Difference between a class and a module
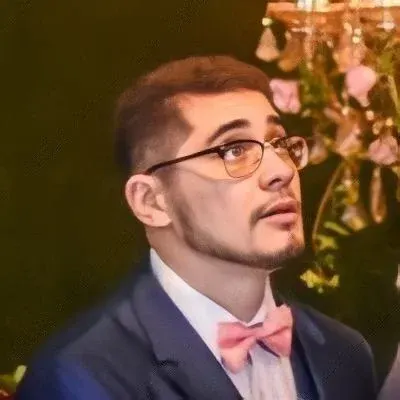
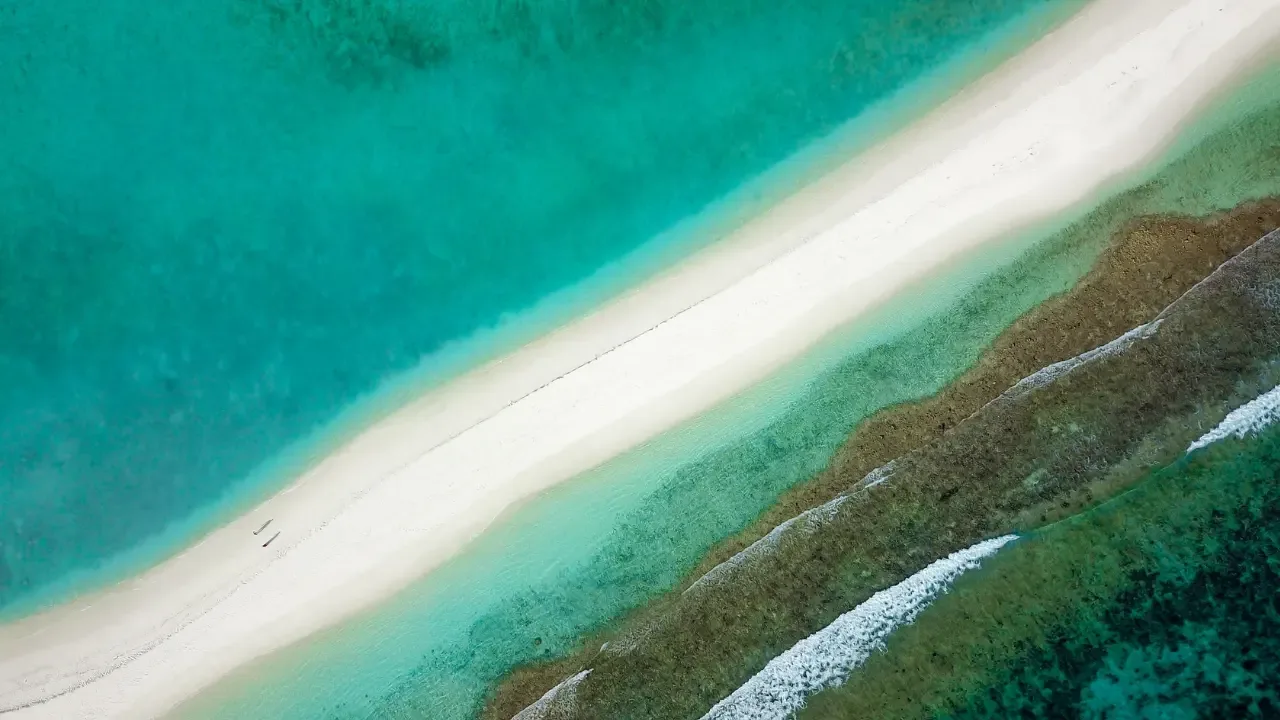
The Class vs. Module Dilemma: Decoding the Mystery! 💡
Are you feeling a little lost in the world of Ruby after coming from Java? 🤔 Don't worry, my friend! I've got your back. 😎 Let's unravel the enigma of classes and modules together! 🧩
The Basics: What's a Class and What's a Module? 📚
In Ruby, a class is like a blueprint for creating objects. It defines the properties and behaviors that the objects of that class can have. It's a fundamental building block for organizing and defining objects in your code. 🏗️
On the other hand, a module can be seen as a container for grouping similar methods or constants. Unlike a class, you can't create objects directly from a module. Instead, modules act as helpers or mixins to provide shared functionality to classes. 🎁
When to Use a Class? 🏢
Classes are commonly used when you want to create multiple instances of objects with similar behavior. They encapsulate the state and behavior required for those objects and help keep your code structured and maintainable. 🚀
For example, imagine you're building an application to manage students. Each student has a name, age, and a set of grades. By creating a Student
class, you can initialize multiple student objects, each with their own unique name, age, and set of grades. Cool, right? 😎
Here's a code snippet to give you a better idea:
class Student
attr_accessor :name, :age, :grades
def initialize(name, age, grades)
@name = name
@age = age
@grades = grades
end
def average_grade
grades.sum / grades.length
end
end
When to Shake Things Up with a Module? 🎉
Modules are like the spice that adds flavor to your code! 🌶️ They provide a way to share methods and constants across multiple classes without the need for inheritance. If you have functionality that can be shared among different classes, a module is your go-to solution. 🤝
Let's say you're developing a game with different characters, like superheroes and villains. Both heroes and villains can perform special moves, so creating a SpecialMove
module allows you to share this functionality with both the Hero
and Villain
classes. Isn't that super powerful? 💥
Check out this example:
module SpecialMove
def perform_special_move
puts "Performing special move!"
end
end
class Hero
include SpecialMove
# more hero-related code...
end
class Villain
include SpecialMove
# more villain-related code...
end
The Big Difference: Class vs. Module 🤔
So, what's the main difference between a class and a module? It all boils down to object instantiation. You can create instances of a class and use them to represent real-world objects. Modules, on the other hand, are used to provide shared functionality and cannot be instantiated directly. 🚫
Final Thoughts and Your Homework! 🎓
Now that you’ve grasped the basics of classes and modules, it's time to embrace the power of Ruby with confidence! 💪
Your Homework: Go ahead and take some time to play around with classes and modules. Create new classes, define methods, include modules, and experiment with the code. That's the best way to solidify your understanding and unlock your Ruby coding skills! 🚀
Don't hesitate to share your experiences or ask any questions in the comments below. Let's keep this conversation going! Together, we'll become Ruby masters! 💎
Keep calm and code on! 💻✨
Take Your Tech Career to the Next Level
Our application tracking tool helps you manage your job search effectively. Stay organized, track your progress, and land your dream tech job faster.
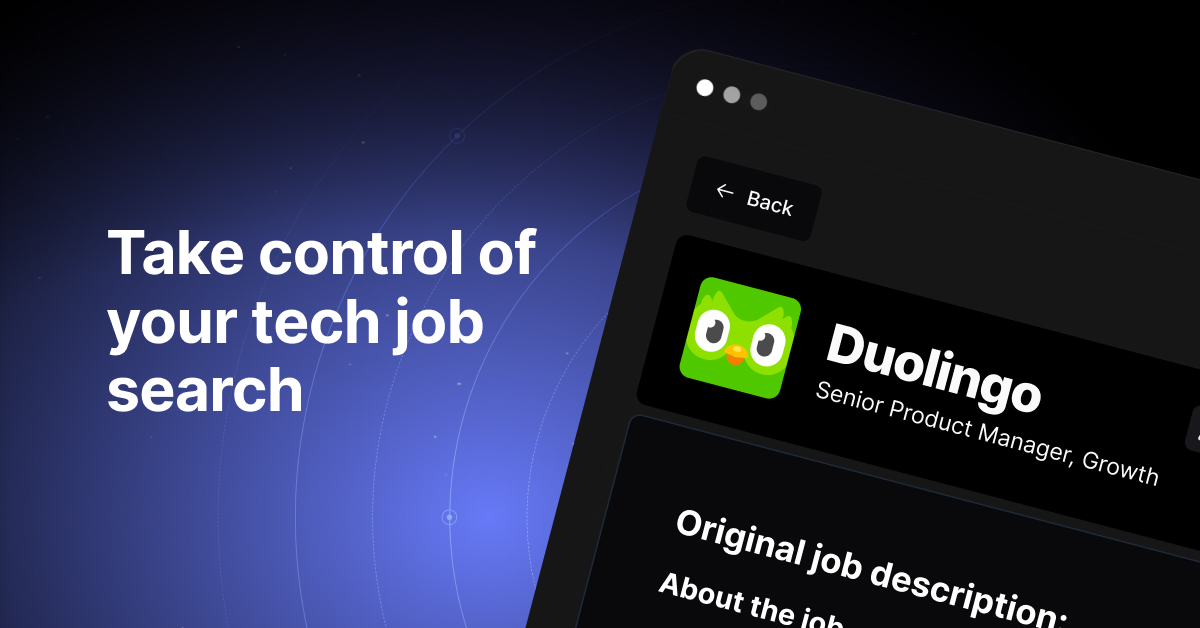