How to use comments in React
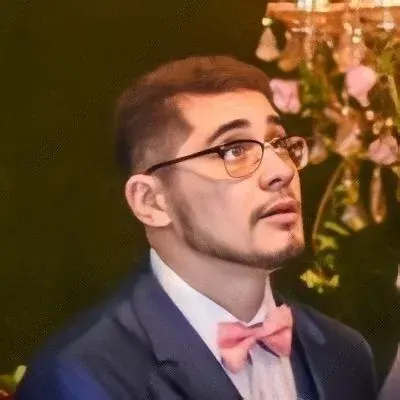
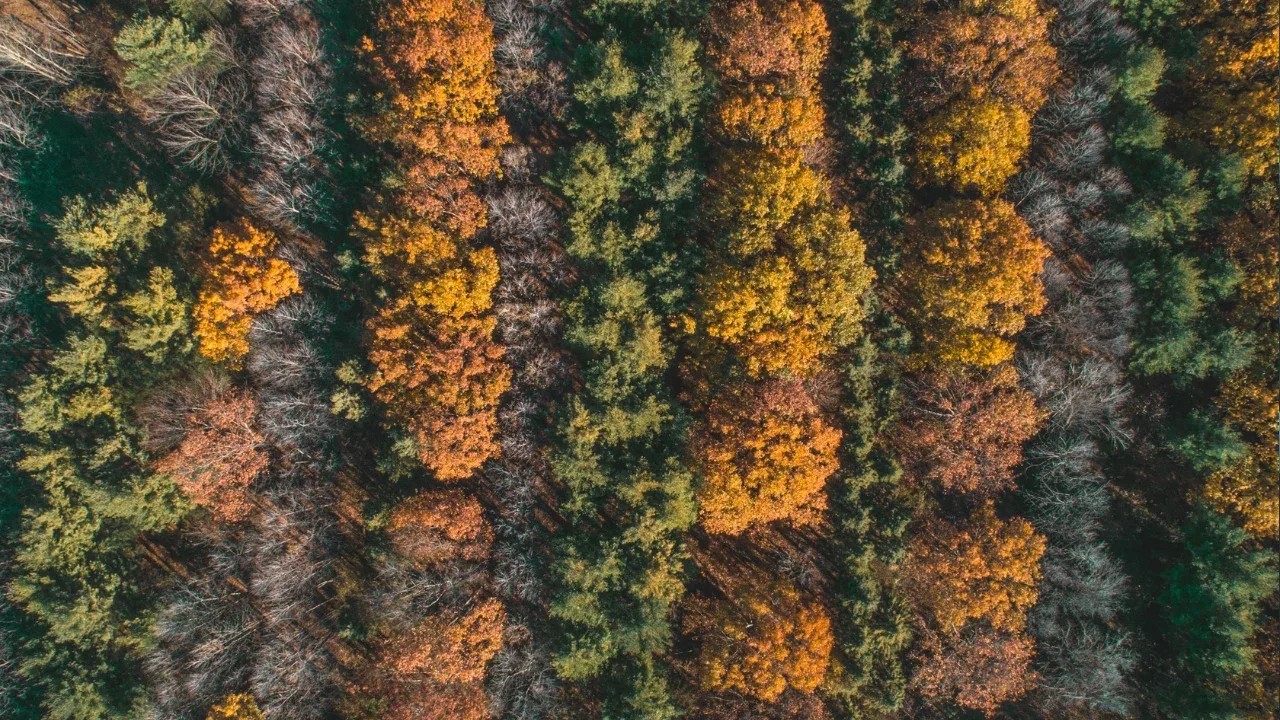
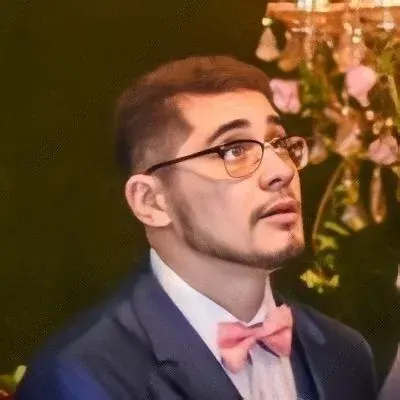
How to Use Comments in React 🗣️
So you're working with React and you want to use comments inside the render
method of your component, huh? 😮 Well, don't fret! I'm here to guide you through it. 👍
The Common Problem 😕
Let's take a look at the example code provided:
'use strict';
var React = require('react'),
Button = require('./button'),
UnorderedList = require('./unordered-list');
class Dropdown extends React.Component {
constructor(props) {
super(props);
}
handleClick() {
alert('I am click here');
}
render() {
return (
<div className="dropdown">
// whenClicked is a property not an event, per se.
<Button whenClicked={this.handleClick} className="btn-default" title={this.props.title} subTitleClassName="caret"></Button>
<UnorderedList />
</div>
)
}
}
module.exports = Dropdown;
As you can see, the developer attempted to use a comment inside the render
method. However, when rendered on the UI, the comment is visible. 😱
The Solution 🛠️
React treats anything between angle brackets as JSX, which means comments inside angle brackets are also rendered as regular content. In order to use comments that won't appear in the UI, we need to use the JavaScript block comment syntax.
In the provided code, the comment // whenClicked is a property not an event, per se.
should be modified as follows:
{/* whenClicked is a property not an event, per se. */}
This JavaScript block comment wrapped in curly braces will now be treated as an actual comment and will not be displayed on the UI. 🎉
Alternative Approach 🔄
If you prefer to use single-line comments, you can accomplish this by using double curly braces:
{/*
This is a single-line comment.
It won't be visible on the UI.
*/}
The Call-to-Action 👋
And there you have it! Now you know how to use comments in React without making them appear in the UI. 💡
But wait, I want to hear from you! Have you encountered any other common issues while working with React? Let me know in the comments below! Let's share our knowledge and help each other out. 🌟