How to push to History in React Router v4?
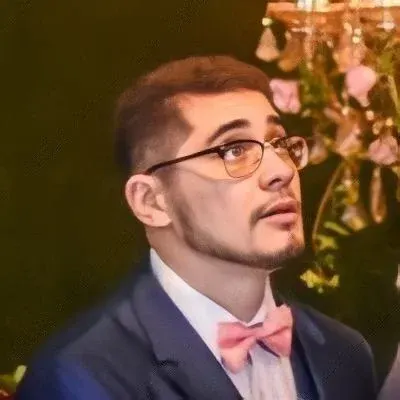
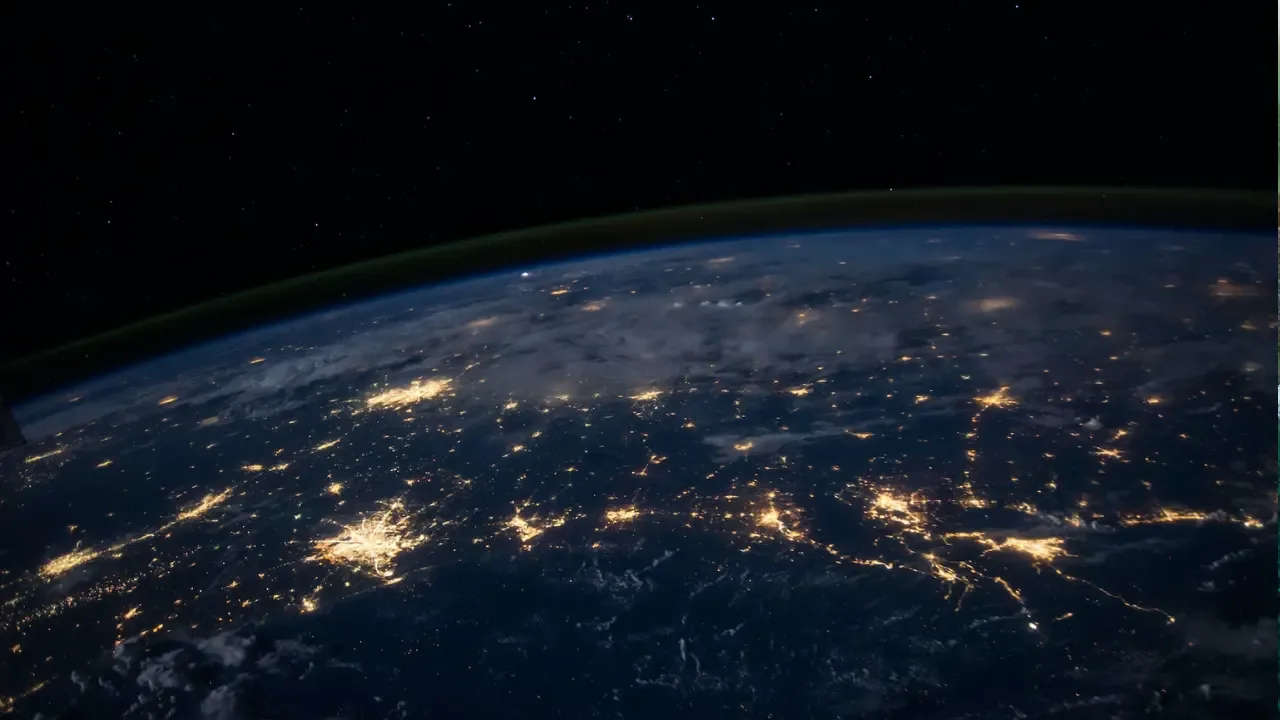
📝 🚀 How to Push to History in React Router v4?
Are you struggling to find the appropriate way to handle the browser history in React Router v4? In the previous version (v3), you could use browserHistory.push
to redirect to a specific page. However, this method is no longer available in v4, and you may be wondering how to achieve the same functionality. Don't worry, we've got you covered with some easy solutions!
🔧 Solution 1: Using the withRouter
Higher Order Component
In React Router v4, you can leverage the withRouter
higher order component to access the history
object and navigate programmatically. Here's how you can use it:
Import the withRouter HOC from the react-router-dom package:
import { withRouter } from 'react-router-dom';
Wrap your component using the withRouter HOC:
class AppProductForm extends React.Component { // ... } export default withRouter(AppProductForm);
Now, you can access the
history
object in your component's props and use it to push to a specific page. Here's an example:export function addProduct(props) { return dispatch => axios.post(`${ROOT_URL}/cart`, props, config) .then(response => { dispatch({ type: types.AUTH_USER }); localStorage.setItem('token', response.data.token); props.history.push('/cart'); }); }
By wrapping your component with withRouter
, you can access the history
object and use the push
method to redirect to the desired page.
🔧 Solution 2: Using the useHistory
Hook
If you prefer using functional components and hooks, React Router v5 introduced the useHistory
hook, which allows you to access the history
object. Here's how you can apply this solution:
Import the useHistory hook from the react-router-dom package:
import { useHistory } from 'react-router-dom';
Use the useHistory hook in your functional component:
function AppProductForm() { const history = useHistory(); // ... }
You can then use the
history
object to push to the desired page:export function addProduct(props) { const history = useHistory(); return dispatch => axios.post(`${ROOT_URL}/cart`, props, config) .then(response => { dispatch({ type: types.AUTH_USER }); localStorage.setItem('token', response.data.token); history.push('/cart'); }); }
With the useHistory hook, you can access the history
object directly within your functional component and use the push
method for navigation.
💡 Remember to import the necessary dependencies (react-router-dom
, etc.) according to your project setup.
🔥 Ready to Supercharge Your React Router v4 Experience?
Implementing navigation and history in React Router v4 doesn't have to be a daunting task. By using the solutions we've discussed, you can easily achieve the desired redirect functionality. Remember to choose the approach that aligns with your coding style and project requirements – either the withRouter
higher order component or the useHistory
hook.
💬 Let us know in the comments below which solution you found most helpful or if you have any questions. Sharing is caring, so if you found this post useful, spread the word to help other developers facing the same challenge!
Happy coding! 😄🚀
Take Your Tech Career to the Next Level
Our application tracking tool helps you manage your job search effectively. Stay organized, track your progress, and land your dream tech job faster.
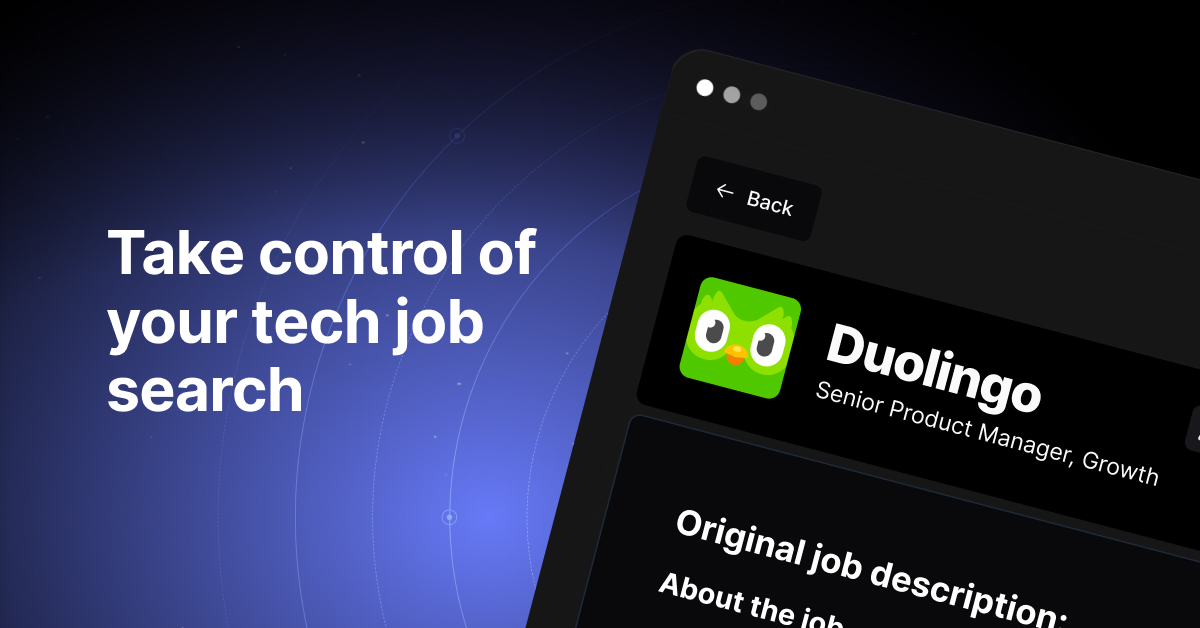