Property "value" does not exist on type "Readonly<{}>"
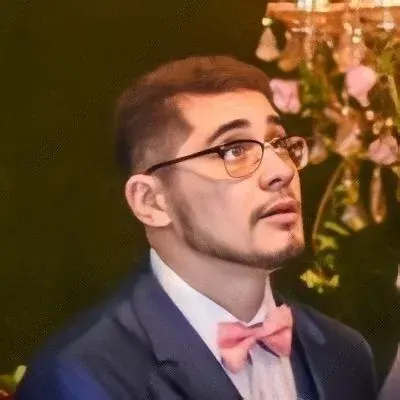
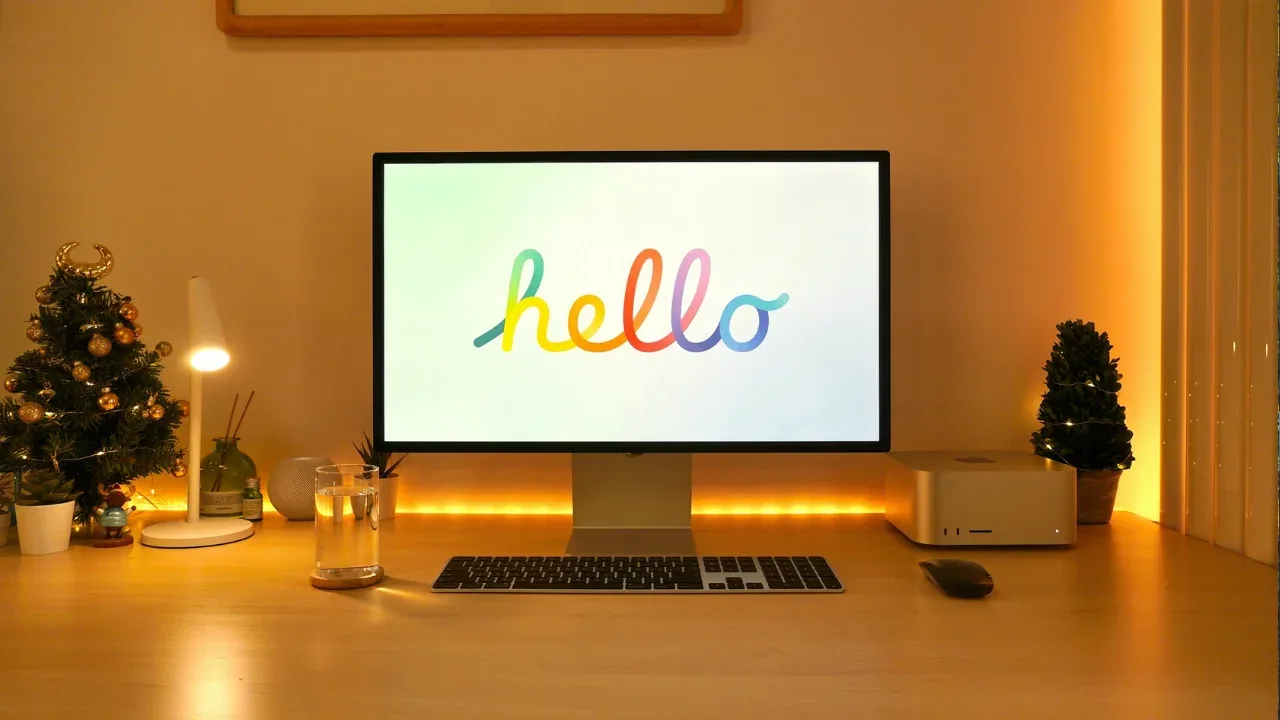
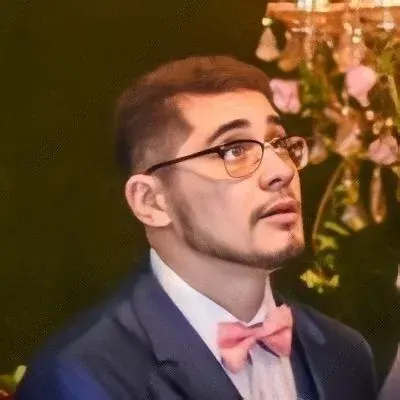
😱 The Dreaded Error: "Property 'value' does not exist on type 'Readonly<{}>'" 😱
So you're trying to create a form in React, displaying data based on an API return value. You followed the code from the official React documentation, but alas, an error has appeared! Fear not, for I am here to guide you through this predicament. Let's break it down, find the issue, and provide easy solutions. 💪
🤔 Understanding the Problem
The error Property 'value' does not exist on type 'Readonly<{}>'
usually occurs when TypeScript cannot find the correct type for a specific property in your code. In this case, it's the value
property in your component's state.
💡 Solution: Typing Your Component's State
To resolve this issue, you need to provide TypeScript with the correct type for your component's state. Currently, it is inferred as Readonly<{}>
, which doesn't have a value
property.
Create an interface to define your component's state and its properties. Add a
value
property of typestring
.
interface AppState {
value: string;
}
Update your component's class declaration to include the type of
state
, using the interface we just created.
class App extends React.Component<{}, AppState> {
// ...
In your component's constructor, initialize the state object with an empty string value for the
value
property.
constructor(props: {}) {
super(props);
this.state = { value: "" };
// ...
Finally, update any references to
this.state.value
andthis.setState
in yourhandleChange
andhandleSubmit
methods, using the correct state property type.
handleChange(event: React.ChangeEvent<HTMLInputElement>) {
this.setState({ value: event.target.value });
// ...
handleSubmit(event: React.FormEvent<HTMLFormElement>) {
alert("A name was submitted: " + this.state.value);
// ...
🎉 Celebrate! Problem Solved!
You've done it! By following these steps, you've successfully resolved the Property 'value' does not exist on type 'Readonly<{}>'
error. You've now typed your component's state properly, allowing TypeScript to recognize the value
property.
Feel free to check your code and run it again. The error should be gone, and you should be able to continue building your form with confidence. 🚀
📣 Join the Conversation!
Did this guide help you overcome the "Property 'value' does not exist on type 'Readonly<{}>'" error? We'd love to hear your success story! Share your experience and any tips you have for resolving TypeScript errors in the comments below.
Remember, don't let errors discourage you from creating awesome things. Keep coding, keep learning, and keep overcoming challenges. Happy coding! ⌨️💻