In general is it better to use one or many useEffect hooks in a single component?
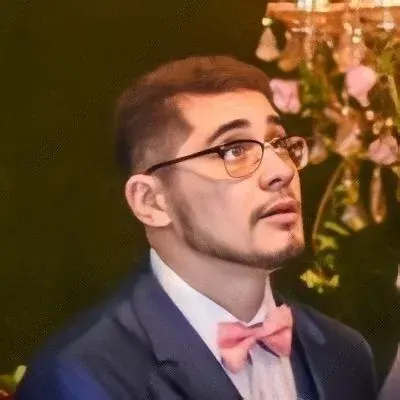
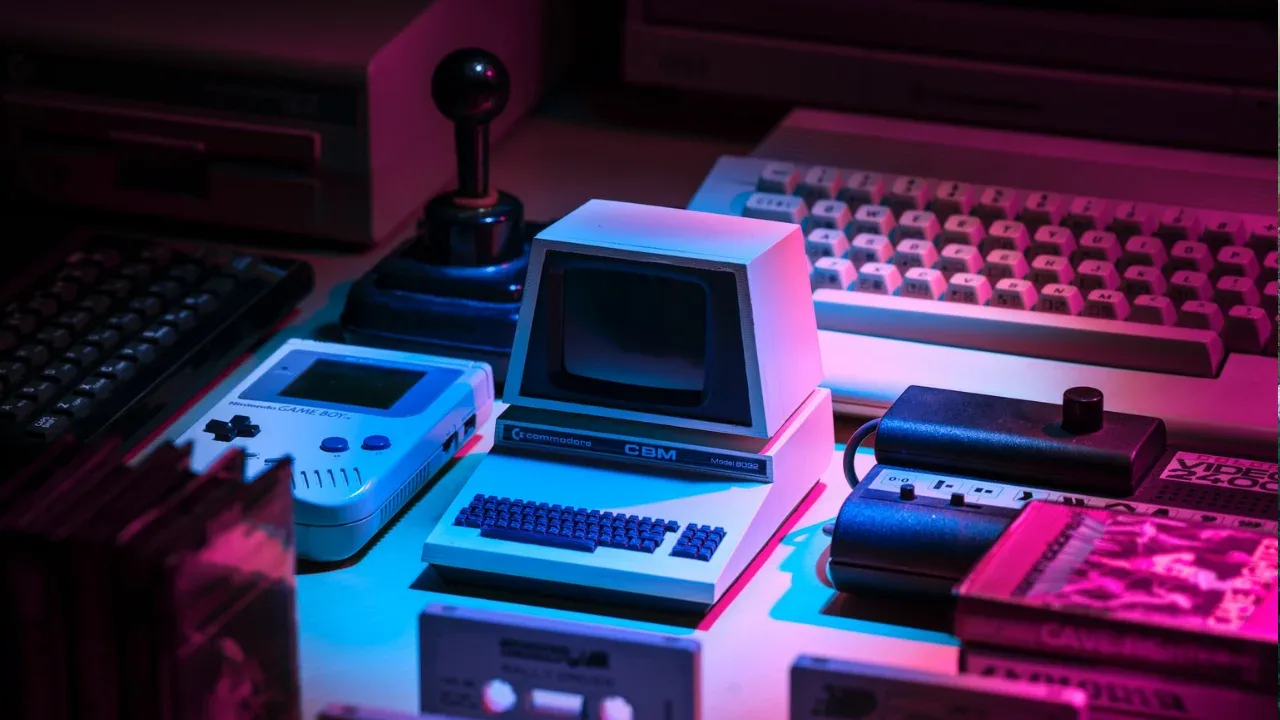
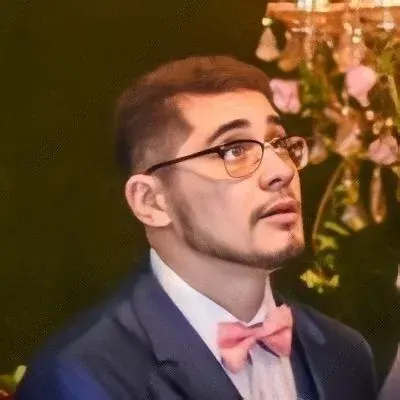
One useEffect or Many useEffects? Which is Better?
š§ So you're building a React component and you have some side effects to apply. You're wondering how to organize them - should you use one useEffect or break them up into several useEffects? It's a common question that many React developers face. But fear not! In this blog post, we'll dive into the pros and cons of both approaches and help you make an informed decision.
The Context š
Let's paint the picture. You have a React component, and it needs to perform some actions when certain conditions are met. These actions could include updating state, fetching data from an API, subscribing to events, or manipulating the DOM. In React, you can achieve this by leveraging the useEffect hook, which allows you to perform side effects in a functional component.
The Dilemma ā
Now comes the question - should you group all these side effects into a single useEffect or split them into multiple useEffects? Both approaches have their advantages and disadvantages, so let's analyze them.
Single useEffect approach
By using a single useEffect, you have all your side effects in one place, making it easier to see and understand what's happening in your component. This approach can be beneficial when your side effects rely on the same dependencies and should trigger simultaneously.
Here's an example:
useEffect(() => {
// Side effect 1
// ...
// Side effect 2
// ...
}, [dependency1, dependency2]);
In this case, both side effect 1 and side effect 2 will be triggered whenever either dependency1
or dependency2
changes.
Multiple useEffects approach
On the other hand, breaking down your side effects into separate useEffects allows for better granularity and organization. This can be handy when you have unrelated or complex side effects that shouldn't run every time a specific dependency changes. It also improves reusability since individual useEffects can be easily extracted and reused in other components.
Here's an example:
useEffect(() => {
// Side effect 1
// ...
}, [dependency1]);
useEffect(() => {
// Side effect 2
// ...
}, [dependency2]);
In this case, side effect 1 will only execute when dependency1
changes, and side effect 2 will only execute when dependency2
changes.
Deciding Factors ā
Now that we've discussed the two approaches, how do you decide which one is better for your use case? Here are a few factors to consider:
Dependencies: If your side effects rely on different sets of dependencies, it might be better to use multiple useEffects. This ensures that each effect only runs when its specific dependencies change.
Readability: If your side effects are closely related and share dependencies, using a single useEffect can make your code more readable and easier to follow. It keeps all the related logic in one place.
Performance: In terms of performance, both approaches are generally comparable. React is intelligent enough to optimize the execution of multiple useEffects. However, if you find that your component's rendering is becoming slow due to excessive side effects, consider refactoring them for optimization.
A Compromise š”
If you're still torn between the two approaches, you can always strike a balance by using a combination of both. Group side effects that are closely related in a single useEffect and break down unrelated or complex side effects into separate useEffects. This way, you get the best of both worlds - organization and granular control.
Conclusion š
In the end, there's no one-size-fits-all solution. Choosing between one useEffect or many useEffects in a single component depends on your specific use case, readability, and maintainability. Assess your dependencies, consider the complexity of your side effects, and find the approach that works best for your project.
So, which approach will you choose for your side effects? Let us know in the comments below, and happy coding! šš»
Was this blog post helpful? We love to hear your feedback! Don't forget to share it with your fellow developers and join our community for more insightful content on React. Together, we can level up our coding game! šš¬