"React" must be in scope when using JSX react/react-in-jsx-scope?
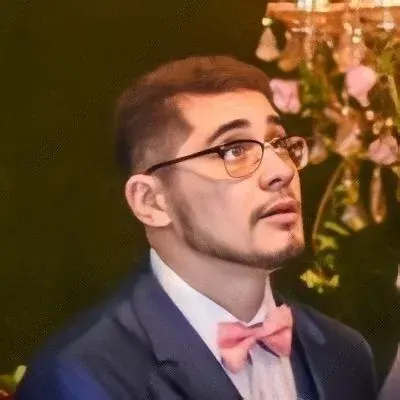
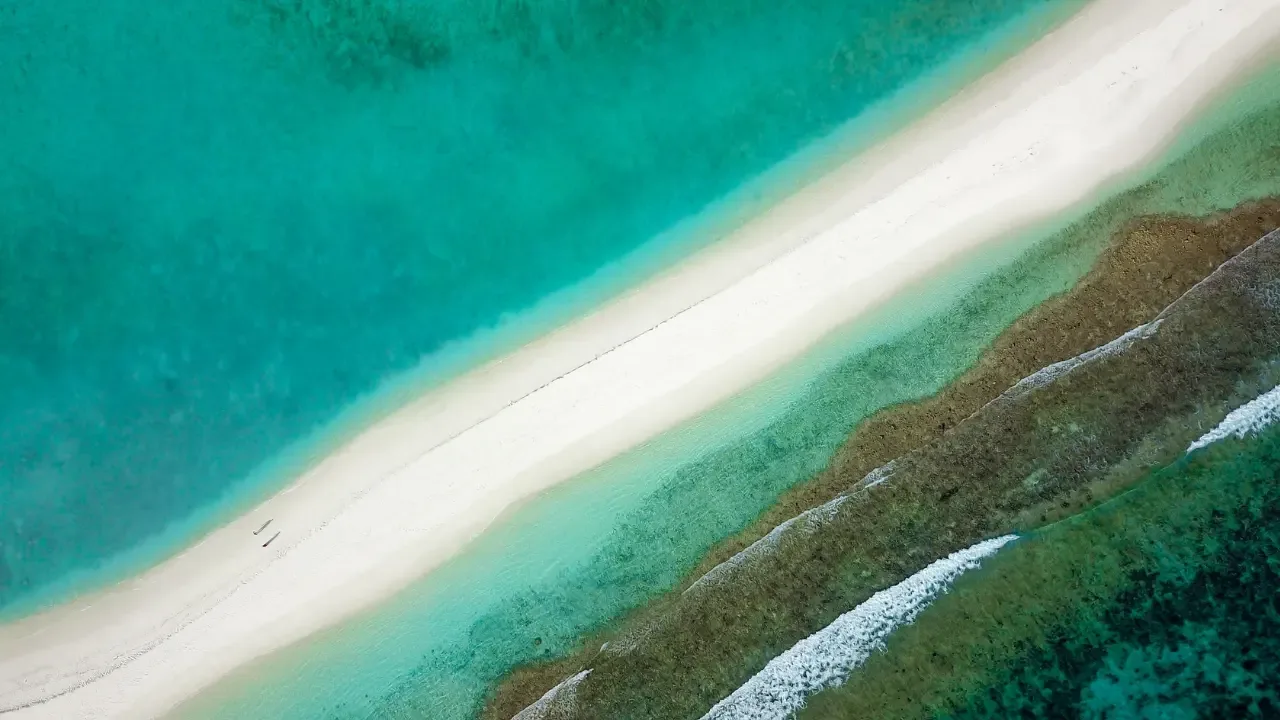
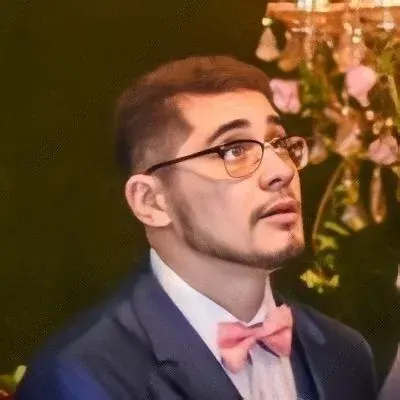
Why 'React' must be in scope when using JSX react/react-in-jsx-scope?
If you are new to React and encountered the error message "'React' must be in scope when using JSX react/react-in-jsx-scope", don't panic! This error often occurs when you forget to import the necessary React library.
Understanding the Error
In React, when you write JSX code, it gets transpiled to regular JavaScript, which uses the React library. To ensure that your JSX code is successfully transformed into JavaScript, you need to import the React library.
Possible Solutions
Here are a few easy solutions to fix the "'React' must be in scope when using JSX react/react-in-jsx-scope" error:
Solution 1: Add the missing import statement
In your code snippet, the import statement for the React library is missing. To fix this, make sure to import React at the beginning of your file:
import React from 'react'; // Add this line
class TechView extends React.Component { // Update this line
// ...
}
Solution 2: Destructure the React import
Another way to fix the error is to destructure the import statement. This allows you to directly use Component
instead of React.Component
in your code:
import React, { Component } from 'react'; // Add this line
class TechView extends Component { // Update this line
// ...
}
🎉 Congratulations! You fixed the error!
After applying one of the above solutions, try running your code again. The error should now be resolved, and your React component should render successfully without any issues.
If you're still encountering any problems, double-check that you have properly installed React in your project and that the React version matches the one you are trying to use.
Conclusion
Remember, when using JSX in React, always ensure that the React library is imported and in scope. This simple step will save you from encountering the "'React' must be in scope when using JSX react/react-in-jsx-scope" error.
I hope this guide helped you understand and resolve the issue you were facing. If you found this post helpful, consider sharing it with others who might be encountering the same problem.
Feel free to leave a comment below if you have any questions or suggestions. Happy coding! 👩💻🚀