What is "not assignable to parameter of type never" error in TypeScript?
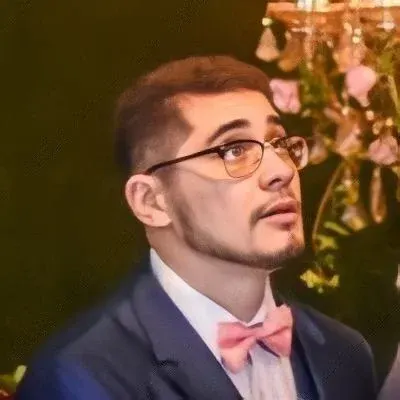

ππ₯βοΈHey there tech enthusiasts! Are you struggling with the dreaded "not assignable to parameter of type never" error in TypeScript? π€ Don't worry, you're not alone! In this blog post, we'll dive deep into this common issue and provide you with easy solutions to conquer it. Let's get started! πͺ
So, you stumbled upon this error while working on your TypeScript code π¨βπ». Here's the snippet that caused all the trouble:
const foo = (foo: string) => {
const result = []
result.push(foo)
}
Ouch! π± And here comes the error message:
[ts] Argument of type 'string' is not assignable to parameter of type 'never'.
Now, you're asking yourself, "What am I doing wrong? Is this a bug?" π Let's break it down and find out!
The error message is telling you that you're trying to assign a value of type 'string' to a parameter of type 'never'. In TypeScript, 'never' represents a type that can never occur. It's used to indicate that something should never happen or a variable should never have a value.
In our code snippet, the issue lies in the line const result = []
. TypeScript does its best to infer the type of 'result' from the assigned value, which is an empty array. Unfortunately, it concludes that the type of 'result' is 'never[]'. π΅
Now, when we try to push the 'foo' argument (which is of type 'string') into 'result', TypeScript throws the error. The reason being, the 'never' type is not compatible with any other types, hence the "not assignable" error. π
π§π‘So, how can we fix this? There are two simple solutions:
1οΈβ£ Specify the type of 'result' explicitly:
const result: string[] = []
result.push(foo)
By explicitly stating the type of 'result' as 'string[]', we ensure that TypeScript understands the expected type and the error magically disappears! β¨
2οΈβ£ Use type inference:
const result = [] as string[]
result.push(foo)
Using the 'as' keyword, we inform TypeScript that we expect 'result' to be of type 'string[]'. This also resolves the error and keeps our code clean and concise. π§Ήπ»
Now that we've conquered the "not assignable to parameter of type never" error, it's time to celebrate! ππ
But before you go, share your experiences with this error in the comments below! Have you encountered it before? Did our solutions work for you? Let's discuss and help each other out! π£οΈπ¬
Remember, don't let TypeScript errors get you down! With a little understanding and some handy tips, you'll be coding like a pro in no time. Happy coding! πβ¨
Take Your Tech Career to the Next Level
Our application tracking tool helps you manage your job search effectively. Stay organized, track your progress, and land your dream tech job faster.
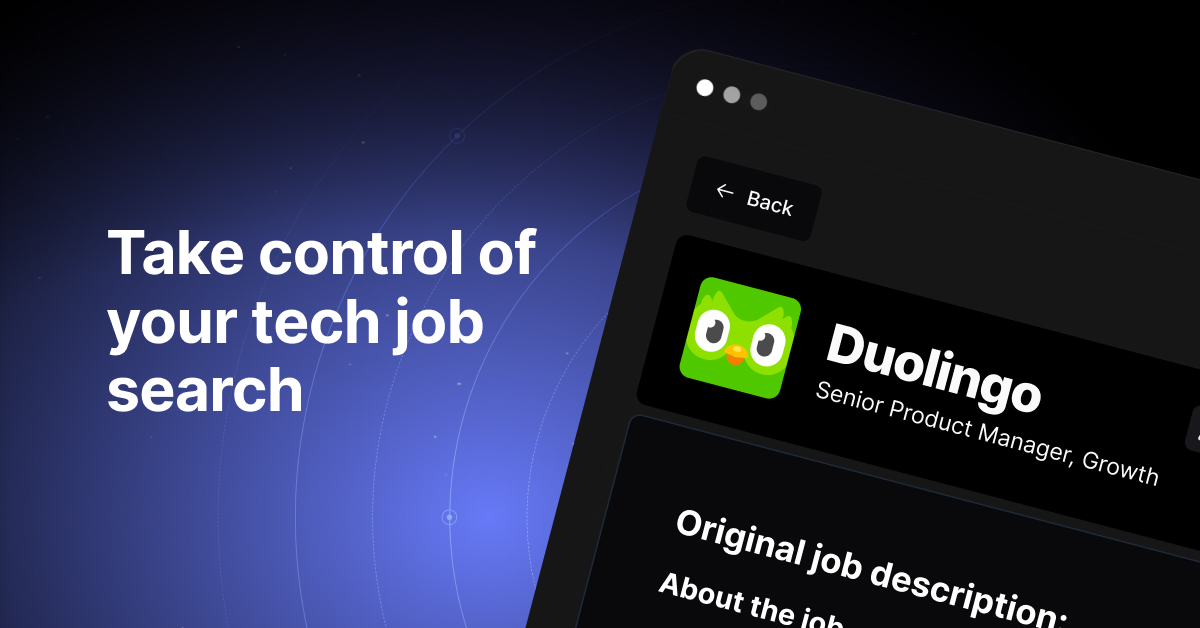