in!
The Challenge: String Case Conversion 🔄
So, you've got a string in Ruby, and you want to convert it to either lower or upper case – a common situation we all encounter while working with strings. Let's start this adventure by exploring a few common stumbling blocks along the way.
The Case Clash: Common Issues You Might Encounter 🚧
1️⃣ Issue: Unexpected Capitalization
Sometimes, you might discover that your string's capitalization is unexpectedly tricky. Perhaps it's an all-caps, partially capitalized, or even a mixture of both. These inconsistencies can make the language's built-in case conversion methods less reliable.
2️⃣ Issue: Encoding Fumbles
Another challenge you might face is dealing with different character encodings. Ruby supports various encodings, and working with strings in different encodings can sometimes lead to unexpected behavior during your case conversion process.
3️⃣ Issue: Special Characters Shenanigans
Lastly, special characters, like diacritics or non-ASCII characters, might not play nice during case conversions. Ruby's default case conversion methods are designed to handle ASCII characters, so you might need to take extra steps to deal with these special characters gracefully.
The Solutions: Taming the Case Conversion Jungle 🦁
Now that we've identified some potential hurdles, it's time to equip ourselves with the solutions to overcome them. Ruby provides various methods to handle string case conversions, each offering unique benefits.
Solution 1: upcase
and downcase
Methods
Ruby's upcase
and downcase
methods are your faithful companions when converting strings to upper or lower case. They handle ASCII characters with ease and are the simplest options for case conversions.
# Converting strings to upper case
string = "Hello, World!"
uppercase_string = string.upcase
# Output: "HELLO, WORLD!"
# Converting strings to lower case
string = "HELLO, WORLD!"
lowercase_string = string.downcase
# Output: "hello, world!"
These methods work great if you don't have to worry about special characters or different encodings.
Solution 2: capitalize
Method
If you only want to capitalize the first letter of a string and keep the rest unchanged, the capitalize
method is there to save the day.
string = "hello, world!"
capitalized_string = string.capitalize
# Output: "Hello, world!"
Note that capitalize
converts all other letters in the string to lower case.
Solution 3: Unicode
Gem
To tackle those tricky special characters and various encodings, the unicode
gem comes to your rescue. It extends Ruby's built-in string case conversion methods, making them more robust and suitable for handling Unicode characters.
require 'unicode'
string = "Café au Lait"
unicode_lowercase_string = Unicode::downcase(string)
unicode_uppercase_string = Unicode::upcase(string)
# Output: "café au lait" and "CAFÉ AU LAIT"
To use the unicode
gem, remember to install it first by running gem install unicode
.
The Grand Finale: Engage with String Case Conversions! 🎉
Now that you're armed with knowledge about turning strings into colorful cases, it's time to put your newfound skills to the test! Try converting strings in different ways, explore special characters, tackle encoding variations, and discover the power of Unicode conversions in your Ruby projects.
Share your adventures, challenges, or creative solutions in the comments below. Let's unleash the magic together and conquer string case conversions!
Thank you for joining me on this thrilling quest. Until we meet again, happy coding! 💻✨
Take Your Tech Career to the Next Level
Our application tracking tool helps you manage your job search effectively. Stay organized, track your progress, and land your dream tech job faster.
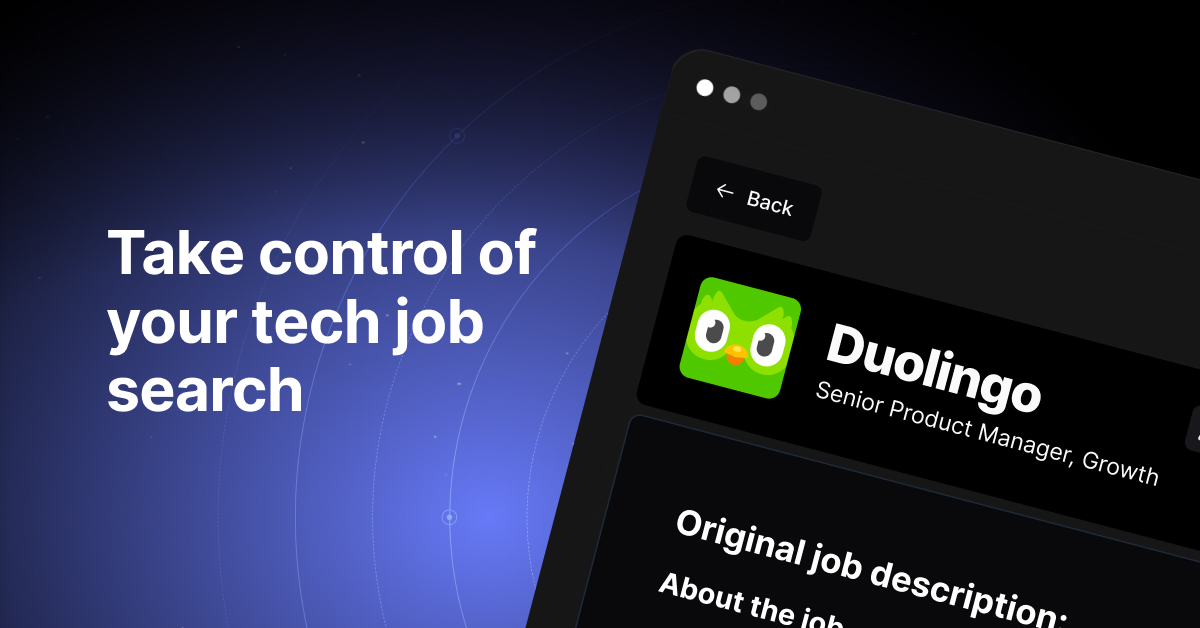