Programmatically navigate using react router V4
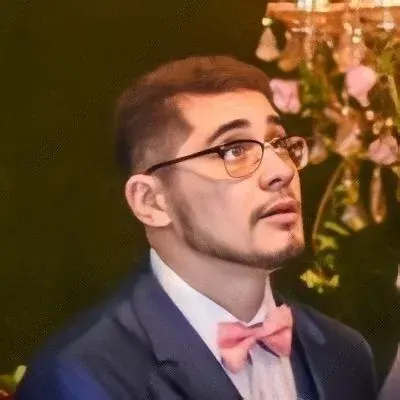
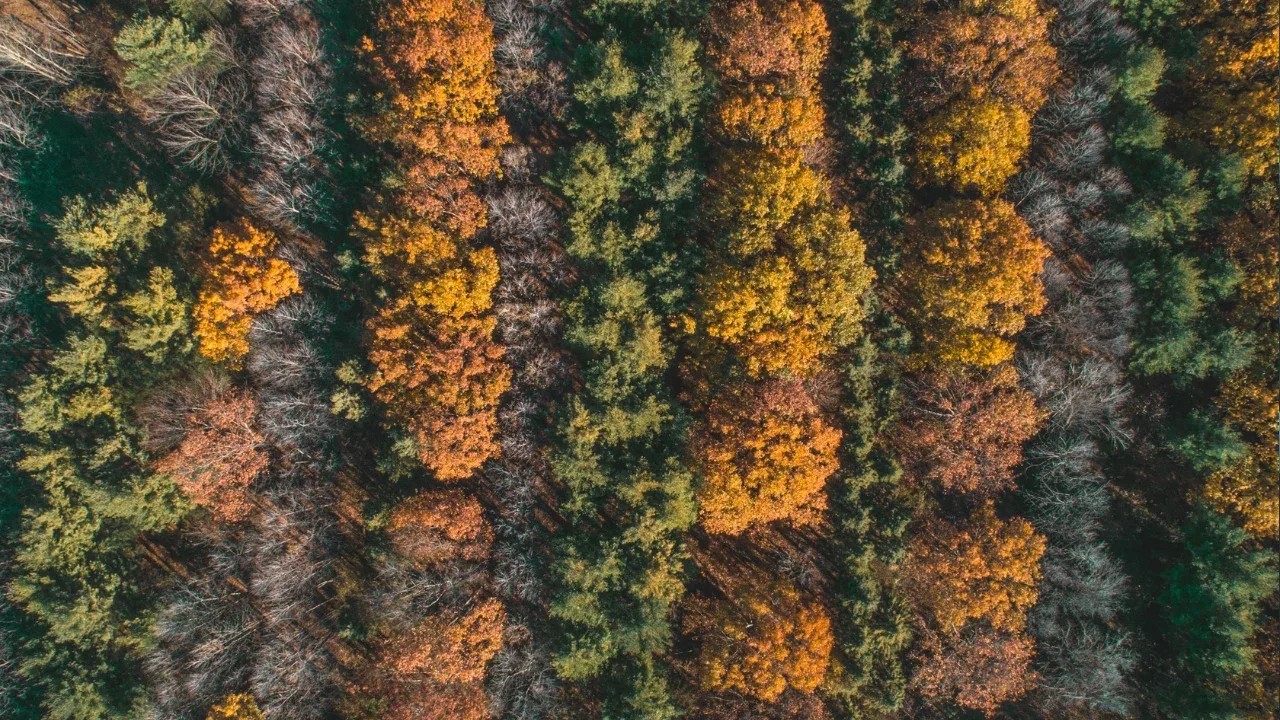
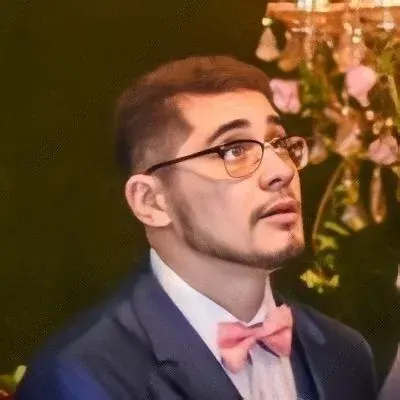
Programmatically navigate using React Router V4: A Complete Guide
š React Router is a popular routing library for React applications, providing a way to navigate between different pages or URL endpoints. In version 4 of React Router, there are some changes to how you can programmatically navigate within your components. š
ā ļø If you have recently upgraded from React Router v3 to v4, you may have noticed that the browserHistory
interface is no longer available. Instead, React Router v4 introduces a new approach to programmatic navigation. Let's explore how to navigate using React Router v4 step-by-step. š
Step 1: Installing React Router
First, make sure you have React Router v4 installed in your project. You can install it using npm or yarn:
npm install react-router-dom
# or
yarn add react-router-dom
React Router v4 uses the separate react-router-dom
package for web applications.
Step 2: Importing Required Modules
To start using React Router in your component, you need to import the required modules:
import { withRouter } from 'react-router-dom';
Step 3: Using withRouter
Wrap your component with the withRouter
higher-order component (HOC). This HOC will give your component access to the history
object, which you can use to navigate programmatically.
class YourComponent extends React.Component {
handleClick = () => {
// Programmatically navigate to /path/some/where
this.props.history.push('/path/some/where');
};
render() {
return (
<div>
{/* Component JSX */}
<button onClick={this.handleClick}>Navigate</button>
</div>
);
}
}
export default withRouter(YourComponent);
Step 4: Programmatically Navigating
Now, you can use the history.push
method inside your component's functions to navigate programmatically. In the example above, we are navigating to /path/some/where
when the button is clicked.
š” Remember, to use this.props.history.push
, you need to wrap your component with the withRouter
HOC.
That's it! You are now able to navigate programmatically using React Router v4. š
Conclusion
React Router v4 provides a straightforward way to handle programmatic navigation within your React components. By using the history
object provided by the withRouter
HOC, you can navigate to different routes based on user interactions or certain conditions.
š If you want to learn more about React Router and its features, make sure to check out the official documentation here.
Now, it's your turn! Give it a try and start navigating dynamically in your React Router v4 project. Feel free to comment below and share your experiences or any questions you may have. Happy coding! š»āØ