How to check if a specific key is present in a hash or not?
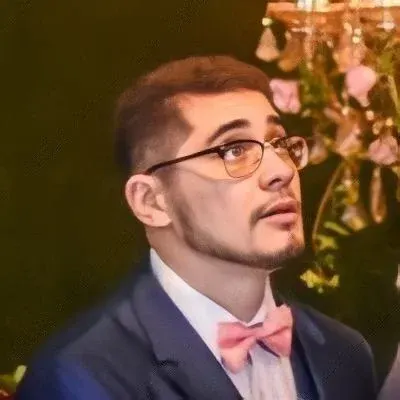
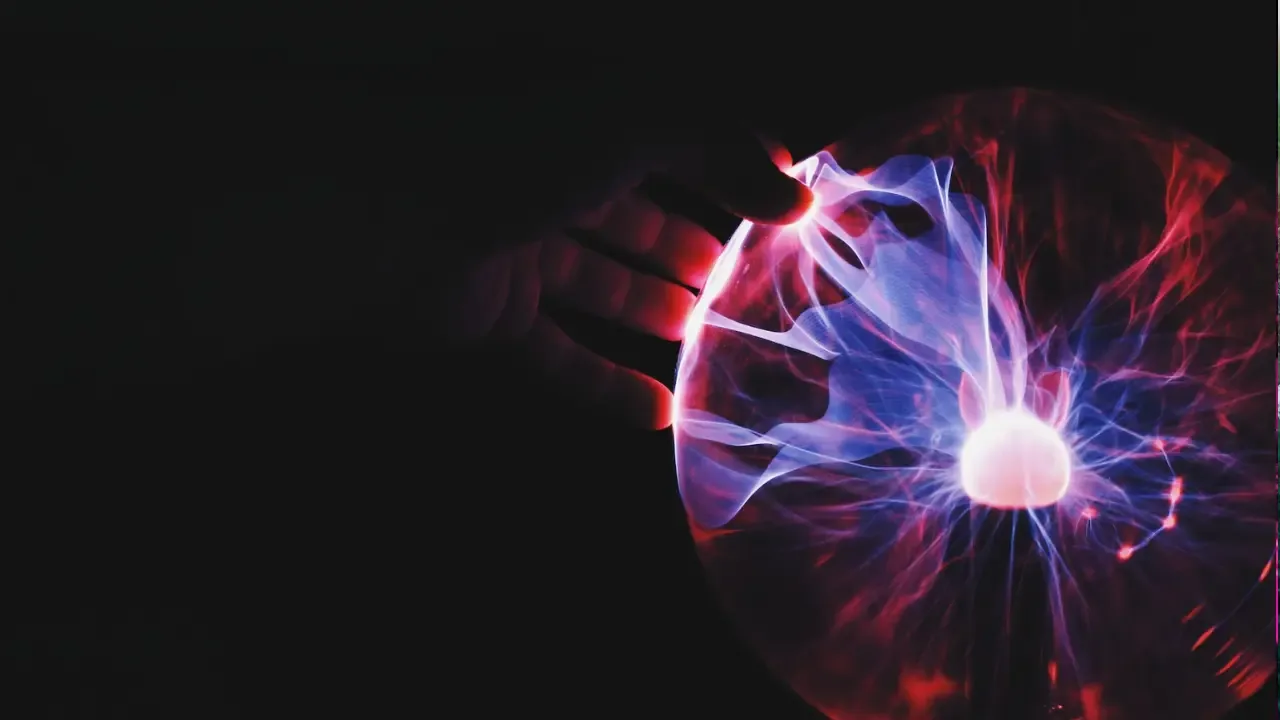
🔍 How to Check if a Specific Key is Present in a Hash?
So, you want to know if a particular key exists in a hash? And not just any key, but the "user" key? I got you covered! 🧐
Let's assume you are working with a session hash in your Ruby code, and you need to check if the "user" key is present or not. But here's the catch - you only want to check the key itself, not its value. Don't worry, I've got a simple solution for you. 💡
To determine whether a specific key exists in a hash, you can use the key?
or include?
methods. These methods return a boolean value indicating the presence of the specified key in the hash.
Here's an example:
session = { "user" => "JohnDoe", "role" => "admin", "logged_in" => true }
if session.key?("user") # or session.include?("user")
puts "User key exists in the session hash!"
else
puts "User key does not exist in the session hash."
end
In this example, we have a session hash containing key-value pairs, such as "user" with the value "JohnDoe," "role" with the value "admin," and "logged_in" with the value true.
By using either the key?("user")
or include?("user")
method, we check if the "user" key exists in the session hash. If it does, we display the message "User key exists in the session hash!" Otherwise, we display "User key does not exist in the session hash."
Easy, right? 🎉
It's important to note that both key?
and include?
methods perform the same function of checking key existence in a hash. You can use either one based on your preference or code style.
But what if you want to avoid duplicating code and have a more concise approach? Let me introduce you to the dig
method. 😏
The dig
method retrieves the value associated with the specified key in a nested hash. If the key doesn't exist, it returns nil
. So, in order to check the existence of the "user" key, you can write:
if session.dig("user")
puts "User key exists in the session hash!"
else
puts "User key does not exist in the session hash."
end
In this example, the dig("user")
method is used to retrieve the value associated with the "user" key. Since we are only interested in checking the existence of the key, we can use it in an if
statement directly.
Now that you know how to check if a specific key is present in a hash, go ahead and use this knowledge to level up your Ruby coding skills! 😎
Feel free to leave a comment with your thoughts or any other questions you might have. Happy coding! 💻
Don't forget to follow us on Twitter @TechBlog for more exciting tech tips and tricks! 🐦
Take Your Tech Career to the Next Level
Our application tracking tool helps you manage your job search effectively. Stay organized, track your progress, and land your dream tech job faster.
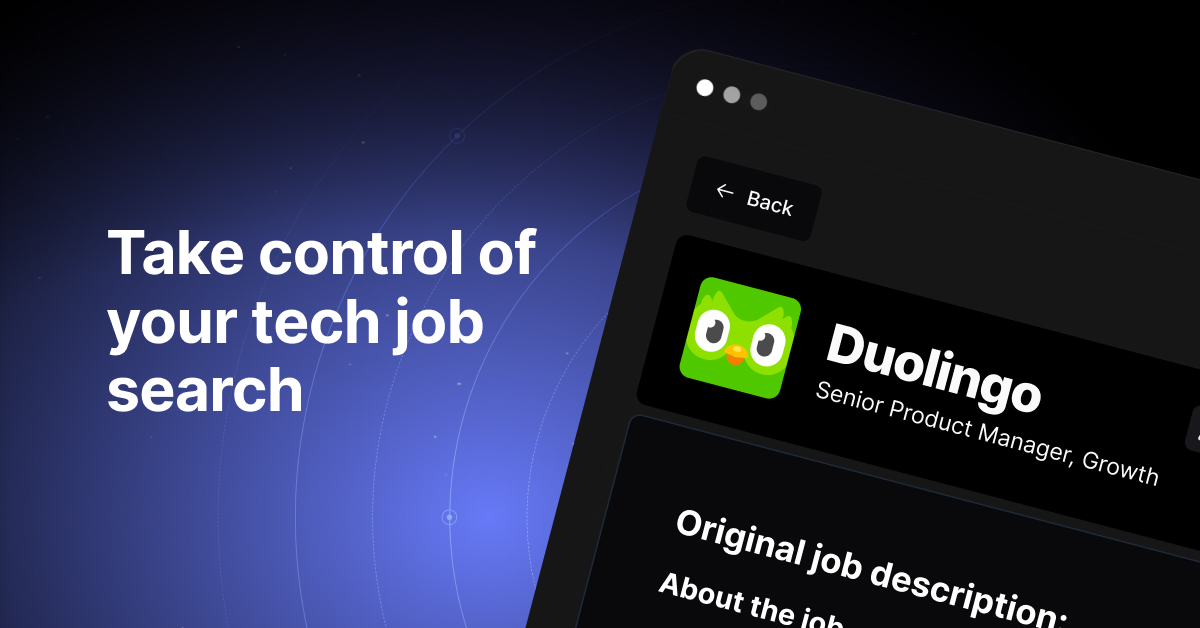