React PropTypes: Allow different types of PropTypes for one prop
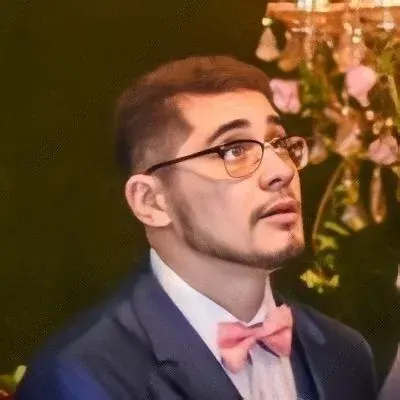
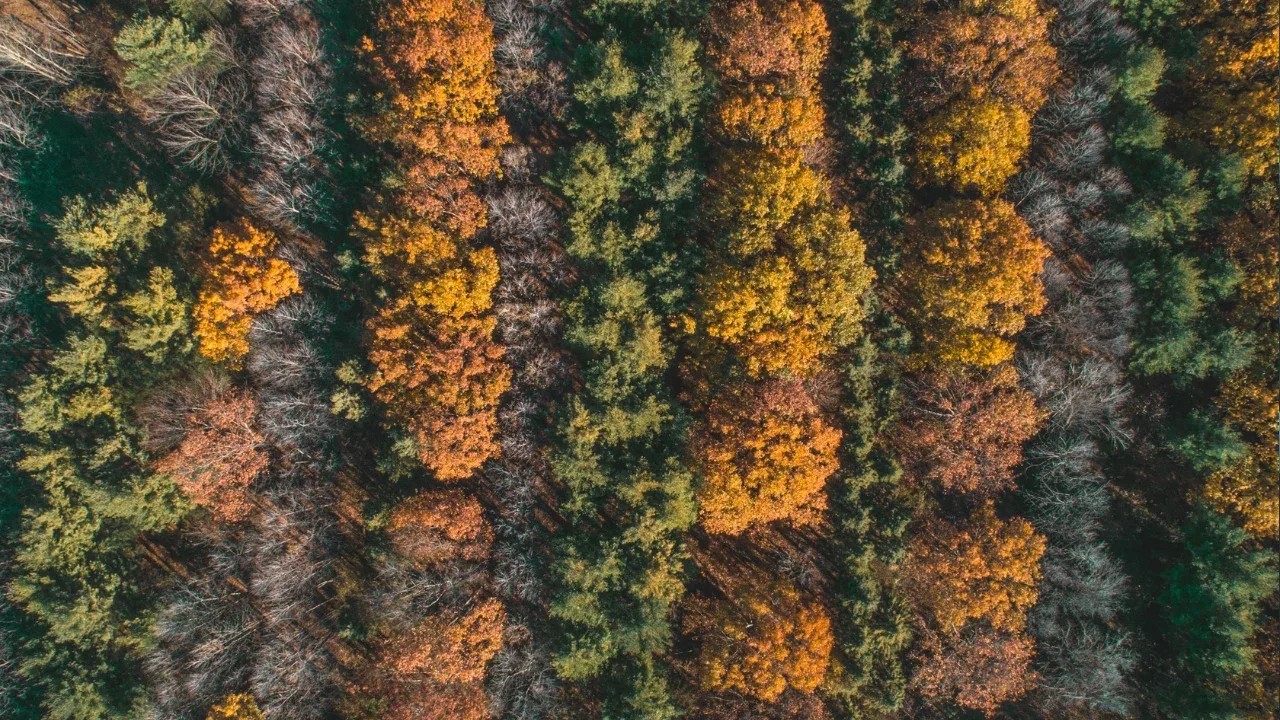
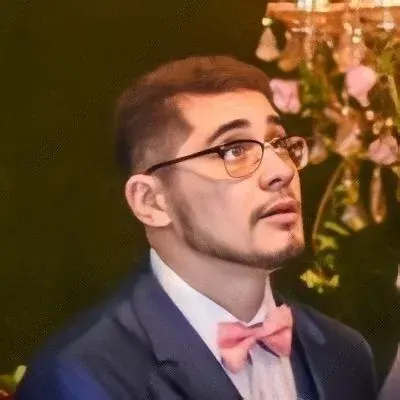
React PropTypes: Allow Different Types of PropTypes for One Prop 😎🔤💯
So, you have a React component that receives a prop called size
. And you're wondering, can you let React.PropTypes
know that this prop can be either a string or a number? 🤔
Well, the answer is yes! You can definitely achieve this using React PropTypes with a small tweak to your propTypes validation. Let me show you how! 🚀
The Warning Message 👀🚨
When you don't specify the type in your propTypes validation, you encountered a warning message:
prop type
size
is invalid; it must be a function, usually from React.PropTypes.
This warning occurs because React.PropTypes
expects a function to be set as the prop type, not the React.PropTypes
object itself.
Solution: One Func to Rule Them All 🛠🔢✨
To address this issue and allow different types for the size
prop, you'll use the function provided by React.PropTypes
to define the prop type.
MyComponent.propTypes = {
size: React.PropTypes.oneOfType([
React.PropTypes.string,
React.PropTypes.number
])
}
By using React.PropTypes.oneOfType
, you can create an array of valid types for the size
prop. In this case, the size
prop can be either a string or a number. 🌟
Complete Example 🌈🎉
To put it all together, here's a complete example of how your propTypes validation should look like:
import React from 'react';
import PropTypes from 'prop-types';
class MyComponent extends React.Component {
// Component code here
}
MyComponent.propTypes = {
size: PropTypes.oneOfType([
PropTypes.string,
PropTypes.number
])
}
export default MyComponent;
Time to Engage! 📢🙌
Now that you know how to allow different types for a single prop using React PropTypes, it's time to put it into practice! Update your code, test it out, and see the magic happen! ✨🔮
If you found this blog post helpful, don't hesitate to share it with your fellow React enthusiasts! And if you have any further questions or tips to share, feel free to leave a comment below. Let's learn and grow together! 🌱🚀
Happy coding! 💻😄