How do I use the conditional operator (? :) in Ruby?
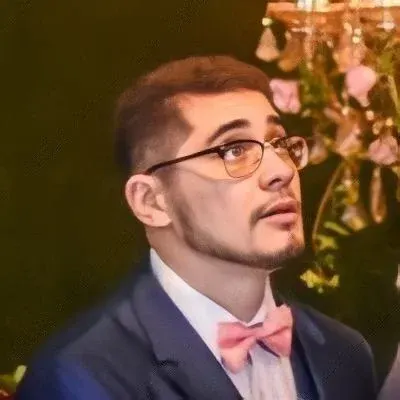
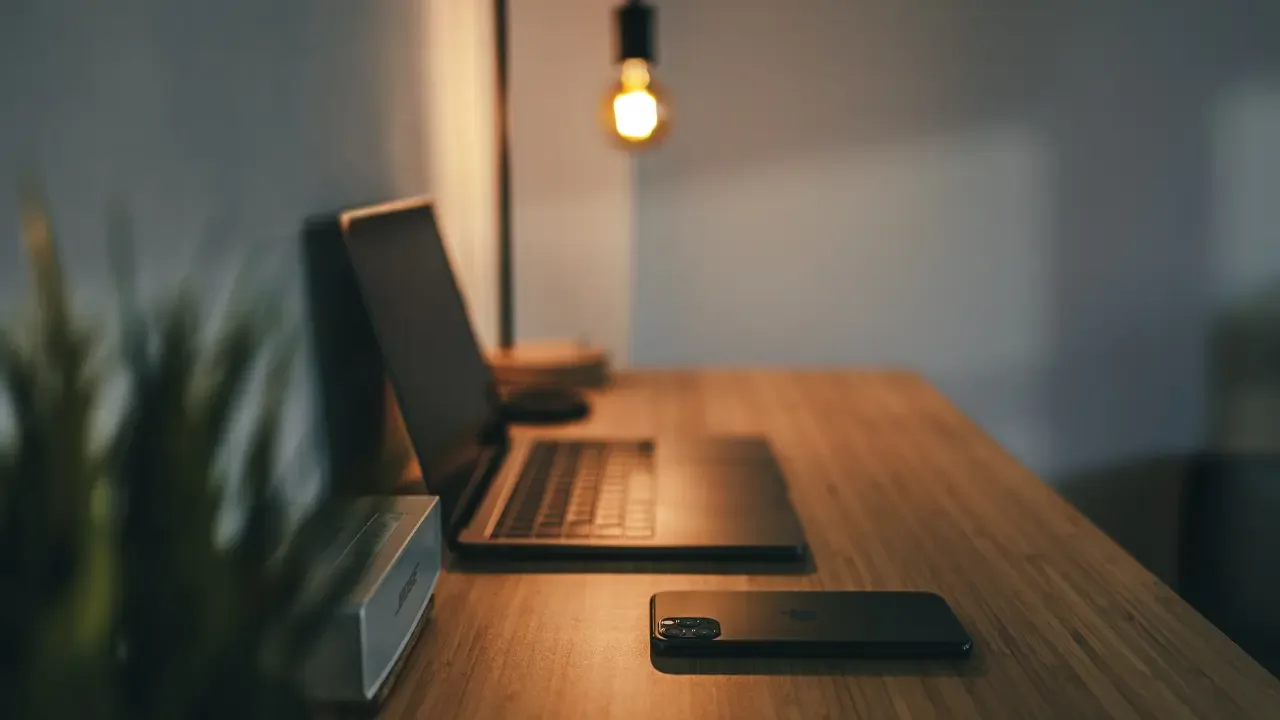
Mastering the Conditional Operator in Ruby: A Guide to Simplify Your Code ✨🔍
So, you've stumbled upon the mysterious ?:
operator in Ruby and you're probably wondering, "How can this little symbol help me write cleaner and more efficient code?" 🧐
Well, fret not, my friend! In this blog post, I'll break down the conditional operator (?:
) in Ruby, explain its syntax and purpose, address any common issues you might encounter, and provide easy solutions to make your code sparkle. 💎✨
But first, let's understand the syntax of the conditional operator in Ruby:
condition ? true_value : false_value
The condition
is evaluated, and if it returns true
, the expression before the :
is executed. Otherwise, the expression after the :
is executed. It's like a compact if-else
statement wrapped in a neat little package. Pretty cool, right? 😎
Now let's dive into the example you provided:
<% question = question.size > 20 ? question.question.slice(0, 20) + "..." : question.question %>
In this case, if the size of the question
is greater than 20 characters, the expression before the :
will be executed. It takes the first 20 characters of the question
using the slice
method and appends "..."
to indicate truncation. If the size is less than or equal to 20, the expression after the :
is executed. It simply returns the original question
. 🗣️💬
However, there's a small issue with this example. As the code is embedded within <%
and %>
, I assume you're using it within an ERB template. In that case, you should consider escaping the resulting HTML, keeping your users safe from potential XSS (cross-site scripting) attacks. One way to accomplish this is by using the html_escape
method:
<% question = question.size > 20 ? ERB::Util.html_escape(question.question.slice(0, 20) + "...") : ERB::Util.html_escape(question.question) %>
By utilizing ERB::Util.html_escape
, we ensure that any special characters are properly encoded, making your application secure and your users happy. 😌🔒
Now that we've addressed that, let me give you a few more tips to level up your skills with the conditional operator:
1. Make it readable: While the conditional operator helps in keeping code concise, it's important not to sacrifice readability. Use it judiciously and consider breaking complex expressions into multiple lines or using traditional if-else
statements when it enhances code clarity.
2. Handle nil cases gracefully: When using the conditional operator, remember to handle cases where the condition evaluates to nil
gracefully. Unchecked nil values can lead to errors. Adding a default value or handling nil explicitly can save you from potential headaches! 🤕
3. Embrace the elegance of the ternary operator: The conditional operator can make your code more elegant and succinct, but don't overuse it. Sometimes, a regular if-else
statement might be more appropriate, especially when dealing with complex conditions or multiple branches. Strive for a balance between conciseness and clarity.
And there you have it! You're now equipped with the knowledge to leverage the conditional operator (?:
) in Ruby effectively. Harness its power to write cleaner, more concise code that even non-programmers would find readable! 🚀🌟
So, why not experiment with the conditional operator right away? Share your code snippets, ask questions, or share your favorite use cases in the comments below! Let's learn and grow together. 😄✍️
Remember, simplicity is the ultimate sophistication. Keep coding, keep learning, and keep spreading the Ruby love! ❤️
Take Your Tech Career to the Next Level
Our application tracking tool helps you manage your job search effectively. Stay organized, track your progress, and land your dream tech job faster.
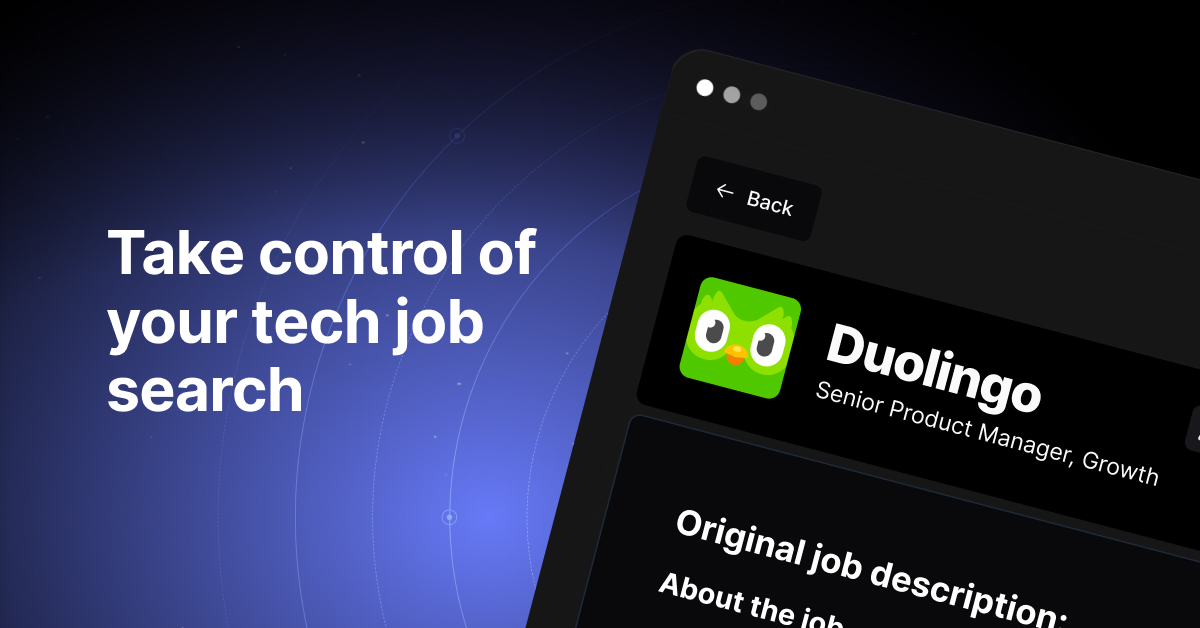