React prop validation for date objects
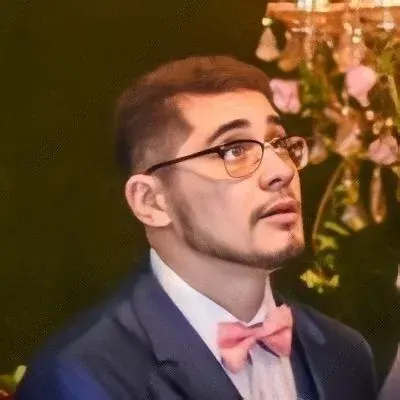
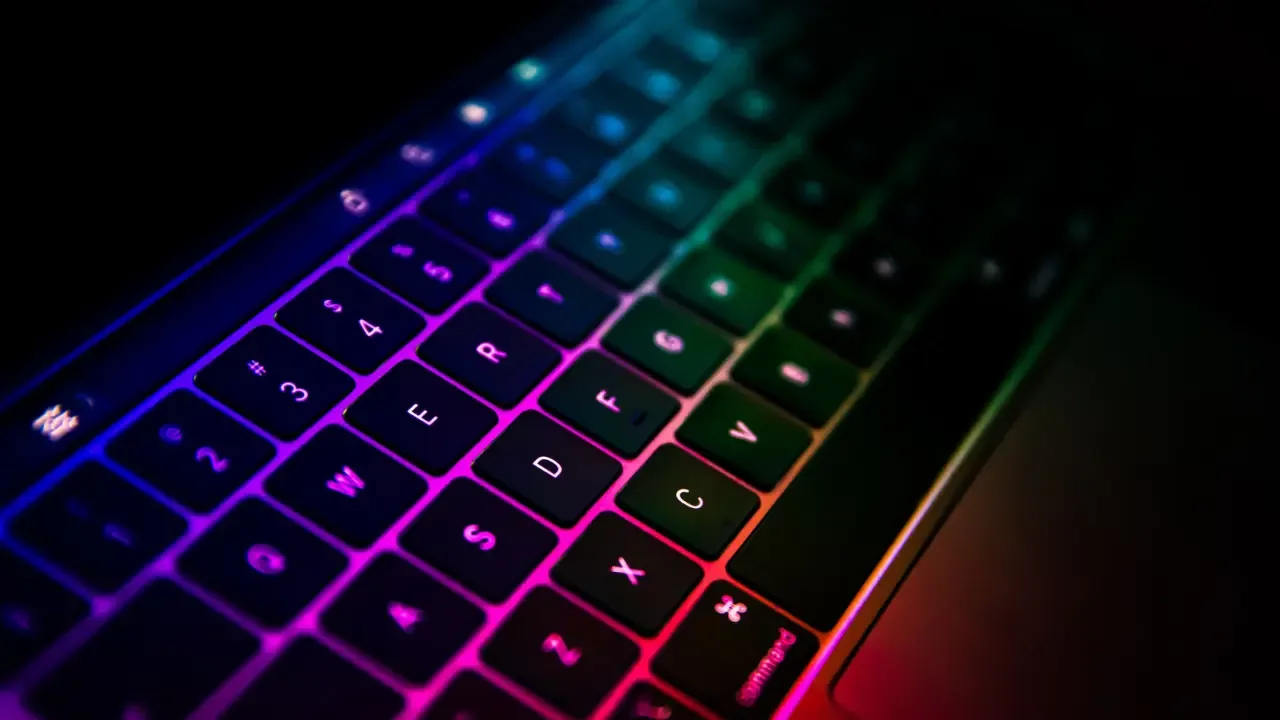
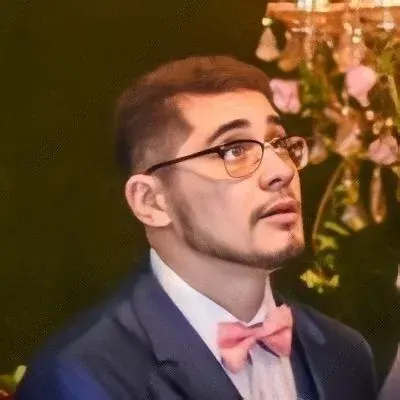
📝📅 React Prop Validation for Date Objects: A Complete Guide 📅📝
Are you struggling with validating Date prop objects in React? You're not alone! Many developers face this common issue and are unsure of the best way to handle it. But worry not, we've got you covered! In this blog post, we'll discuss the currently preferred way to validate a Date prop in React, address common issues, and provide easy yet effective solutions to ensure your code is clean and lint error-free. Let's dive in!
🎯 The Problem: PropTypes.object and the forbid-prop-types Rule
You mentioned using React.PropTypes.object to validate your Date prop. While this is a common approach, it fails the forbid-prop-types lint rule, causing frustration and confusion. So, how can we address this issue and find a better way to validate our Date props?
💡 The Solution: PropTypes.shape or a Custom Validator
There are two main solutions to consider: using PropTypes.shape or creating a custom validator.
1️⃣ PropTypes.shape:
PropTypes.shape allows you to define an object shape for your prop, validating its properties. Here's an example of how you can use PropTypes.shape to validate a Date prop:
import PropTypes from 'prop-types';
...
MyComponent.propTypes = {
myDateProp: PropTypes.shape({
getTime: PropTypes.func.isRequired, // Validate the getTime function
toISOString: PropTypes.func.isRequired, // Validate the toISOString function
}).isRequired,
};
By defining the required functions of the Date object, we ensure that the prop is indeed a Date object. However, note that this approach doesn't validate the entire Date object, only specific functions.
2️⃣ Custom Validator:
Another solution is to create a custom validator that performs a more thorough validation. Let's take a look at an example:
import PropTypes from 'prop-types';
...
function isDateProp(propValue, propName, componentName) {
if (!(propValue instanceof Date)) {
return new Error(
`Invalid prop ${propName} supplied to ${componentName}. Validation failed. Expected a Date object.`
);
}
}
MyComponent.propTypes = {
myDateProp: isDateProp.isRequired,
};
By using a custom validator, we can directly check if the prop value is an instance of the Date object. If it's not, an error is thrown.
Both options are valid approaches, so choose the one that suits your specific needs and coding style.
💪 Take Action: Level Up Your React Prop Validation Game 💪
Now that you have two reliable solutions to validate Date props in React, it's time to put them into practice! Improve your code quality and make your linter happy by adopting these techniques.
🌟 Challenge: Share Your Experience and Learn from Others 🌟
Have you faced any challenges with React prop validation for Date objects? Or do you have different approaches to share? We would love to hear your thoughts and insights! Engage with our community by commenting below and let's learn from each other.
🚀 Keep Learning, Keep Growing! 🚀
Mastering React prop validation is just one step on your journey as a React developer. Stay curious, keep learning, and explore more about React and related topics to become an even better developer. Remember, the sky is the limit!
We hope this guide has provided you with clarity and actionable solutions to your React prop validation woes. Don't let those pesky lint errors get you down. Happy coding!
📚🔗 Additional Resources:
👋 Until Next Time! 👋