React Hooks: useEffect() is called twice even if an empty array is used as an argument
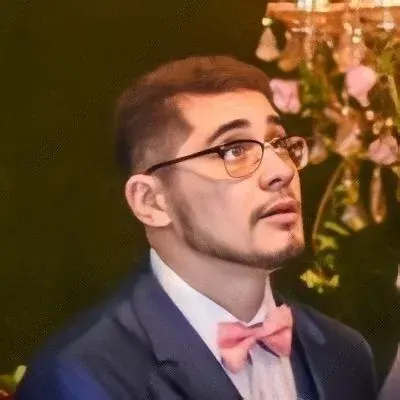
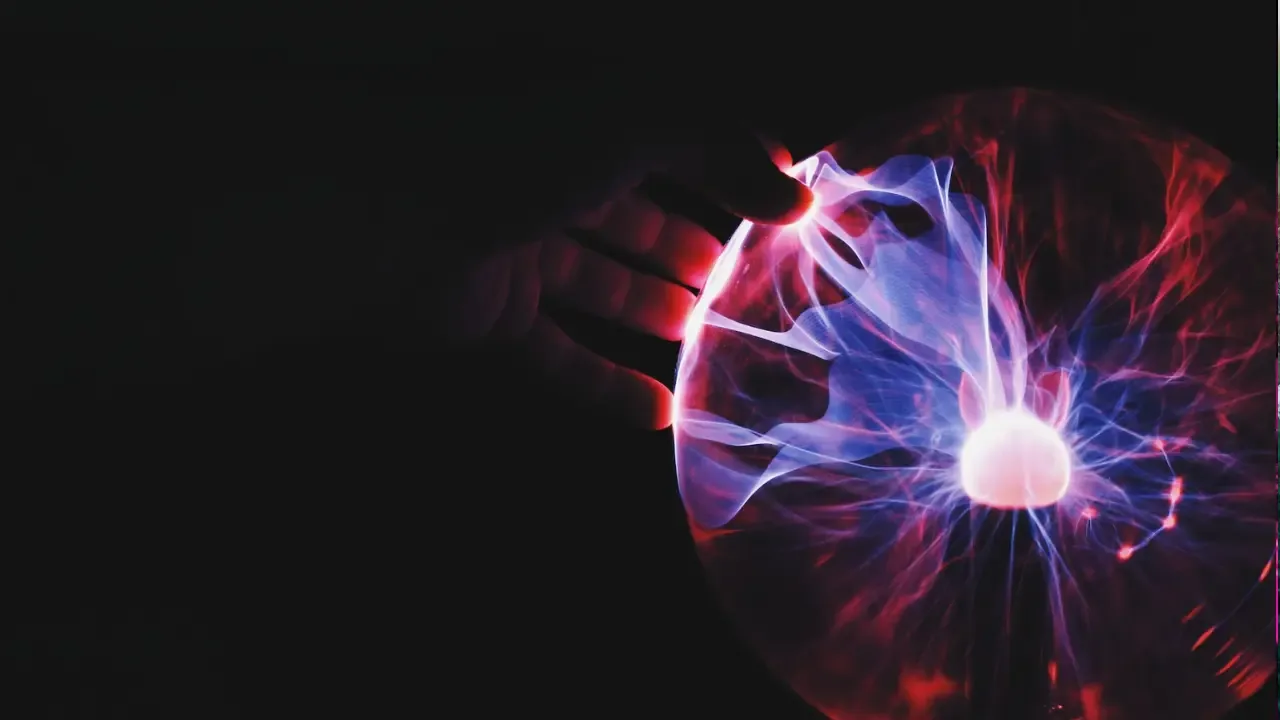
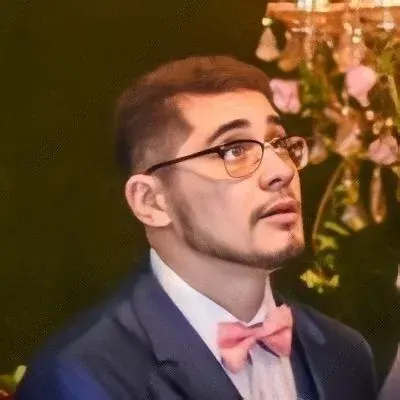
📝 Blog Post: React Hooks useEffect() is called twice even if an empty array is used as an argument
Are you new to ReactJS and encountering a problem where the useEffect() hook is being called twice, even with an empty array as an argument? 😩 Don't worry, you're not alone! Many developers have faced this issue, and we're here to help you understand why it happens and provide you with a proper fix.
Let's take a closer look at your code snippet:
import React from 'react';
import './App.css';
import { useState, useEffect } from 'react';
import Postspreview from '../components/Postspreview'
const indexarray = []; // The array to which the fetched data will be pushed
function Home() {
const [isLoading, setLoad] = useState(true);
useEffect(() => {
/*
Query logic to query from DB and push to indexarray
*/
setLoad(false); // To indicate that the loading is complete
}, []);
if (isLoading === true) {
console.log("Loading");
return <div>This is loading...</div>;
}
else {
console.log("Loaded!"); // This is actually logged twice.
return (
<div>
<div className="posts_preview_columns">
{indexarray.map(indexarray =>
<Postspreview
username={indexarray.username}
idThumbnail={indexarray.profile_thumbnail}
nickname={indexarray.nickname}
postThumbnail={indexarray.photolink}
/>
)}
</div>
</div>
);
}
}
export default Home;
🔍 Understanding the issue
The issue lies in how the useEffect() hook is being used. When you pass an empty array ([]), it tells React to only run the effect once, similar to the componentDidMount lifecycle method. However, in your code, the useEffect() hook seems to have some extra closing brackets "})" that are causing a syntax error.
💡 Solution
To fix the issue, remove the extra closing brackets and modify your code as follows:
import React, { useState, useEffect } from 'react';
import './App.css';
import Postspreview from '../components/Postspreview'
const indexarray = []; // The array to which the fetched data will be pushed
function Home() {
const [isLoading, setLoad] = useState(true);
useEffect(() => {
/*
Query logic to query from DB and push to indexarray
*/
setLoad(false); // To indicate that the loading is complete
}, []);
if (isLoading) {
console.log("Loading");
return <div>This is loading...</div>;
} else {
console.log("Loaded!"); // This will now be logged only once.
return (
<div>
<div className="posts_preview_columns">
{indexarray.map(indexarray =>
<Postspreview
username={indexarray.username}
idThumbnail={indexarray.profile_thumbnail}
nickname={indexarray.nickname}
postThumbnail={indexarray.photolink}
/>
)}
</div>
</div>
);
}
}
export default Home;
🎉 That's It!
By removing the extra closing brackets and ensuring that the condition in the if statement is more concise, you will now see the "Loaded!" message logged only once, avoiding the unnecessary double loading.
Don't hesitate to try it out and let us know if it solves your problem! If you have any more questions or need further assistance, feel free to reach out.
Happy coding! 💻🚀
📣 Engage with us!
We love hearing from our readers! If you found this blog post helpful, share it with your friends and colleagues who might be facing a similar issue. Let's help the community grow together! 🌱
Have any other questions or topics you'd like us to cover? Leave a comment below and we'll be sure to address them in future blog posts. Your feedback and engagement are highly appreciated! 💬
Stay tuned for more exciting tech tips and tricks! Follow us on social media for the latest updates:
Twitter: twitter.com/techblog
Facebook: facebook.com/techblog
Thanks for reading! Keep coding, keep smiling! 😄✨