What is the best way to access redux store outside a react component?
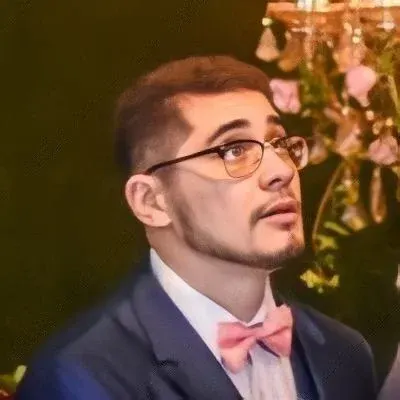
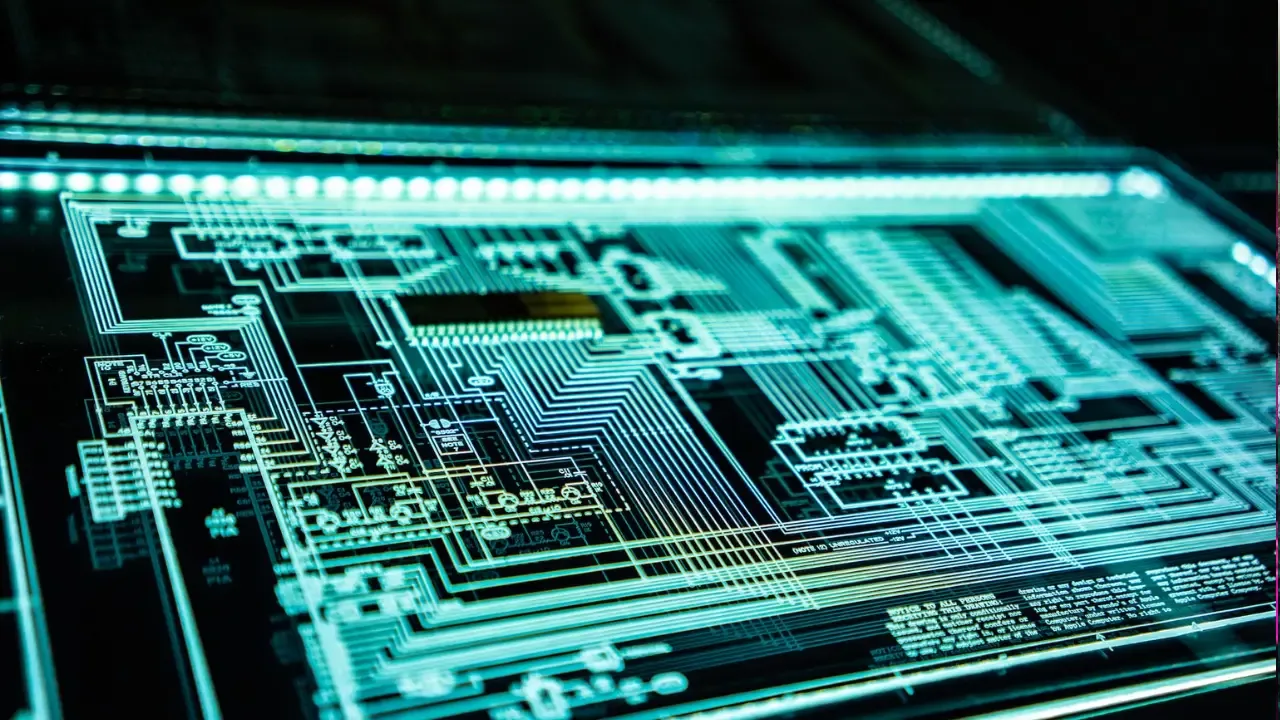
Easy Access to Redux Store Outside a React Component 🌟
So you've got the hang of using @connect
to access the Redux store within a React component, but now you find yourself in a situation where you need to access the store outside of a component. Specifically, you want to use an authorization token from the store in your api.js
file to create a global Axios instance for your app. Fear not -- we've got a simple and efficient solution for you! 😎
The Challenge 🤔
Let's quickly recap the problem: you want to access a data point from your Redux store (specifically, the authorization token) and use it in your api.js
file to set the Axios headers. However, traditional methods like @connect
won't work outside of a component. So, how can you achieve global access to the Redux store in a non-component file?
The Solution 💡
Introducing the redux
package, the powerhouse behind Redux which allows us to access the store from anywhere in our app. To utilize this package, we need to follow a few simple steps:
Install the
redux
package via your favorite package manager:npm install redux
Import the necessary
redux
functions in yourapi.js
file:import { createStore } from 'redux'
Initialize the Redux store with your reducers:
import rootReducer from './reducers' // assuming your reducers are in a separate file const store = createStore(rootReducer)
Export the Redux store:
export default store
Back in your
api.js
file, import the Redux store:import store from './store'
Now, you can access the store's data using
getState()
. Here's an example of how you can retrieve the authorization token:const authToken = store.getState().auth.tokens.authorization_token
Optimizing the Solution 🚀
While the above solution works perfectly fine, we can make it a bit more elegant by utilizing Redux's middleware functionality. By creating a custom middleware, we can set the Axios headers automatically whenever the authorization token changes. Here's how:
Install the
redux-thunk
package:npm install redux-thunk
Import the
applyMiddleware
function fromredux
and thethunk
middleware fromredux-thunk
:import { createStore, applyMiddleware } from 'redux' import thunk from 'redux-thunk'
Update the store initialization code to include the
thunk
middleware:const store = createStore(rootReducer, applyMiddleware(thunk))
Create a custom middleware function:
const updateAxiosHeaders = store => next => action => { if (action.type === 'UPDATE_AUTH_TOKEN') { const authToken = store.getState().auth.tokens.authorization_token // Set the Axios headers with the authorization token api.defaults.headers.common['Authorization'] = authToken } return next(action) }
Apply the custom middleware to your store:
const store = createStore(rootReducer, applyMiddleware(thunk, updateAxiosHeaders))
Now, whenever the
authToken
changes in the Redux store (via an action likeUPDATE_AUTH_TOKEN
), the custom middleware will automatically update the Axios headers.
Call-to-Action ✨
And that's it! You now have a streamlined solution for accessing the Redux store outside of a React component and using it to set Axios headers. 🎉
We hope this guide has been helpful to you! If you have any further questions or insights, please share them in the comments below. Let's make accessing Redux store outside of a component a breeze for everyone! 💪
Take Your Tech Career to the Next Level
Our application tracking tool helps you manage your job search effectively. Stay organized, track your progress, and land your dream tech job faster.
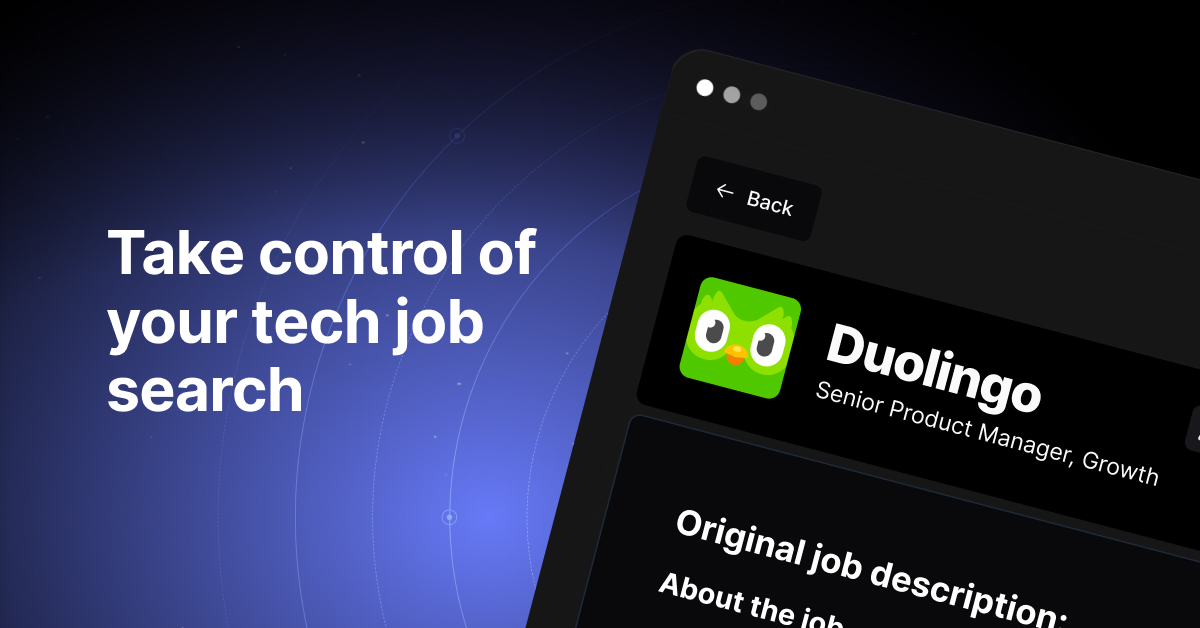