ReactJS: Warning: setState(...): Cannot update during an existing state transition
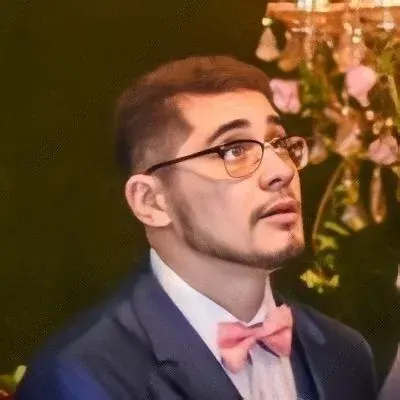
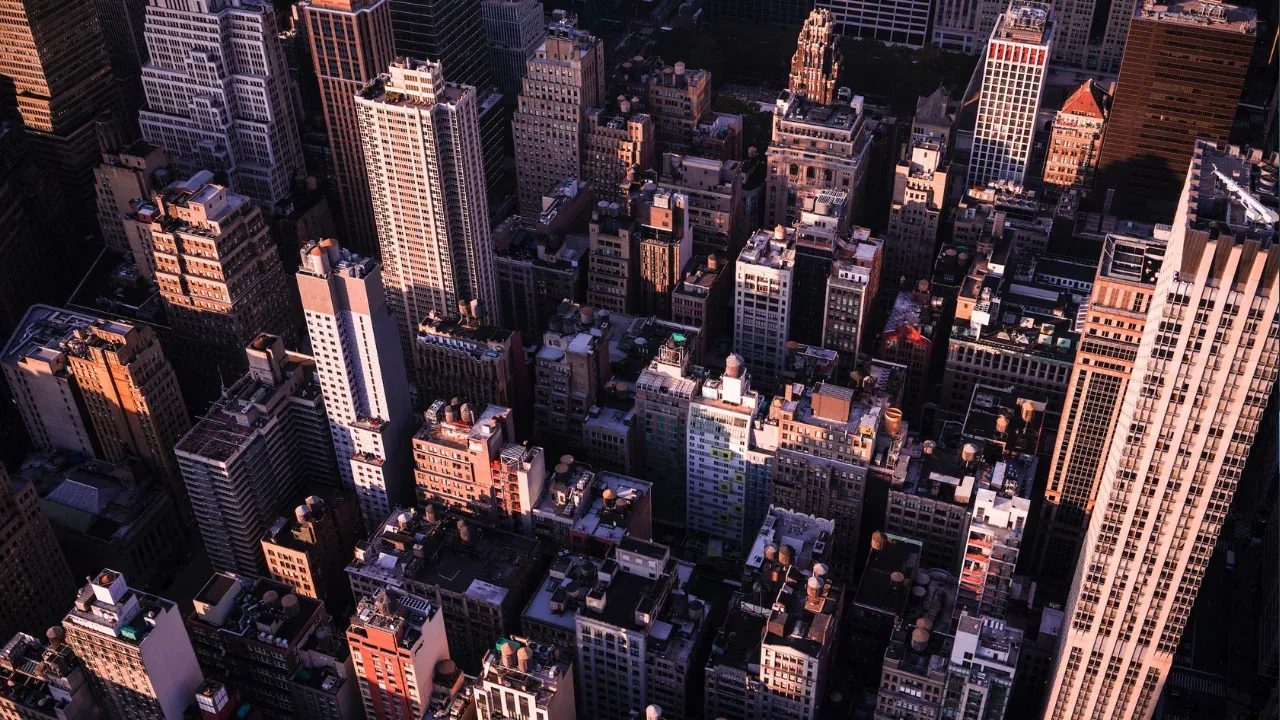
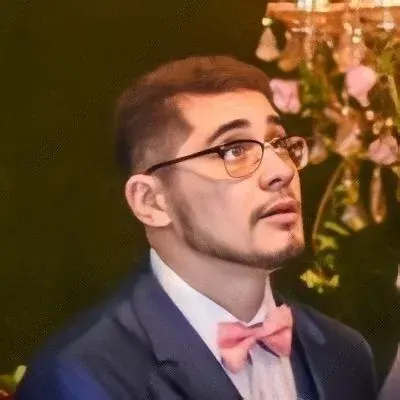
ReactJS: Warning: setState(...): Cannot update during an existing state transition 😱
Are you getting this error while working with ReactJS, and it's driving you crazy? Don't worry, I've got you covered! In this blog post, I'll explain why this error occurs, provide easy solutions to fix it, and ensure your app runs smoothly on all devices - including low-end mobile phones. 💻📱
The Problem 😫
One of our fellow developers was trying to refactor some code in their render view. They wanted to move the bind function from the render view to the constructor to improve performance on low-end devices. Here's the code snippet:
<Button href="#" active={!this.state.singleJourney} onClick={this.handleButtonChange.bind(this,false)}>Retour</Button>
But they kept encountering a frustrating error message that looked like this:
Warning: setState(...): Cannot update during an existing state transition (such as within `render` or another component's constructor). Render methods should be a pure function of props and state; constructor side-effects are an anti-pattern, but can be moved to `componentWillMount`.
The Explanation 🤔
Let's break it down. The error message is telling us that we cannot update the state during an existing state transition, such as within the render method or another component's constructor. ReactJS expects render methods to be pure functions of props and state and advises against performing constructor side-effects.
In our code snippet, the error occurs because we're updating the state in the onClick
event handler of the button. This event handler triggers a state change, but it's happening during the render phase, which is not allowed.
The Solution ✅
Luckily, there's an easy solution to this problem. Instead of updating the state directly in the render
method, we should move it to the componentWillMount
lifecycle method. Let's see how we can modify the code to fix the error:
export default class Search extends React.Component {
constructor() {
super();
this.state = {
singleJourney: false
};
this.handleButtonChange = this.handleButtonChange.bind(this);
}
componentWillMount() {
// Move the state update here
this.setState({
singleJourney: false
});
}
handleButtonChange(value) {
this.setState({
singleJourney: value
});
}
render() {
// Rest of the code
}
}
By moving the state update to the componentWillMount
method, we ensure that it happens before the render phase, allowing us to escape the state transition issue.
The Call-to-Action 🚀
Now that you've learned how to fix the "Cannot update during an existing state transition" error in ReactJS, it's time to put your knowledge into action. Try implementing the suggested solution in your own code and see if it resolves the issue.
If you have any questions or need further assistance, feel free to leave a comment below. I'm here to help you out! Happy coding! 💪⌨️
Don't forget to share this blog post with your fellow ReactJS developers who might be struggling with the same issue. Sharing is caring! ❤️🔗