Updating an object with setState in React
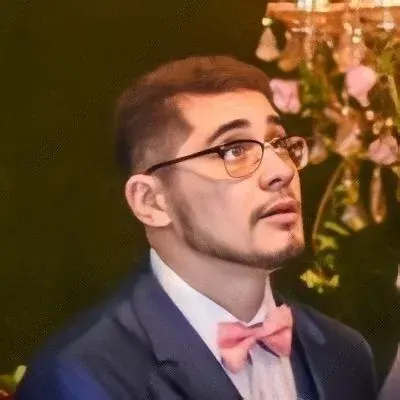
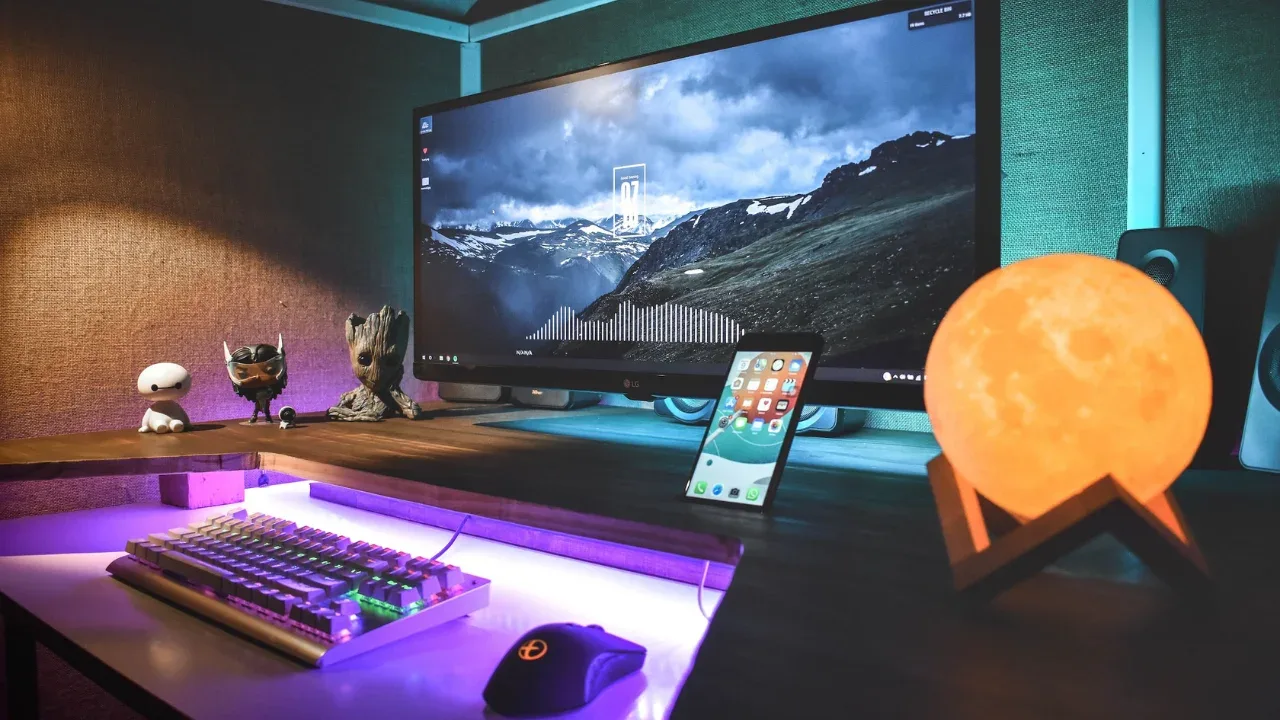
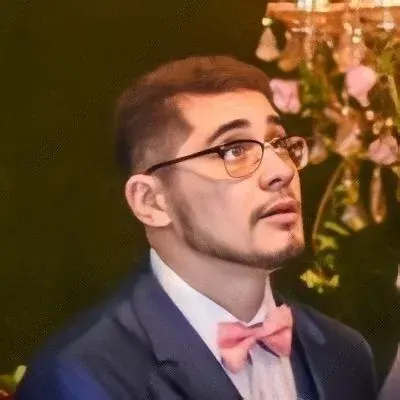
Updating an Object with setState in React: The Ultimate Guide! π¨βπ»π₯
Are you stuck trying to update an object's properties using setState
in React? Don't worry, you're not alone! π
Many React developers have faced this issue, but fear not - we're here to guide you through it with easy solutions and clear explanations. Get ready to level up your React game! πβ¨
The Problem:
So, you have a state object with a nested object inside it, just like this:
this.state = {
jasper: { name: 'Jasper', age: 28 },
}
You want to update the name
property of the nested jasper
object using setState
, but things don't seem to work when you try the following approaches:
this.setState({jasper.name: 'someOtherName'});
or
this.setState({jasper: { name: 'someothername' }})
The Explanation:
βοΈHere's the deal: When you call setState
, React merges your update into the current state, instead of replacing it entirely. This means that when you use setState
with an object, you need to provide the entire object that you want to update, not just the property you wish to change.
In the first approach, {jasper.name: 'someOtherName'}
, you're using object literal shorthand, which causes a syntax error since it's not valid syntax.
In the second approach, {jasper: { name: 'someothername' }}
, you are replacing the entire jasper
object, not just updating the name
property. As a result, the age
property of the jasper
object is lost and not preserved within the state update.
The Solution:
π Drumroll, please! You can solve this issue in a few different ways:
Option 1: Using the Spread Operator (Recommended) π
this.setState(prevState => ({
jasper: { ...prevState.jasper, name: 'someOtherName' }
}));
In this approach, we use the spread operator (...
) to create a shallow copy of the jasper
object. Then, we update the name
property, ensuring that the age
property remains unchanged.
Option 2: Using Object.assign
(Old-school, but still good) π©
this.setState(prevState => ({
jasper: Object.assign({}, prevState.jasper, { name: 'someOtherName' })
}));
Here, we use Object.assign
to create a new object by merging the properties of prevState.jasper
and { name: 'someOtherName' }
. Remember to pass an empty object {}
as the first argument to Object.assign
to initialize the new object.
Option 3: Using a deep clone (Not recommended for complex objects) π«
const updatedJasper = JSON.parse(JSON.stringify(this.state.jasper));
updatedJasper.name = 'someOtherName';
this.setState({ jasper: updatedJasper });
In this approach, we create a deep clone of the jasper
object using JSON.parse(JSON.stringify())
, update the name
property in the clone, and finally, update the state with the new jasper
object.
The Call-to-Action:
Congratulations, you've mastered the art of updating object properties with setState
in React! π If you found this guide helpful, share it with fellow React enthusiasts to spread the knowledge. And don't forget to subscribe to our newsletter for more exciting React tips and tricks! ππ‘
Feel free to leave a comment below if you have any questions or need further assistance with React or any other cool tech topic. Let's continue learning and coding together! ππ©βπ»π¨βπ»
Stay curious, stay excited, and happy coding! πͺβ¨