What"s the difference between "super()" and "super(props)" in React when using es6 classes?
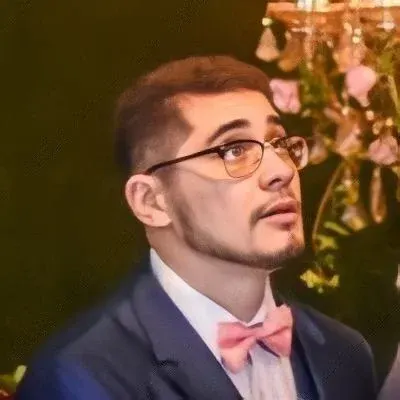
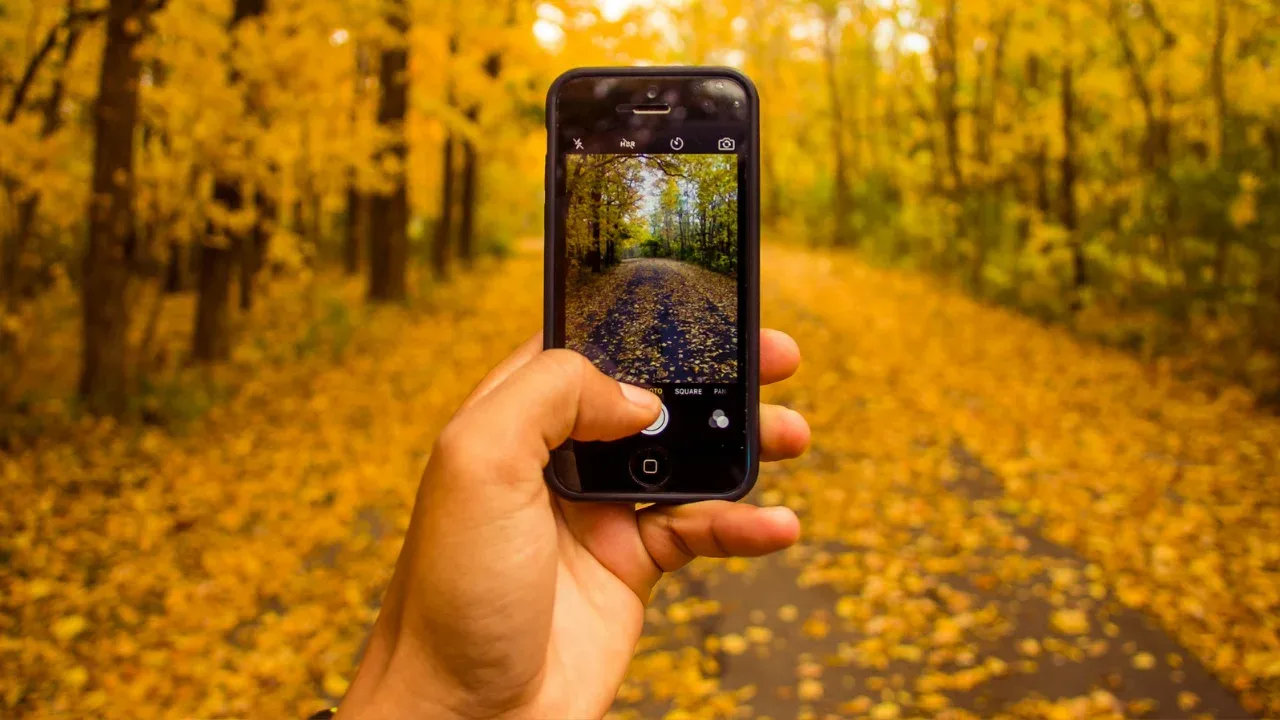
The Super Dilemma: super()
vs super(props)
in React ES6 Classes 😕🤔
So you're building a React app, and you stumbled upon a roadblock in your code. You have a component class with a constructor, and you're not sure whether to use super()
or super(props)
inside it. Don't fret! This blog post is here to save the day and clarify the differences between these two approaches. By the end, you'll have a crystal-clear understanding and be able to handle this dilemma like a pro. Let's dive in! 🏊♀️📚
The Basics: What is super()
? 🧐
In React, super()
is used to call the constructor of the parent class (the React.Component
class in this case) before initializing your own class. It ensures that all the important functionality of the parent class is inherited by the child class. If you forget to call super()
, you'll encounter an error due to an incomplete initialization. So, remember: always use super()
as the first line of code in your constructor!
When to Pass props
to super(props)
? 🎁
Now let's talk about the props
parameter. In React, props
is an object that contains all the properties passed to a component by its parent component. When you use super(props)
, you're not only calling the parent constructor but also passing the props
object to it. This allows the parent component to access and utilize the props
data as well.
So, when is it important to pass props
to super()
? The answer lies in whether you need to access the props
in your constructor or in the parent class. If your constructor requires access to the props
object, then using super(props)
is the way to go. However, if you don't need to access props
in the constructor, you can simply call super()
without passing props
as a parameter, and React will handle it implicitly. Easy peasy! 🤩
Example Time! 🎉👩💻
Let's take a look at a practical example to solidify our understanding. Consider the following code snippet:
class MyComponent extends React.Component {
constructor(props) {
super(props);
// additional component initialization code
}
}
In this example, we have a MyComponent
class inheriting from React.Component
. The constructor
takes in props
as a parameter and calls super(props)
. By doing so, the MyComponent
class ensures that both its own constructor and the parent class constructor are properly invoked. This allows us to access the props
object within the constructor and perform any additional initialization specific to MyComponent
.
The Simpler Approach: super()
Without props
🚀
But what if you don't really need to access the props
object in your constructor? In such cases, you can simplify your code by omitting the props
parameter when calling super()
. Here's an example:
class MyComponent extends React.Component {
constructor() {
super();
// additional component initialization code
}
}
By excluding props
from super()
, React will still take care of the inheritance behind the scenes, and you can focus on other aspects of your component without worrying about the props
object in the constructor.
The Final Verdict: super()
or super(props)
? 🤔✅
To sum it all up, you can use either super()
or super(props)
in your constructor, depending on whether you need to access the props
object within the constructor or the parent class.
Use
super()
if you don't need to accessprops
in the constructor.Use
super(props)
if you want to access theprops
object in the constructor or the parent class.
Remember to always call super()
as the first line of code in your constructor to ensure proper class initialization.
Your Turn to Decide! ✍️🗳️
Now that you understand the difference between super()
and super(props)
, it's time to choose the appropriate approach for your React component. Take a look at your code and consider whether you need to access the props
object in the constructor or parent class. Once you've made your decision, go ahead and implement it.
If you found this blog post helpful, feel free to share it with your fellow developers and spread the knowledge! Also, I'd love to hear your thoughts and any other React topics you'd like me to cover. Leave a comment below and let's keep the conversation going! Happy coding! 😄💻🚀
Take Your Tech Career to the Next Level
Our application tracking tool helps you manage your job search effectively. Stay organized, track your progress, and land your dream tech job faster.
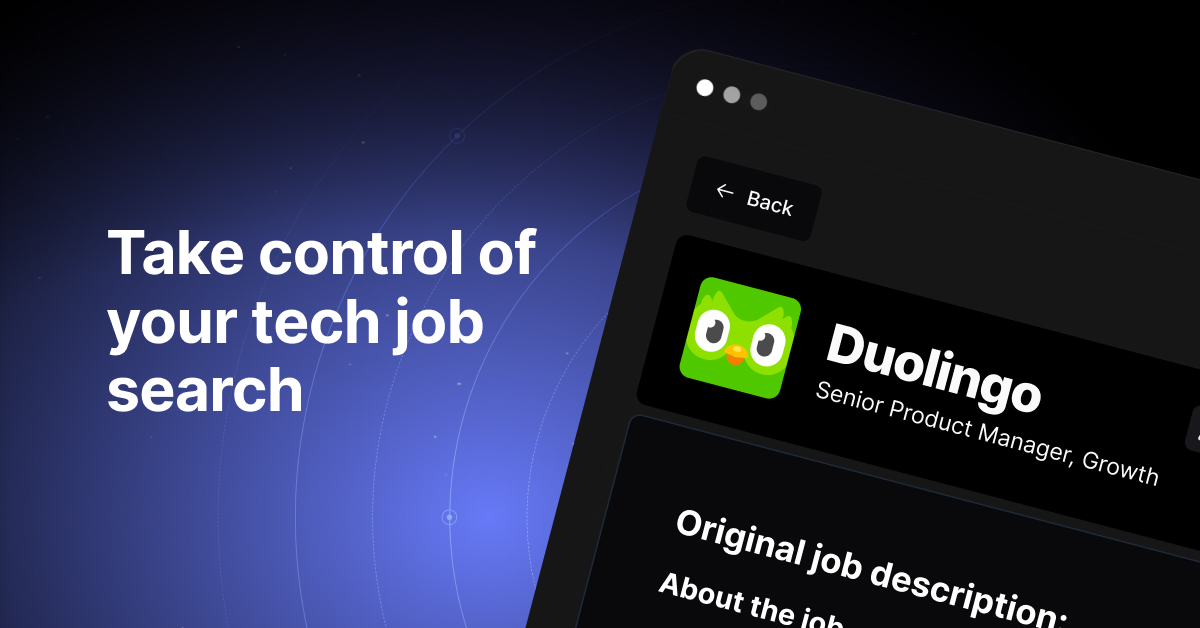