Inline CSS styles in React: how to implement a:hover?
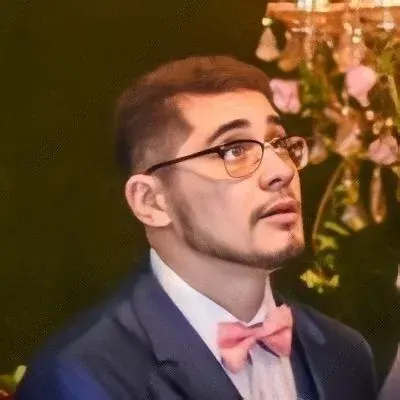
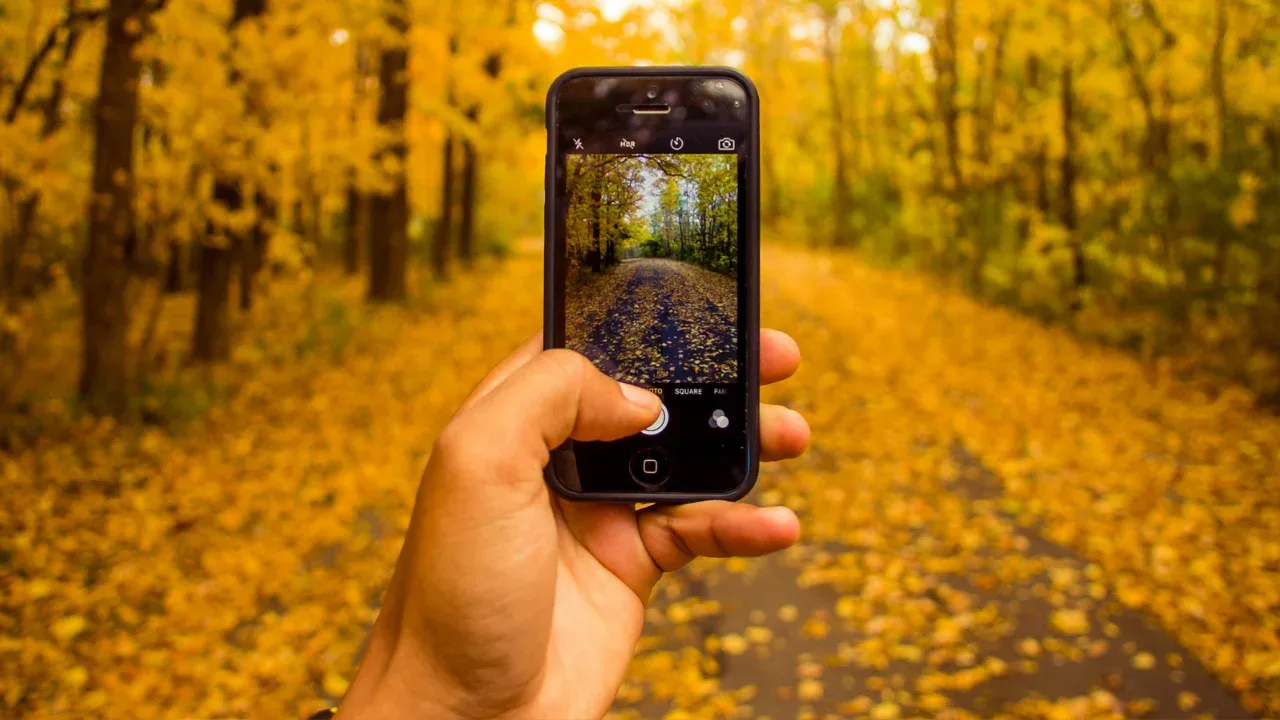
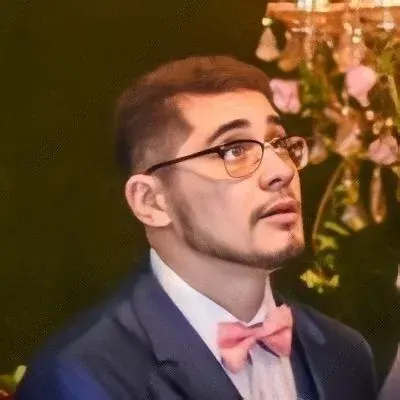
🔍 Inline CSS styles in React: how to implement :hover
?
If you're a fan of the inline CSS pattern in React, you might have encountered some roadblocks when trying to implement the :hover
selector. The good news is that there are easy and effective ways to achieve hover effects while using inline CSS styles.
💡 The Challenge
Inline CSS styles don't directly support pseudo-selectors like :hover
, which poses a challenge when you want to apply hover effects to your React components. However, fear not! There are alternative approaches that will allow you to implement the :hover
functionality smoothly.
💪 Solution 1: Using a Clickable Component
One suggested solution from the React community is to create a Clickable
component that manages the hover state and passes it as props to the desired component, like <Link />
. Here's an example:
<Clickable>
<Link />
</Clickable>
The Clickable
component wraps the Link
component and sets the onMouseEnter
and onMouseLeave
events to trigger the hover effects. However, if the Clickable
component is implemented by wrapping the child component in a <div>
, it can cause some unintended behaviors.
🚀 Simpler Solution: Using CSS Modules
If you're looking for a simpler and more straightforward solution, consider using CSS Modules. CSS Modules allow you to write CSS in a modular and scoped manner, making it easier to manage styles and apply hover effects.
Here's an example of how you can implement hover effects using CSS Modules:
import styles from './Link.module.css';
const Link = () => {
return (
<a className={styles.link} href="#">My Link</a>
);
};
In this example, we import the CSS module for the Link
component and apply the appropriate class name (styles.link
) to the <a>
tag. Within the CSS module, you can define the hover effects:
.link:hover {
/* Your hover styles */
color: blue;
text-decoration: underline;
}
With CSS Modules, you can enjoy the benefits of inline CSS while also being able to apply hover styles easily and intuitively.
🔥 Engage and Share Your Thoughts!
Implementing hover effects in inline CSS styles can be a bit tricky, but with the right approach, you can achieve the desired functionality. Which solution do you prefer? Have you encountered any challenges, or do you have additional tips to share? Let us know in the comments below and share this post with fellow React enthusiasts! 🎉