class << self idiom in Ruby
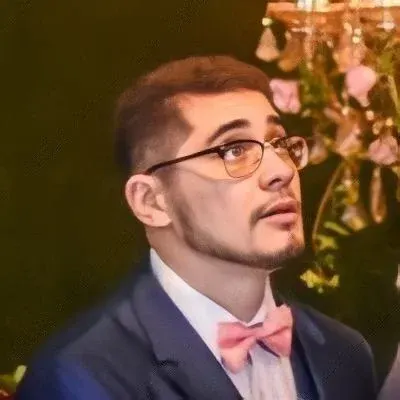
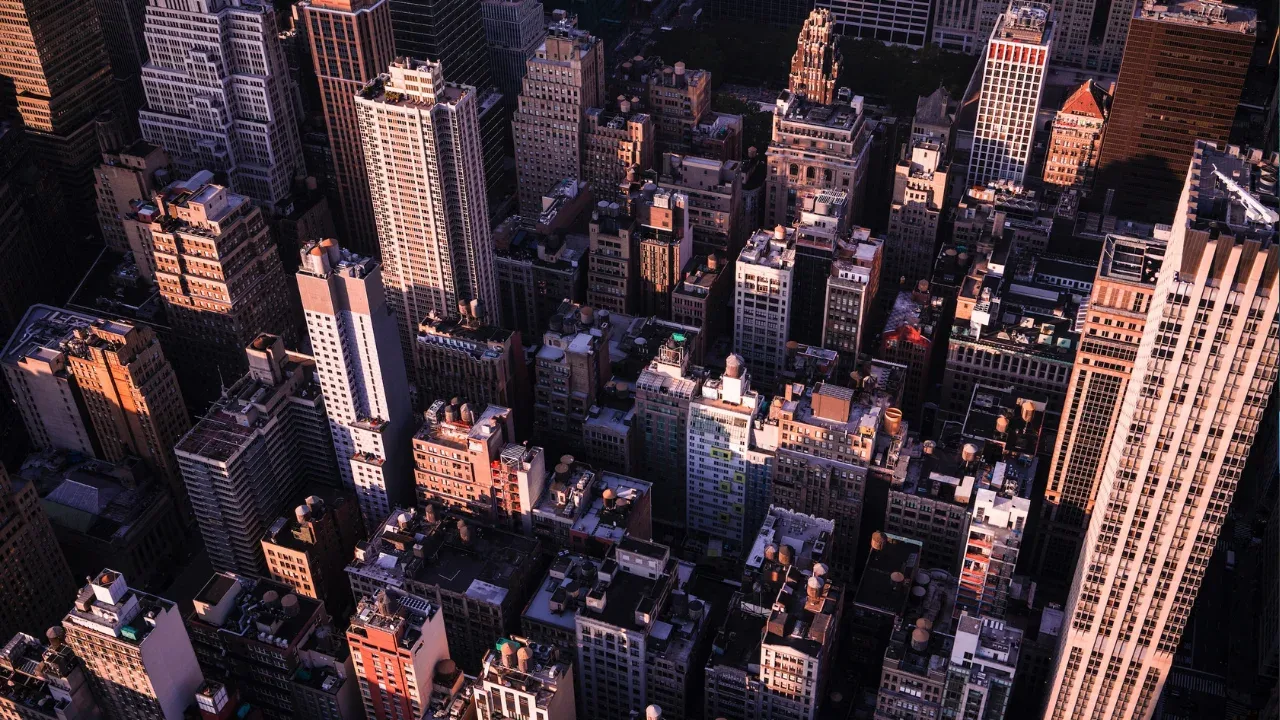
📝 Blog Post - Mastering the class << self
Idiom in Ruby 🚀
Do you sometimes stumble upon the class << self
idiom in Ruby and wonder what it actually does? 🤔 Fear not! In this blog post, we'll demystify this concept and provide you with easy-to-understand solutions to common issues you may encounter. Let's dive in! 💎
Understanding the class << self
Idiom
The class << self
idiom is a powerful construct in Ruby that allows you to define methods within a singleton class. But what is a singleton class? 🤷♀️
In Ruby, every object has its own singleton class, which is like a hidden class that only that object has access to. The class << self
syntax opens up the singleton class of the current object.
When used within a class definition, class << self
reopens the class and lets you define instance methods on the class itself. This means that the defined methods will be callable directly on the class, without needing an instance of the class. It's a way to add class-level behavior or modify existing class methods easily.
Common Issues and Easy Solutions
Issue 1: Accidentally defining instance methods instead of class methods
Let's say you want to define a class method called total_count
, which counts the total number of instances created. 📊 However, if you mistakenly define it as an instance method, you would encounter unexpected behavior.
class MyClass
def self.total_count
# implementation
end
end
Solution: Use the class << self
idiom to define the method within the singleton class. This ensures that the method is correctly defined as a class method.
class MyClass
class << self
def total_count
# implementation
end
end
end
Issue 2: Inheritance and overriding class methods
Suppose you have a base class Parent
with a class method called foo
. Now you create a subclass Child
, and you want to modify the behavior of the foo
method specifically for the Child
class. How can you achieve that? 🧐
class Parent
def self.foo
# base implementation
end
end
class Child < Parent
def self.foo
# overriding Parent#foo for Child
end
end
Solution: Use the class << self
idiom in the subclass to reopen the class and modify the method implementation.
class Parent
def self.foo
# base implementation
end
end
class Child < Parent
class << self
def foo
# overriding Parent#foo for Child
end
end
end
Ready to Supercharge Your Ruby Skills?
Mastering the class << self
idiom opens up new possibilities for your Ruby code. By understanding its usage and how to address common issues, you'll level up your skills in no time! 💪
Now that you've learned the ins and outs of this idiom, we encourage you to experiment with it in your own code. Feel free to share your findings or any questions you have in the comments below.
Happy coding! 😄👩💻👨💻
Note: If you found this blog post helpful, don't forget to share it with your fellow Rubyists. Let's spread the knowledge! 🌟
Take Your Tech Career to the Next Level
Our application tracking tool helps you manage your job search effectively. Stay organized, track your progress, and land your dream tech job faster.
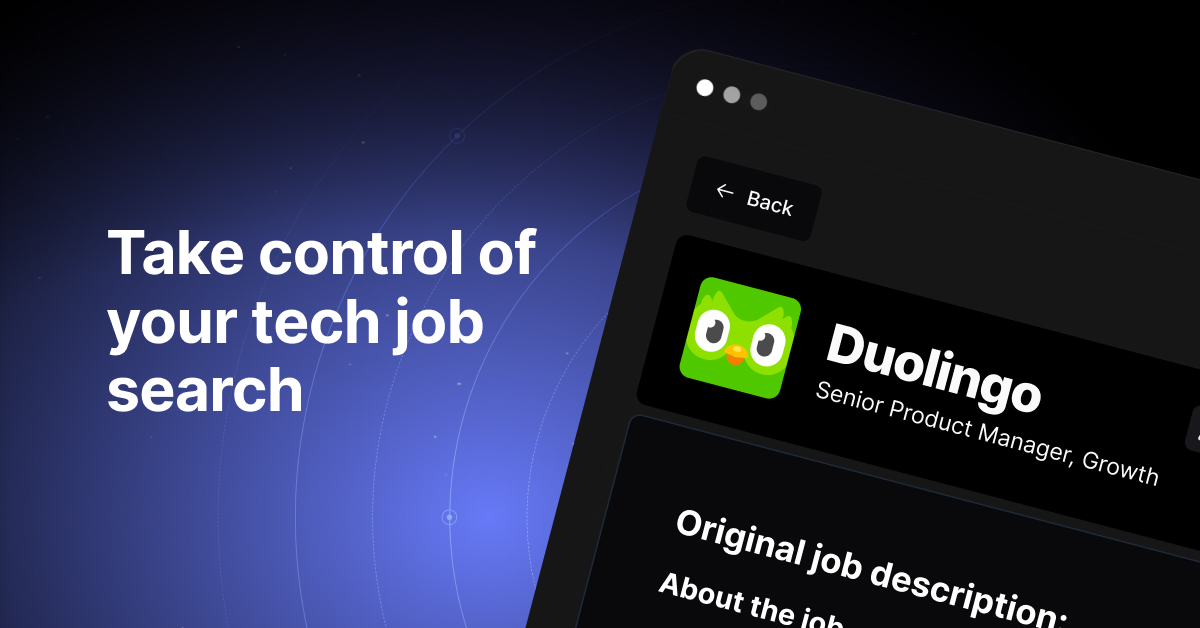