How to use refs in React with Typescript
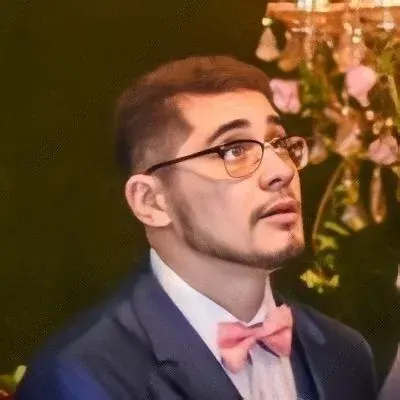
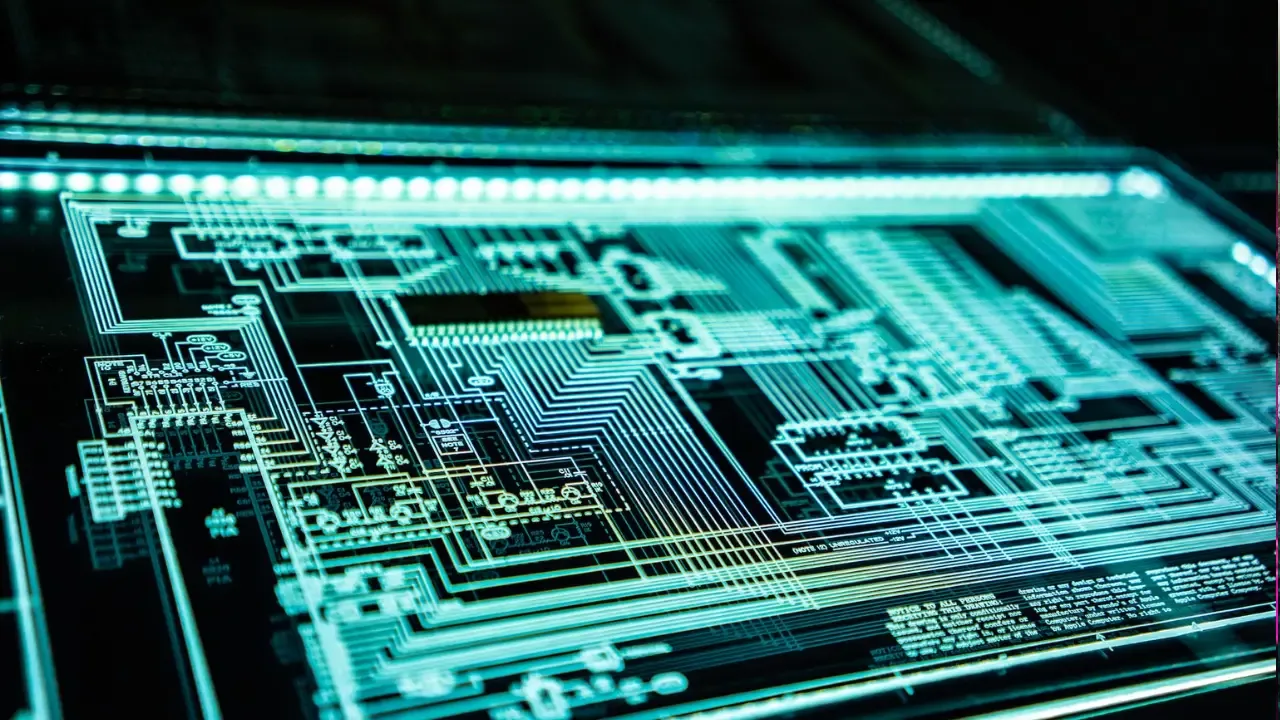
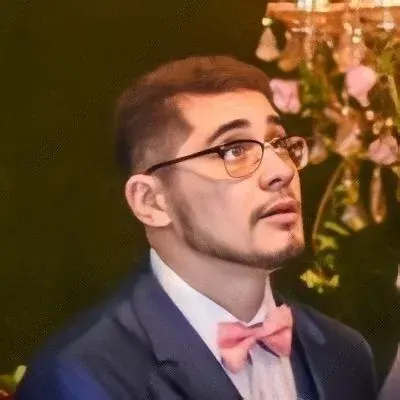
📝 Title: Mastering Refs in React with Typescript
Introduction
Are you struggling to understand how to use refs in React while also enjoying the benefits of static typing and IntelliSense in Typescript? You're not alone. In this guide, we'll demystify the process of using refs in React with Typescript and provide easy solutions to common problems.
Understanding the Problem
Let's start by examining the code example you provided. The AppRefs
interface suggests that you're attempting to use refs to reference React nodes. However, the current implementation falls short of achieving the desired static typing and IntelliSense.
The main issue lies in using the ref
attribute as a string (ref="stepInput"
), which yields an untyped reference to the HTML element rather than a reference to the specific React component. This leads to a lack of type-checking capabilities and IntelliSense support.
Easy Solution
To overcome this problem and harness the power of static typing and IntelliSense, follow these simple steps:
Import the useRef hook: In your React component file, import the useRef hook from the 'react' library.
Define a Ref Type: Create a type that matches the expected reference (in this case, an HTMLInputElement).
import * as React from 'react';
// ...
type InputRef = React.RefObject<HTMLInputElement>;
Declare Ref Variables: Declare the ref variable(s) within your component and assign a new ref object using the
useRef
hook.
const stepInput: InputRef = React.useRef<HTMLInputElement>(null);
Assign Ref to the Element: In the JSX code, assign the ref variable to the desired element using the
ref
attribute.
<input type="text" ref={stepInput} />
Accessing the Value: To access the value of the input element, use
stepInput.current.value
wherever required.
With these steps, you've successfully implemented refs with static typing and IntelliSense in React with Typescript.
Take it to the Next Level
Now that you've mastered the art of using refs in React with Typescript, it's time to level up your development skills! Experiment with more advanced scenarios, such as:
Using multiple refs to reference different elements within your component.
Utilizing callbacks with refs to perform actions on the referenced elements.
Exploring third-party libraries that enhance the ref experience with additional features.
Conclusion
By following these easy steps, you can unlock the full potential of refs in React while enjoying the benefits of static typing and IntelliSense in Typescript. Say goodbye to guessing games and hello to a more efficient and enjoyable coding experience.
We hope this guide has been helpful in addressing any confusion or frustrations you had regarding refs in React with Typescript. Give it a go, share your experiences, and stay curious!
🌟 Remember, the learning journey never ends. Keep evolving and keep coding! 🔥
What were your experiences with using refs in React with Typescript?
Have you encountered any other challenges while working with these technologies?
Share your thoughts and experiences in the comments below! 🚀