Sending the bearer token with axios
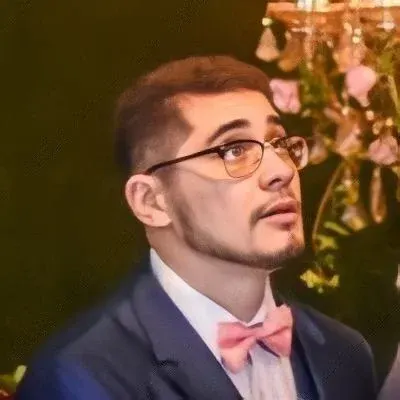
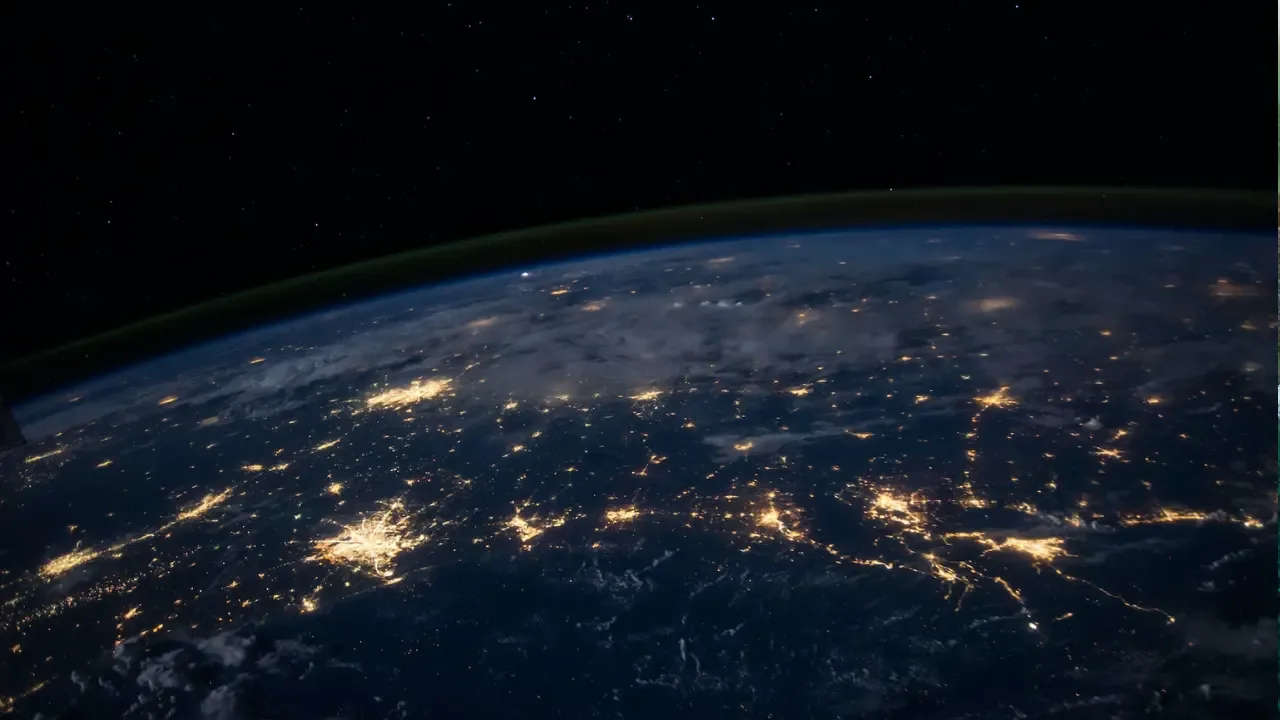
Sending the Bearer Token with Axios: A Complete Guide 🚀
Are you having trouble sending the Authorization
header with your requests using Axios in your React app? You've come to the right place! In this guide, we'll address the common issue of the Authorization
header not being sent and provide you with easy solutions. Let's dive in! 💪
The Problem: Unable to Send the Authorization Header 🚫
You're using Axios, a popular library for making HTTP requests in JavaScript, but you're facing an issue where the Authorization
header is not being sent along with your requests. This can be frustrating, especially if you're working on an app that requires authentication.
Here's an example of the code you're using:
tokenPayload() {
let config = {
headers: {
'Authorization': 'Bearer ' + validToken()
}
}
Axios.post(
'http://localhost:8000/api/v1/get_token_payloads',
config
)
.then( ( response ) => {
console.log( response )
} )
.catch()
}
You're setting the Authorization
header by concatenating the token obtained from your browser storage using the validToken()
method. However, the requests are consistently resulting in a 500 error response, with the message "The token could not be parsed from the request" from the backend.
The Solution: Axios Interceptors and Token Parsing ✅
To solve this issue, we need to modify our code and make use of Axios interceptors. Interceptors allow us to inspect and modify HTTP requests and responses. In this case, we'll use an interceptor to ensure that the Authorization
header is included with every request sent using Axios.
Here's an updated version of the code that includes the required interceptor:
import axios from 'axios';
// Create an instance of Axios
const api = axios.create({
baseURL: 'http://localhost:8000/api/v1',
});
// Add an interceptor to include the Authorization header
api.interceptors.request.use((config) => {
const token = validToken(); // Obtain the token from browser storage using the validToken() method
config.headers.Authorization = `Bearer ${token}`;
return config;
});
// Perform the request using the modified Axios instance
api.post('/get_token_payloads')
.then((response) => {
console.log(response);
})
.catch((error) => {
console.error(error);
});
In this updated code, we create an instance of Axios called api
and add an interceptor to it using api.interceptors.request.use()
. Inside the interceptor function, we obtain the token using the validToken()
method, and then set the Authorization
header with the necessary Bearer
prefix.
Now, when you make a request using the api
instance, the Authorization
header will automatically be included, ensuring that the token is sent with each request.
A Note on Other Modules with React 📚
While Axios is a popular choice for making HTTP requests in React, there are other modules available that you might consider for your project. Some popular alternatives include fetch
and superagent
. Each module has its pros and cons, so it's a good idea to explore their documentation and decide which one best fits your needs.
Engage with the Community! 💬
We hope this guide has helped you resolve the issue of sending the Authorization
header with Axios in your React app. If you have any further questions or face any difficulties, feel free to leave a comment below or reach out to the community for support. Let's learn and grow together! 🌱
Remember to share this guide with others who might find it useful, and stay tuned for more informative tech content on our blog. Happy coding! 😄👩💻👨💻
📣 Call-to-Action: Join the conversation!
Tell us about your experience with Axios and how you solved the issue of sending the Authorization
header. Leave a comment below and share your insights with the community. Let's learn from each other! 🌟
Take Your Tech Career to the Next Level
Our application tracking tool helps you manage your job search effectively. Stay organized, track your progress, and land your dream tech job faster.
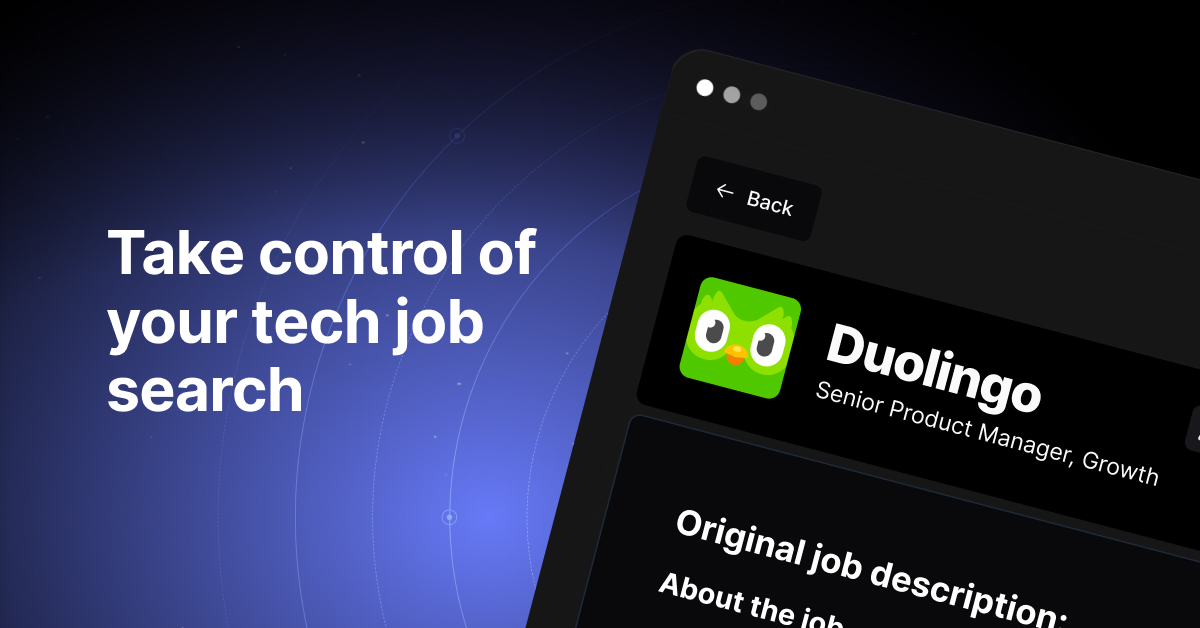