Difference between map and collect in Ruby?
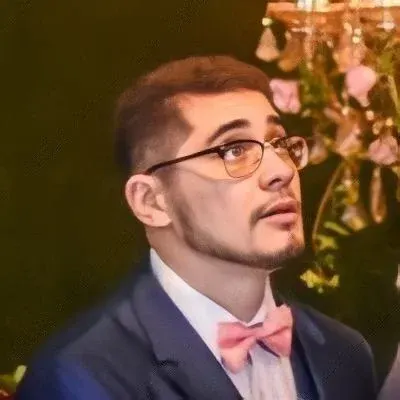
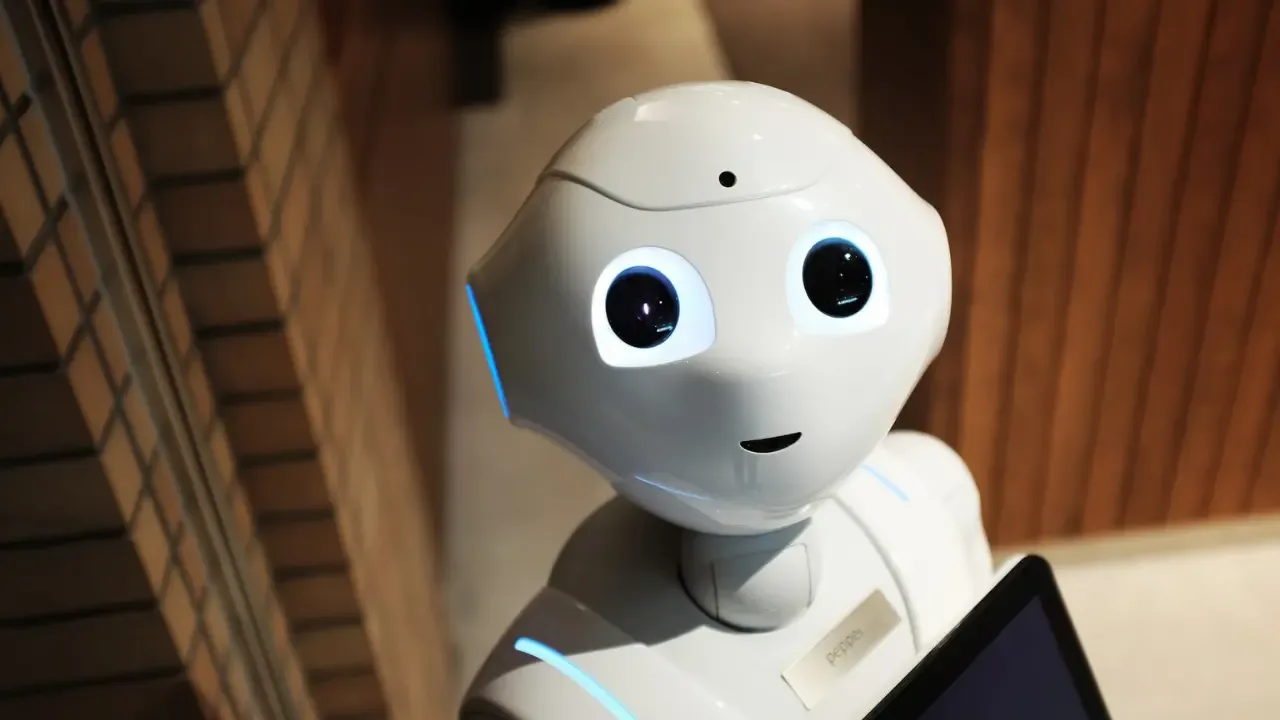
Map vs Collect: What's the Difference?
š¤ Have you ever wondered what the difference is between using map
and collect
on an array in Ruby/Rails? š¤·āāļø If so, you're not alone! Many developers have found themselves searching for a clear answer, only to come across conflicting opinions and incomplete explanations. But fear not! We're here to set the record straight and help you understand the nuances between these two methods.
Understanding the Basics
Before diving into the differences, let's first understand the common ground. Both map
and collect
are powerful methods that allow you to iterate over an array and perform some operation on each element. The beauty of these methods lies in their ability to transform and manipulate data efficiently.
āļø A quick reminder: In Ruby, map
and collect
are synonymous. They can be used interchangeably without causing any discrepancies in your code. So, no need to worry about using one over the other based on technicalities!
The Argument for Use Case
The main difference between map
(or collect
, if you prefer) and other enumerable methods lies in their primary use case. While both methods iterate over an array and perform the same operation on each element, the key distinction comes down to what you intend to do with the results.
š Use "map" for Transformation
The map
method is the go-to choice when you want to transform each element of the array into something else. It's ideal for cases where you need to create a new array by applying some logic or modification to each element.
For example, let's say you have an array of numbers and you want to square each of them:
numbers = [1, 2, 3, 4, 5]
squared_numbers = numbers.map { |num| num ** 2 }
puts squared_numbers # Output: [1, 4, 9, 16, 25]
By using map
, we transformed each number in the original array to its squared equivalent, resulting in a new array of squared numbers.
š Use "collect" for Side Effects
On the other hand, the collect
method is typically used when you want to perform some operation on each element but also have side effects, such as modifying the original array or updating external values.
Let's consider a scenario where you want to double each number in the array and update the original array itself:
numbers = [1, 2, 3, 4, 5]
numbers.collect! { |num| num * 2 }
puts numbers # Output: [2, 4, 6, 8, 10]
In this example, we used collect!
(with an exclamation mark) to denote that the original array is modified directly. Each number in the array was doubled, resulting in the update of the original array itself.
Performance Considerations
You might be wondering if there are any performance differences between map
and collect
. Well, the good news is that both methods perform similarly under the hood. Ruby's implementation treats them as identical methods, so you won't notice any significant performance discrepancies between the two.
ā ļø However, it's essential to consider the potential side effects of using collect!
(with an exclamation mark) ā since it modifies the original array directly, it can lead to unexpected behavior if not used carefully.
Conclusion
To summarize, the difference between map
and collect
in Ruby is primarily semantic, with a slight variation in use case. Use map
when your intention is to transform each element and create a new array, while collect
is better suited for modifying the original array or having side effects.
š” Remember, when in doubt, go with your coding style or personal preference! The two methods are essentially the same, so choose the one that aligns best with your coding habits.
We hope this explanation clears up any confusion and helps you confidently choose between map
and collect
in your future Ruby endeavors!
š Now it's your turn! Have you encountered any tricky scenarios where choosing between map
and collect
became challenging? Share your thoughts, experiences, and questions in the comments below! Let's dive deeper into this topic together. š¬
Take Your Tech Career to the Next Level
Our application tracking tool helps you manage your job search effectively. Stay organized, track your progress, and land your dream tech job faster.
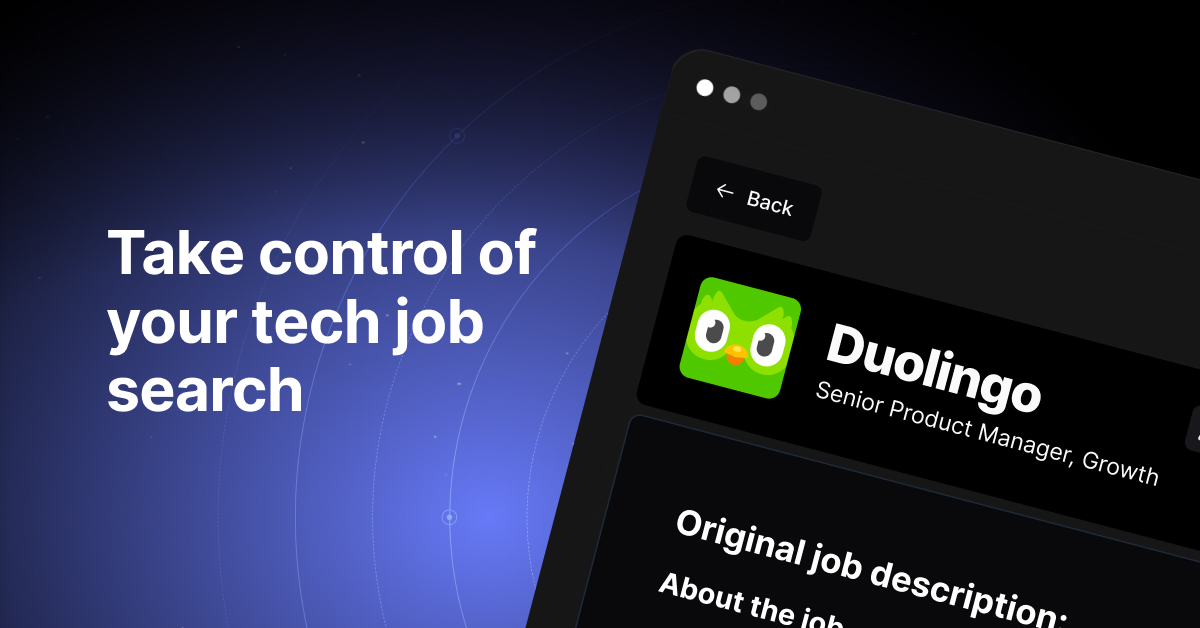