React Router v4 - How to get current route?
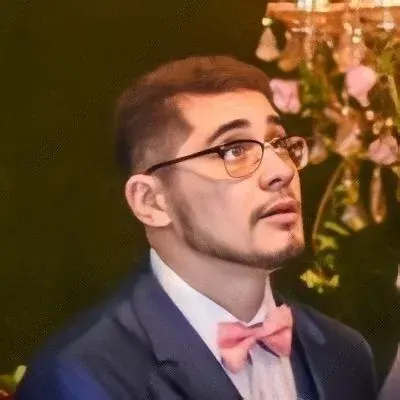
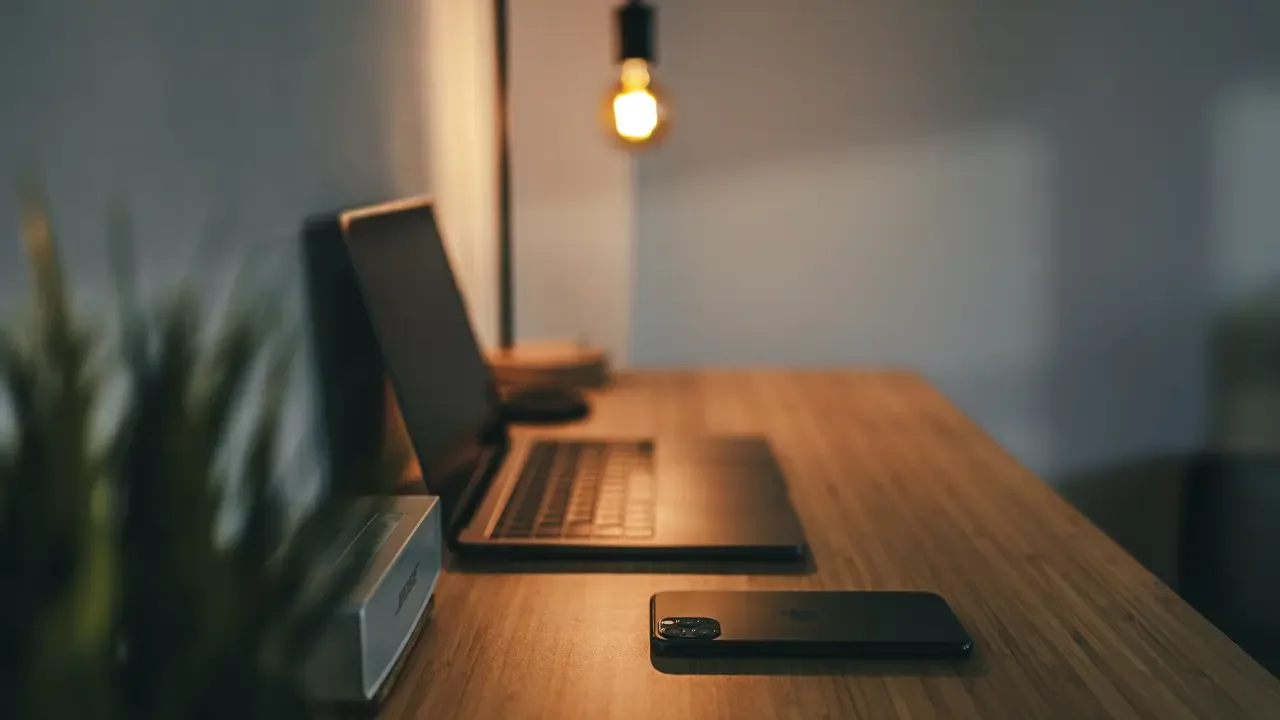
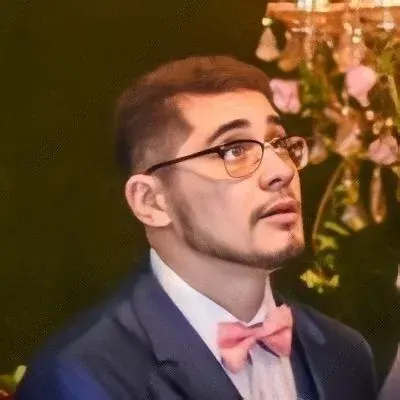
🚀 React Router v4 - How to get current route?
Are you struggling to pass the current route as a prop to the <AppBar />
component in React Router v4? Don't worry, I've got you covered! In this guide, I'll show you how to easily retrieve the current route and pass it to the desired component. Let's dive in! 😎
🧐 Understanding the Problem
To provide a dynamic title for the <AppBar />
component based on the current route, we need a way to access the current route within the component. In React Router v4, the route information is not directly available as a prop. So, how can we solve this problem? 🤔
💡 The Solution - withRouter
The solution lies in using the withRouter
higher-order component provided by the React Router library. By wrapping our component with withRouter
, we can access the current route information through the location
prop. Let's see how it works!
First, import the withRouter
function from React Router:
import { withRouter } from 'react-router-dom';
Next, wrap your component with the withRouter
higher-order component:
const AppBarWithRouter = withRouter(AppBar);
Now, the AppBarWithRouter
component has access to the location
prop, which contains the current route information. You can now use this prop to dynamically modify the title.
🚀 Implementing the Solution
Let's update the code snippet you provided to demonstrate how to pass the current route as the title
prop to the <AppBar />
component:
import React from 'react';
import { BrowserRouter as Router, Route, withRouter } from 'react-router-dom';
const AppBar = ({ title, location }) => {
// Modify the title based on the current route
const currentRoute = location.pathname;
const modifiedTitle = `Current Route: ${currentRoute}`;
return (
<div>
<h1>{modifiedTitle}</h1>
</div>
);
};
const AppBarWithRouter = withRouter(AppBar);
const App = () => {
return (
<Router basename="/app">
<main>
<AppBarWithRouter />
<Route path="/customers" component={Customers} />
</main>
</Router>
);
};
In the updated code snippet, we've wrapped the AppBar
component with withRouter
to gain access to the location
prop. We then modified the title
prop by appending the current route to it. Finally, we rendered <AppBarWithRouter />
in the <App />
component.
🚀 Try It Out!
Save the modifications, run your app, and navigate to different routes. You should now see the current route being displayed as the title in the <AppBar />
component. How cool is that? 😄
📣 Call-to-Action
React Router v4 provides great functionality to handle routes in your React application. By using the withRouter
higher-order component, you can easily access the current route and pass it to any desired component. Give it a try and unleash the power of dynamic routes in your app!
If you found this guide helpful, feel free to share it with fellow developers and leave a comment below. Also, don't hesitate to reach out if you have any questions or other topics you'd like me to cover. Happy coding! 🚀🎉