Is it possible to use if...else... statement in React render function?
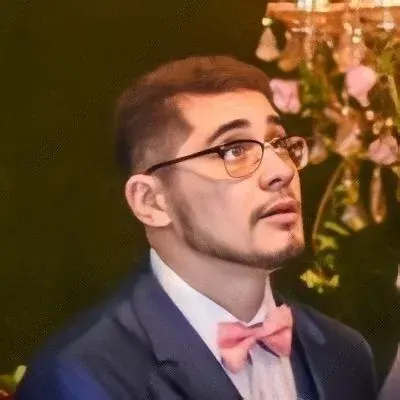
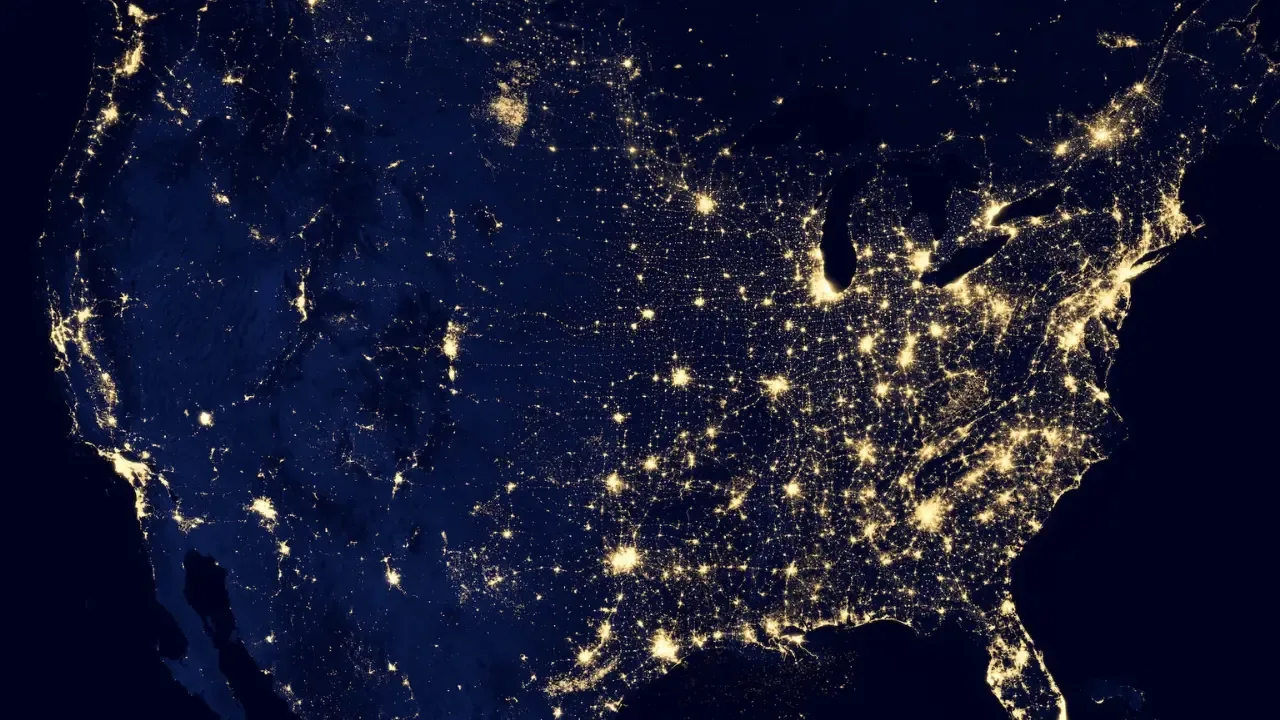
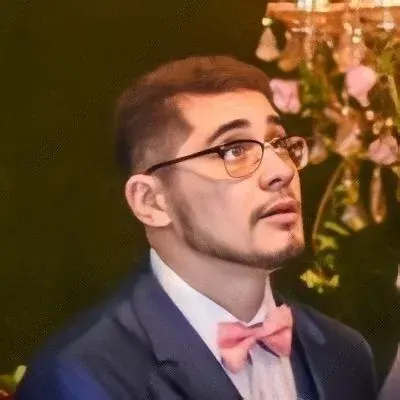
Is it possible to use if...else... statement in React render function? 🤔
So, you're working on a React component and you have come across a situation where you want to conditionally render different elements based on a certain prop. But, when you tried to use an if...else
statement inside the render()
function, it didn't work as expected. 😞
Don't worry, you're not alone! Many React developers have faced this issue before. The reason behind this is because the render()
function in React expects a single JSX element to be returned, rather than multiple elements or conditional statements.
For example, in the code snippet you provided, you tried to conditionally render an element based on the hasImage
prop. However, this approach doesn't work inside the render()
function because you're using an if...else
statement directly.
But fear not! There are a couple of simple and effective solutions to overcome this issue. Let's dive into them right away. 💪
Solution 1: Using a Variable
One way to conditionally render elements is by storing the JSX element in a variable, and then returning that variable inside the render()
function. Here's how you can do it:
render() {
let elementToRender;
if (this.props.hasImage) {
elementToRender = <ElementWithImage />;
} else {
elementToRender = <ElementWithoutImage />;
}
return (
<div>
<Element1/>
<Element2/>
{elementToRender}
</div>
);
}
In this solution, we declare a variable called elementToRender
and assign the appropriate JSX element based on the condition. Finally, we render that variable inside the return
statement.
Solution 2: Using the Ternary Operator
Another concise way to conditionally render elements is by using the ternary operator (condition ? expression1 : expression2
). This operator allows us to write the condition and choose between two expressions based on that condition.
Here's how you can utilize the ternary operator to conditionally render elements in your render()
function:
render() {
return (
<div>
<Element1/>
<Element2/>
{this.props.hasImage ? <ElementWithImage /> : <ElementWithoutImage />}
</div>
);
}
In this solution, we directly use the ternary operator inside the JSX expression to evaluate the condition and render the appropriate element accordingly.
Now that you have learned these easy solutions, you can leverage them to conditionally render elements inside the render()
function of your React components without any hassle. 🙌
Conclusion
To sum it up, while using if...else
statements directly inside the render()
function of React components might not work as expected, you can overcome this limitation by either using a variable or the ternary operator to conditionally render elements. These techniques will make your code more readable, maintainable, and aligned with React's requirements.
So, go ahead and try out these solutions in your React projects, and let us know how it goes! Feel free to share your thoughts, experiences, or any other alternative approaches you've discovered in the comments below. 👇 Happy coding!