How to set default Checked in checkbox ReactJS?
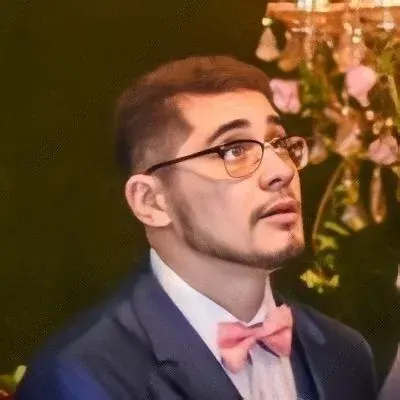
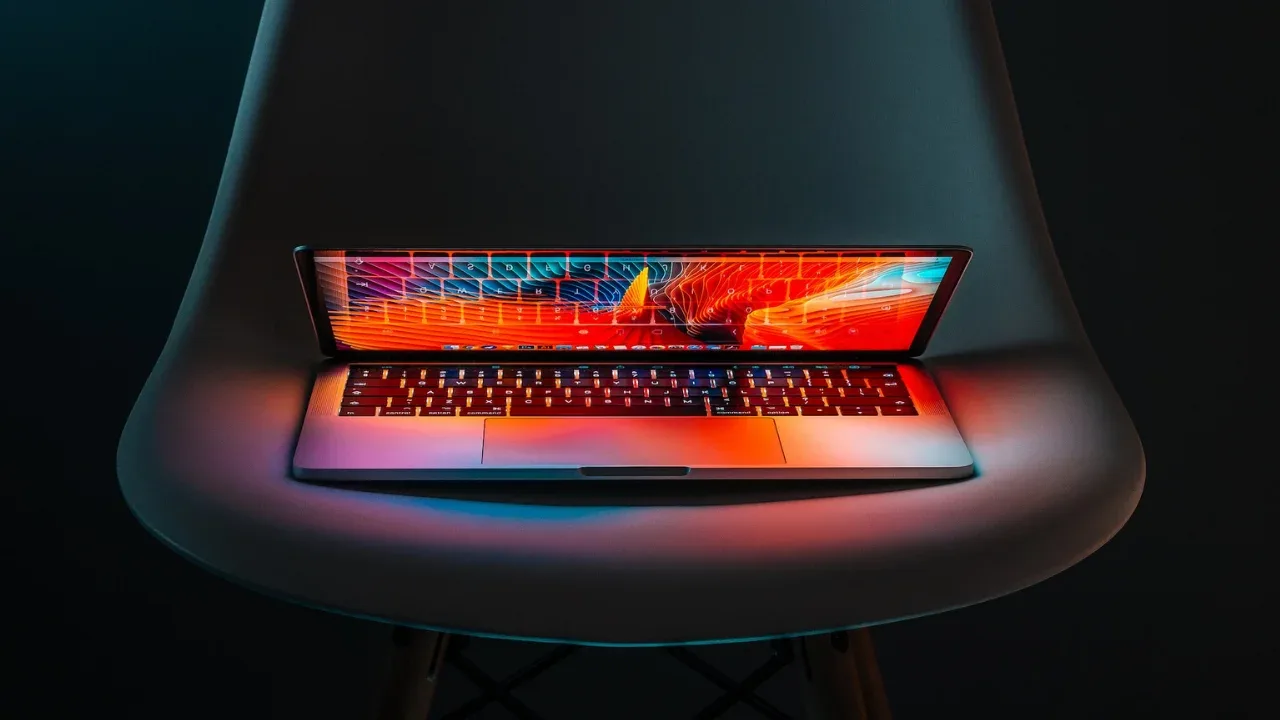
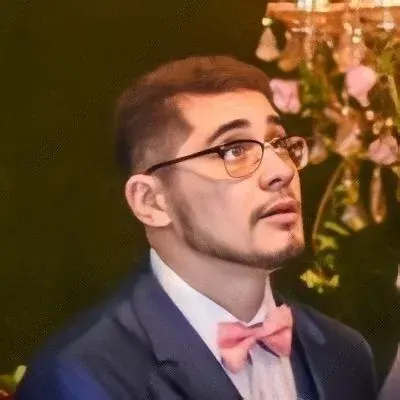
How to Set Default Checked in Checkbox ReactJS? 😕🔧
Are you having trouble with setting the default checked value in a checkbox using ReactJS? 🤔 Don't worry, you're not alone! Many developers face issues when they try to assign the checked
attribute with the value "checked"
in React. However, there's a simple solution to this problem!
The Issue 🤷♂️
After assigning checked="checked"
, you may find that you cannot interact with the checkbox state by clicking to uncheck/check it. This can be quite frustrating, especially when you're expecting the checkbox to be pre-selected but also allow user interaction. 😩
The Solution 💡👨💻
To set the default checked value and still have user interaction with the checkbox, you need to use the defaultChecked
attribute instead of checked
.
Here's how you can do it in React:
import React from 'react';
function Checkbox() {
return (
<input
type="checkbox"
defaultChecked={true}
// You can also use defaultChecked={false} if you want the checkbox to be unchecked by default
/>
);
}
export default Checkbox;
By using defaultChecked
, you can now have the checkbox pre-selected while also allowing the user to interact with it as expected. 🙌
Example Usage 🌟
Let's say you have a form where you want to include a checkbox that is pre-selected by default. You can use the defaultChecked
attribute within your checkbox component like this:
import React from 'react';
function MyForm() {
return (
<form>
<h2>My Form</h2>
<Checkbox />
{/* Add more form elements here */}
</form>
);
}
export default MyForm;
Now, whenever you render the MyForm
component, the checkbox will be pre-selected, and users can still interact with it.
Get Rid of Checkbox Initialization Troubles! 🚫🤯
With this simple solution, you can set the default checked value in a checkbox using ReactJS without sacrificing user interactivity. No more struggling with checkbox initialization troubles! 😌
So, go ahead and implement this solution in your project, and let us know how it worked for you. Feel free to share your experiences in the comments section below and help fellow developers tackle this issue as well. Remember, sharing is caring! ❤️️
Happy coding! 👨💻💻