When to use React setState callback
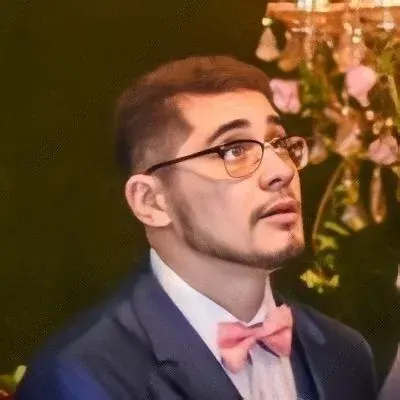
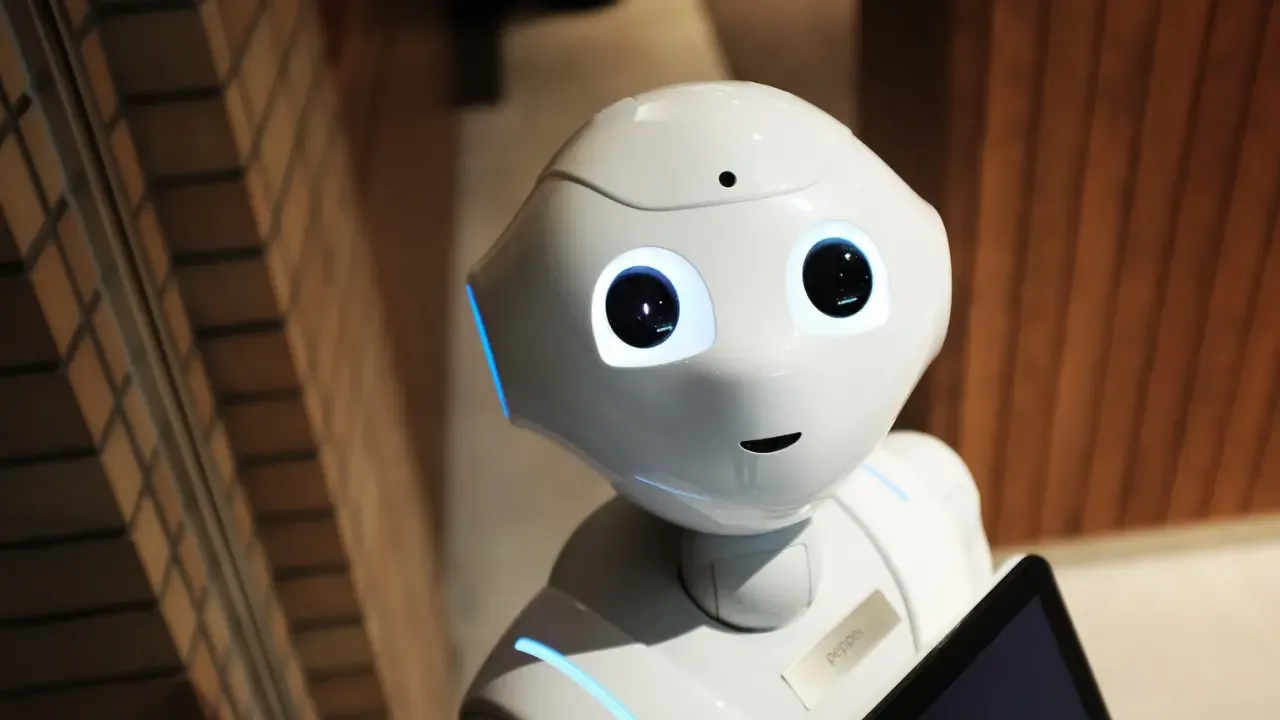
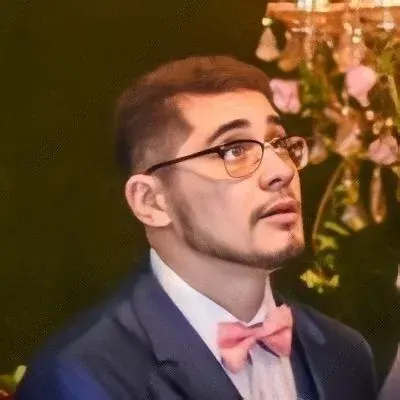
π When to use React setState callback: The Ultimate Guide
π Welcome to my tech blog! Today, we're going to dive into the world of React and discuss an important question: when should you use the setState
callback?
The Scenario
π§ Let's start by understanding the scenario that prompts this question. In React, whenever the state of a component changes, the render
method is automatically triggered. This means that you can perform actions directly within the render
method whenever a state change occurs.
π‘However, there is a specific use case for using the setState
callback.
The Use Case: π― The setState Callback
π€ Imagine this situation: you want to execute some code immediately after a state change, but you want to ensure that this code is executed after the state is actually updated. Here's where the setState
callback comes in handy.
π By passing a callback function as the second argument to the setState
method, you can guarantee that this function will be called once the state change is complete. This makes it the perfect solution for scenarios where you need to perform actions that rely on the updated state.
Common Issues Addressed
π Now, let's address some common issues you might encounter and see how the setState
callback can help resolve them.
1. Accessing the Updated State
π Consider a scenario where you want to access the updated state immediately after a setState
operation. Since setState
is asynchronous, simply calling it wouldn't give you the updated state right away. But fear not! By leveraging the setState
callback, you can access and work with the updated state as soon as it's available.
2. Ensuring Sequential Updates
π In some cases, you might need to perform multiple state updates in a sequence. However, due to the asynchronicity of setState
, the order of these updates may not be guaranteed. With the setState
callback, you can ensure that a series of state updates are performed in the desired order, preventing any unexpected behavior.
3. Triggering Side Effects
π Let's say you want to trigger a side effect, such as making an API call or updating a third-party library, immediately after a state change. By utilizing the setState
callback, you can easily handle such scenarios, ensuring that your actions are executed at the right moment.
Easy Solutions with Examples
π§ Now, let's get practical! Here are a few examples to demonstrate how to use the setState
callback for these common issues:
Accessing the Updated State:
this.setState({ count: this.state.count + 1 }, () => {
console.log("Updated count:", this.state.count);
});
Ensuring Sequential Updates:
this.setState((prevState) => ({ count: prevState.count + 1 }), () => {
console.log("Updated count:", this.state.count);
});
Triggering Side Effects:
this.setState({ isLoading: true }, () => {
// Make API call or update third-party library here
console.log("API call completed");
});
Remember, the callback function within setState
is executed after the state update is complete. You can perform any desired actions within this callback!
Call-to-Action: Engage and Share Your Experience
π£ I hope this guide helped you understand when and how to use the setState
callback in React. Now, it's your turn to share your experience!
π¬ Have you encountered any challenges or interesting use cases where the setState
callback proved to be valuable? Let's discuss in the comments section below! Your insights and experience might help fellow developers facing similar situations.
π If you found this guide helpful, don't forget to share it with your friends and colleagues! Let's spread the knowledge together.
Happy coding! ππ