How do I parse a YAML file in Ruby?
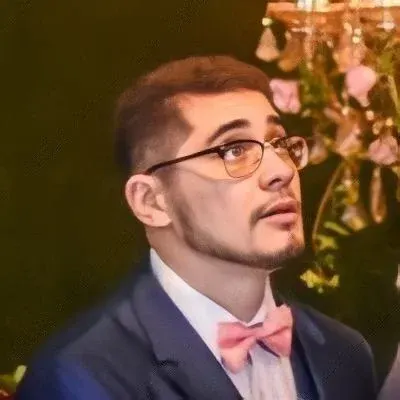
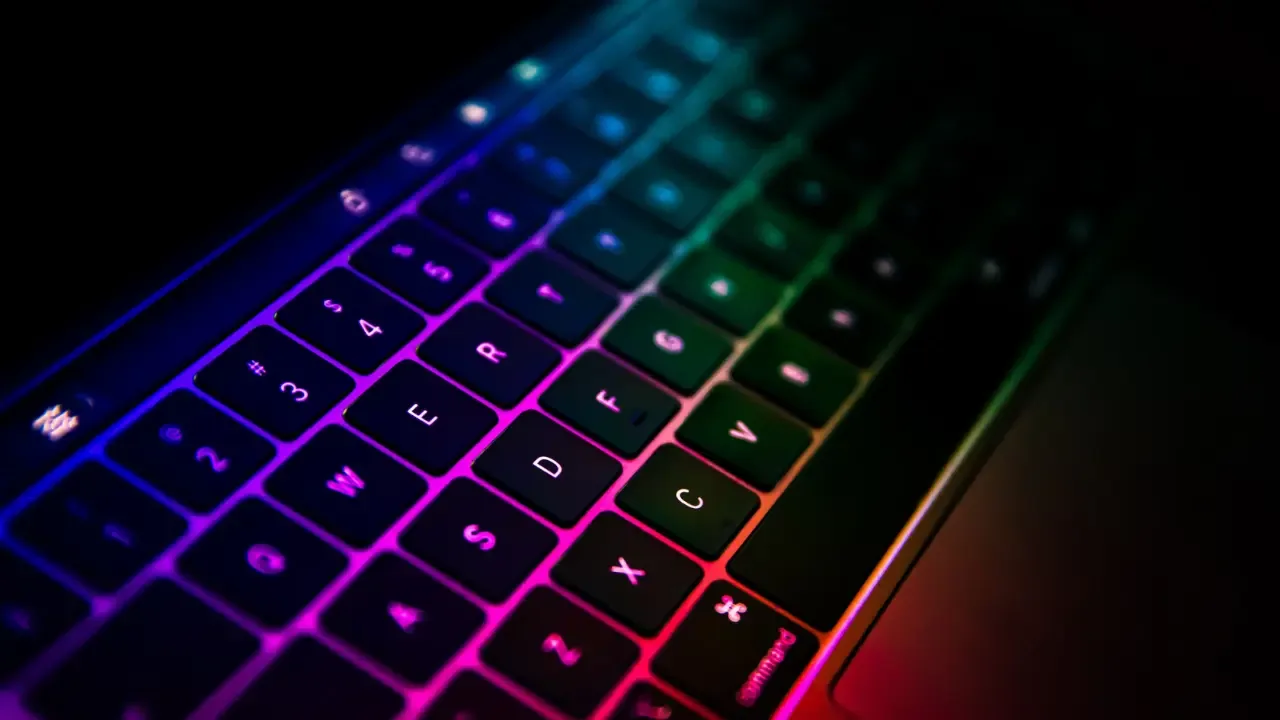
How to Parse a YAML File in Ruby: A Beginner's Guide
Are you struggling with parsing a YAML file in Ruby? 😕 Don't worry, you're not alone! Many developers encounter issues when trying to extract data from YAML files. In this guide, I'll walk you through the process step-by-step and provide easy solutions to common problems. By the end, you'll be a YAML parsing pro! 🥳
Understanding the Problem
Let's start by understanding the problem at hand. The code provided seems correct, but it throws an error stating that the value is nil
. This means that the variable @custom_asset_packages_yml
is not being assigned the YAML data correctly.
Solution 1: Verify YAML File Existence
To begin, we need to ensure that the YAML file you're trying to parse actually exists. The code snippet you provided checks if the file exists, but let's make it more explicit and handle a scenario where the file doesn't exist:
yaml_file_path = "#{RAILS_ROOT}/config/asset_packages.yml"
if File.exist?(yaml_file_path)
@custom_asset_packages_yml = YAML.load_file(yaml_file_path)
else
# Handle the case where the file doesn't exist
end
By using the File.exist?
method, we check if the YAML file exists before attempting to parse it. This will help prevent any nil
value errors in the future.
Solution 2: Accessing Nested Data
Now that we have successfully loaded the YAML file, we can move on to extracting the data. The specific structure of your YAML file suggests that javascripts
is an array of hashes, where each hash contains fo_global
as the key and an array as its value.
To access the nested data, we need to iterate through the arrays and hashes:
if !@custom_asset_packages_yml.nil?
@custom_asset_packages_yml['javascripts'].each do |js|
js['fo_global'].each do |script|
# Do something with each script
end
end
end
By using nested iterations, we can access each script individually and perform any necessary operations.
Call-to-Action: Share Your Experience!
Congratulations! You now know how to parse a YAML file in Ruby. 🎉 I hope this guide has helped you overcome any difficulties you were facing. Now it's your turn to share your experience! Have you encountered any other challenges when working with YAML files? Do you have any additional tips to share with the Ruby community? Leave a comment below and let's start a conversation! 💬✨
Remember, knowledge grows when shared. So, spread the word and help others in their YAML parsing journey. Happy coding! 💻🚀
Take Your Tech Career to the Next Level
Our application tracking tool helps you manage your job search effectively. Stay organized, track your progress, and land your dream tech job faster.
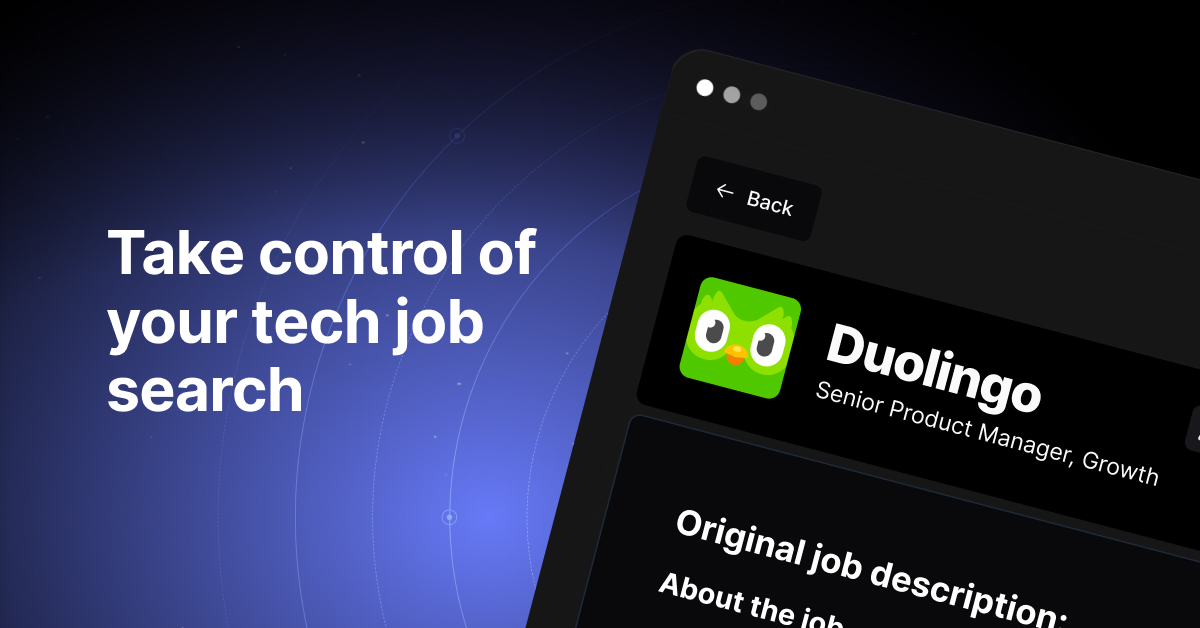