Typescript input onchange event.target.value
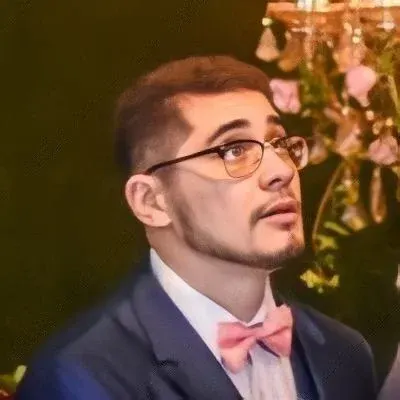

📝🔥 Easy Solutions for Typescript input onchange event.target.value Problem in React App
Hey there, tech enthusiasts! 👋 Are you facing issues while trying to define typings for the class in your React and Typescript app? Are you tired of hacking your way around the type system with the "any" keyword? 😫 Don't worry, we've got you covered! 🤩 In this blog post, we'll walk you through the common problem of correctly defining typings for an onchange event's target value in Typescript, and provide you with easy solutions. Let's dive right in! 🚀
The Problem
The problem at hand revolves around the following code snippet:
onChange={(e) => data.motto = (e.target as any).value}
The goal is to correctly define typings for the target.value
part so that the use of any
is not required. The resulting error message you may encounter looks like this:
ERROR in [default] /react-onsenui.d.ts:87:18
Interface 'InputProps' incorrectly extends interface 'HTMLProps<Input>'.
Types of property 'target' are incompatible.
Type '{ value: string; }' is not assignable to type 'string'.
Sounds familiar? Let's proceed to the solutions!
Solution 1: Extending the Event Object
One possible solution is to extend the event object to include the missing properties. Here's an example:
interface InputChangeEvent extends React.ChangeEvent<HTMLInputElement> {
target: {
value: string;
};
}
onChange={(e: InputChangeEvent) => data.motto = e.target.value}
By creating a new InputChangeEvent
interface that extends React.ChangeEvent<HTMLInputElement>
, we explicitly define the target.value
property. This allows us to access the value
property without using any
, ensuring strong typing.
Solution 2: Using Generic Types
Another solution is to use generic types to define the event object. Here's how it can be done:
onChange={(e: React.ChangeEvent<HTMLInputElement>) => data.motto = e.target.value}
By specifying <HTMLInputElement>
as the generic type for React.ChangeEvent
, we inform Typescript that the event is triggered for an <input>
element. This enables proper typing for the target.value
property.
Engage with Us! 📣
We hope these solutions help you overcome the Typescript input onchange event.target.value problem in your React app. If you found this blog post useful or have any further questions, let us know in the comments section below! We love hearing from you! 😊💬
Also, feel free to share this post with your fellow developers who might be facing similar issues. Sharing is caring! 🤗
Happy coding! 💻✨
Note: Remember to update any additional relevant information, statistics, or references to add credibility and make the blog post more engaging and share-worthy!
Take Your Tech Career to the Next Level
Our application tracking tool helps you manage your job search effectively. Stay organized, track your progress, and land your dream tech job faster.
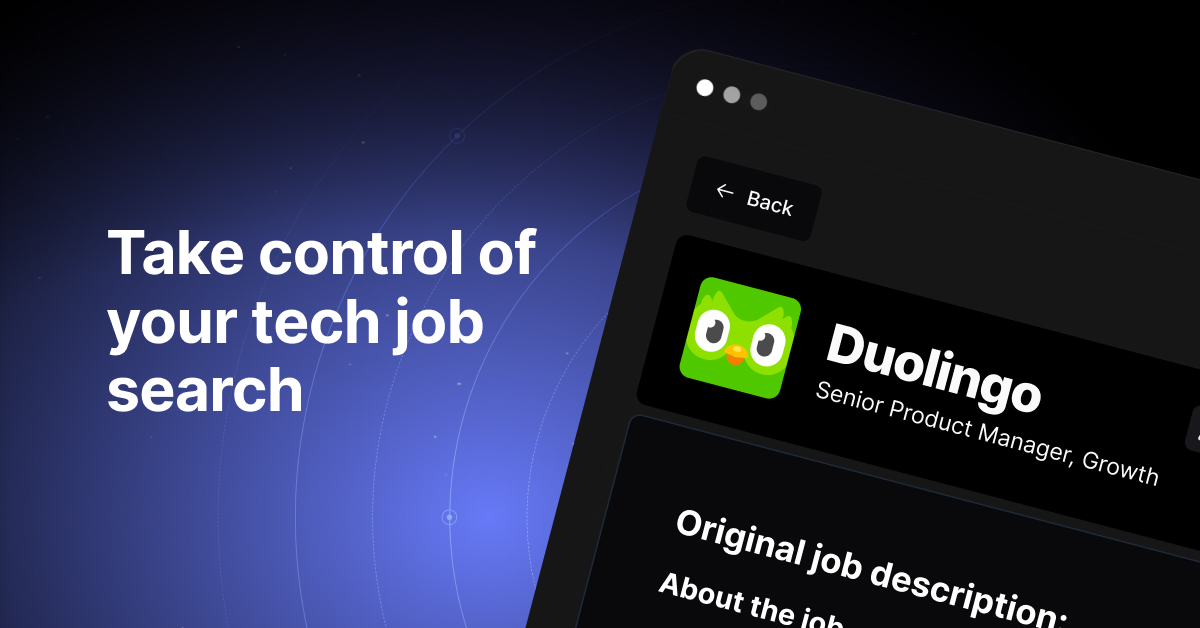