How to pass params with history.push/Link/Redirect in react-router v4?
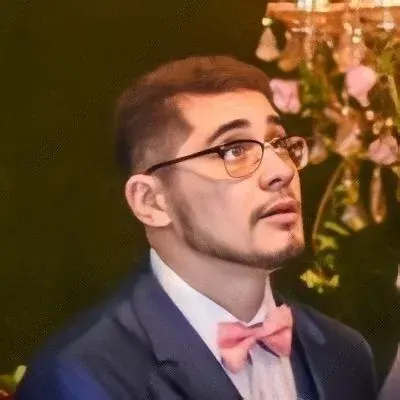
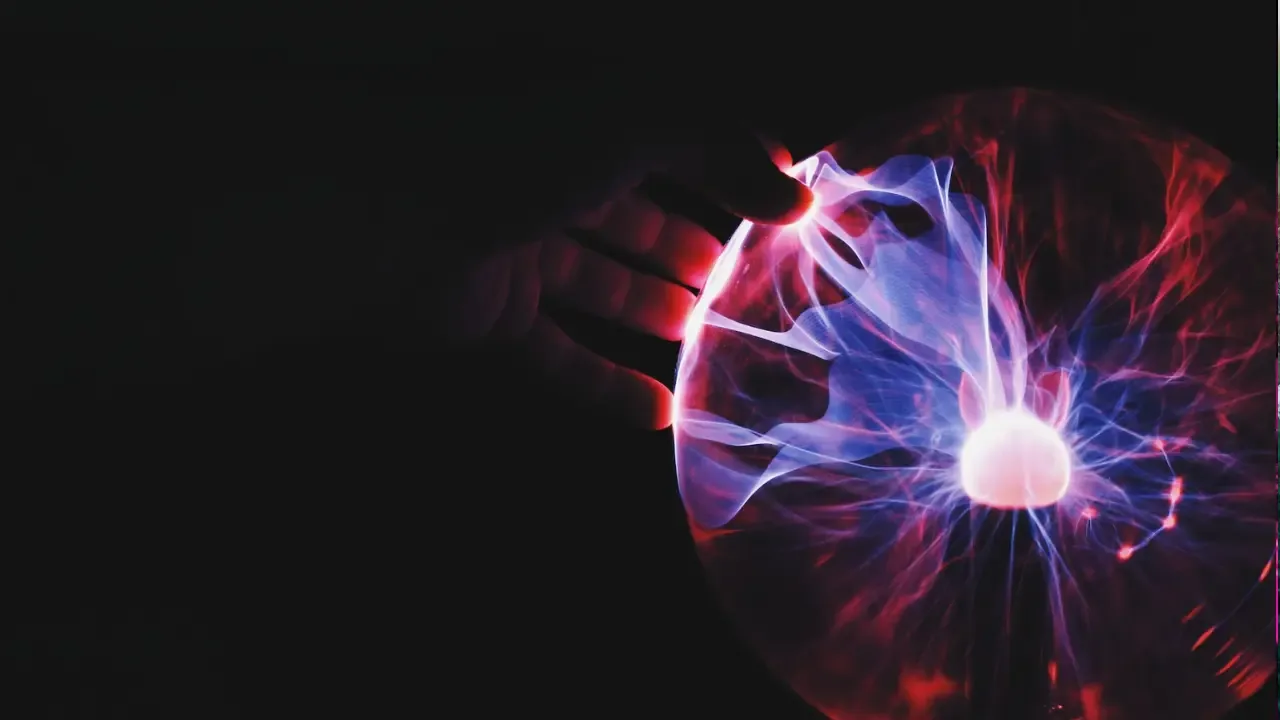
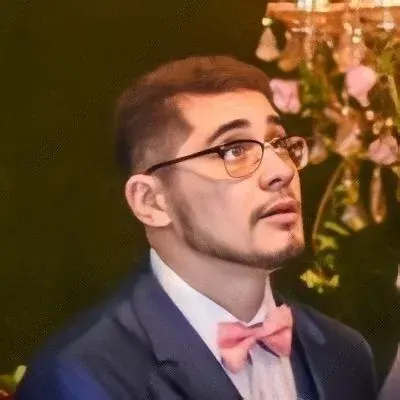
🚀 How to Pass Params with history.push/Link/Redirect in React-Router v4?
Are you struggling to pass parameters with history.push
, Link
, or Redirect
in React-Router v4? Don't worry, you're not alone! Many developers face this issue when working on React projects. But fear not, because in this blog post, we'll demystify this problem and provide you with easy solutions.
💡 Understanding the Problem
When using React-Router v4, passing parameters through history.push
can be a bit tricky. The traditional approach of appending the parameter directly to the URL might not work as expected. For example, if you try the following:
this.props.history.push('/page?id=123');
You'll notice that the URL won't change, and the parameter won't be passed correctly. So how can we pass parameters using history.push
?
🎯 Solution: React-Router's Location State
React-Router v4 provides a simple and effective solution for passing parameters using history.push
. Instead of appending the parameter to the URL, we can use the location
state to pass the parameter.
Here's how you can do it:
this.props.history.push({
pathname: '/page',
state: {
id: 123
}
});
By passing an object to history.push
with a pathname
and state
, you can now access the parameter in your target component.
🌈 Accessing the Parameter in the Target Component
To access the parameter in the target component, you can use this.props.location.state
.
For example, if you want to access the id
parameter in the /page
component, you can do the following:
const { id } = this.props.location.state;
Now you have access to the parameter and can use it as per your requirement.
🚀 Passing Params with Link and Redirect
The same technique can be used with the <Link>
and <Redirect>
components as well. Here's how you can pass parameters using these components:
<Link
to={{
pathname: '/page',
state: {
id: 123
}
}}
>
Go to Page
</Link>
<Redirect
to={{
pathname: '/page',
state: {
id: 123
}
}}
/>
With these components, you can easily pass parameters in the state
object and access them in the target components.
📣 Take It to the Next Level
Now that you've learned how to pass parameters with history.push
, Link
, and Redirect
in React-Router v4, it's time to put your newfound knowledge into action! Try implementing this technique in your React projects and see the magic happen.
Share your success stories, implementations, and any other questions you may have in the comments below. Let's build a thriving community of React developers who can tackle any problem together!
Happy coding! 👩💻👨💻