Best way to convert strings to symbols in hash
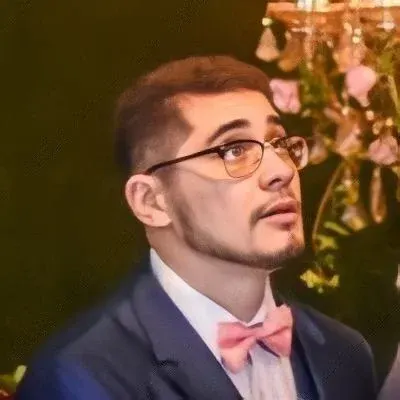
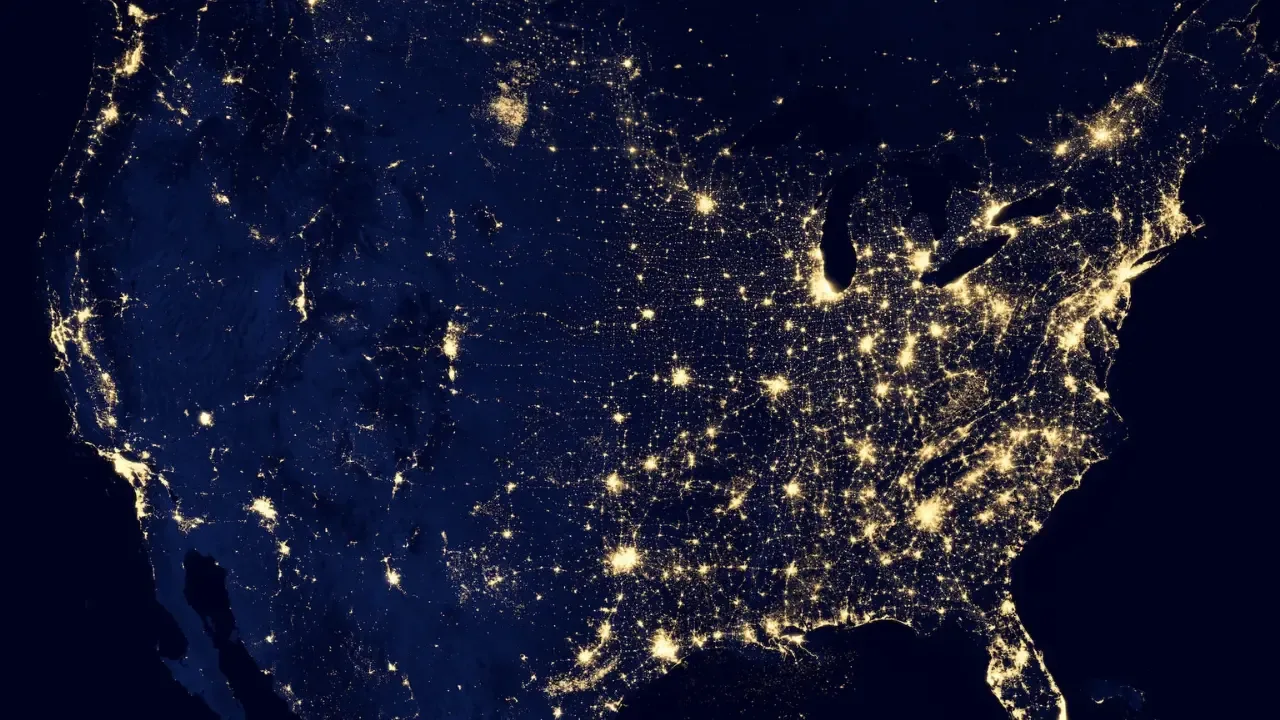
The Best Way to Convert Strings to Symbols in a Hash
Are you tired of dealing with string keys in your hashes? Do you find it cumbersome and error-prone to constantly access values with square brackets? Well, worry no more because in this blog post, I'll show you the best way to convert strings to symbols in a hash in Ruby!
The Problem
Let's imagine a scenario where you have a YAML file that you need to parse into a hash. By default, the keys in the resulting hash will be strings. However, if you prefer to work with symbols as keys, you'll run into some difficulties.
Using the example code snippet provided:
my_hash = YAML.load_file('yml')
You'd end up with a hash where the keys are strings. So, if you want to access a value, you'd have to use:
my_hash['key']
This can be tedious and less readable, especially when you're used to accessing hash values using symbols.
The Solution
Luckily, Ruby provides an easy solution to convert all the keys in a hash from strings to symbols by using the transform_keys
method. 🎉
my_hash = my_hash.transform_keys(&:to_sym)
By calling transform_keys
on the hash and passing &:to_sym
as an argument, each key in the hash will be converted to a symbol, allowing you to access the values using symbol notation, like so:
my_hash[:key]
Isn't that awesome? 😄
A Complete Example
To make things clearer, let's walk through a complete example. Consider the following YAML file (example.yml
):
name: John Doe
age: 30
city: New York
We want to parse this YAML file into a hash with symbol keys. Here's how you would do it:
require 'yaml'
# Load YAML file into a hash with string keys
my_hash = YAML.load_file('example.yml')
# Convert string keys to symbols
my_hash = my_hash.transform_keys(&:to_sym)
# Access values using symbol keys
puts my_hash[:name] # Output: John Doe
puts my_hash[:age] # Output: 30
puts my_hash[:city] # Output: New York
Now, we can access the values in the my_hash
hash using the much cleaner and easier-to-read symbol notation. 🚀
Your Turn to Convert!
Now that you know the best way to convert strings to symbols in a hash, it's time to give it a try in your own projects. Say goodbye to the headache of dealing with string keys and embrace the simplicity of symbol keys.
Have a similar problem or a different topic you'd like me to address? Let me know in the comments below! Let's dive into the world of Ruby and transform our coding experience together. 💪💻
Join the Conversation
I'd love to hear how this solution worked for you or if you have any other tips and tricks related to working with hashes in Ruby. Leave a comment below and share your thoughts and experiences.
Remember to share this blog post with your fellow Ruby developers who might find it helpful. Happy coding! 👩💻👨💻
*[to_sym]: This is a Ruby method that converts a string to a symbol
Take Your Tech Career to the Next Level
Our application tracking tool helps you manage your job search effectively. Stay organized, track your progress, and land your dream tech job faster.
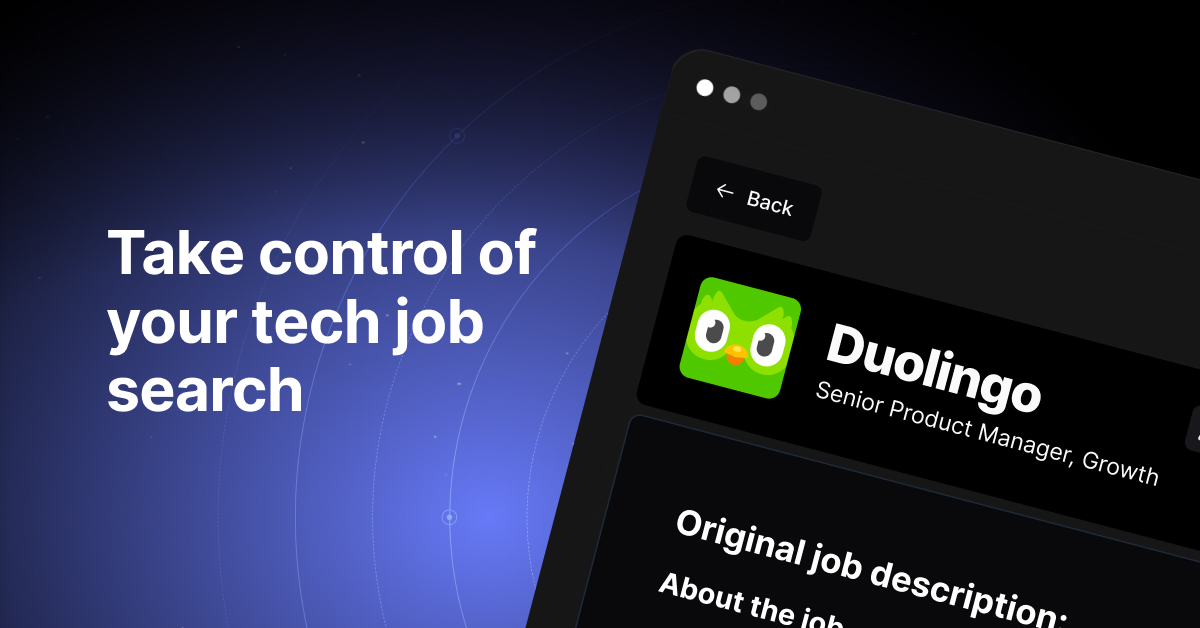