How to pass data from child component to its parent in ReactJS?
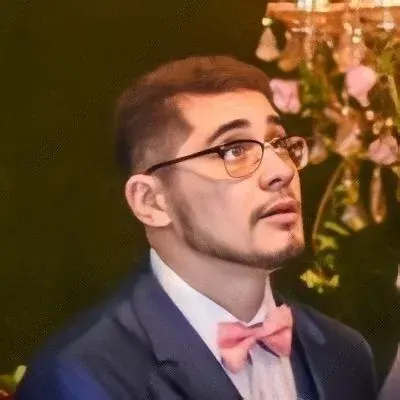
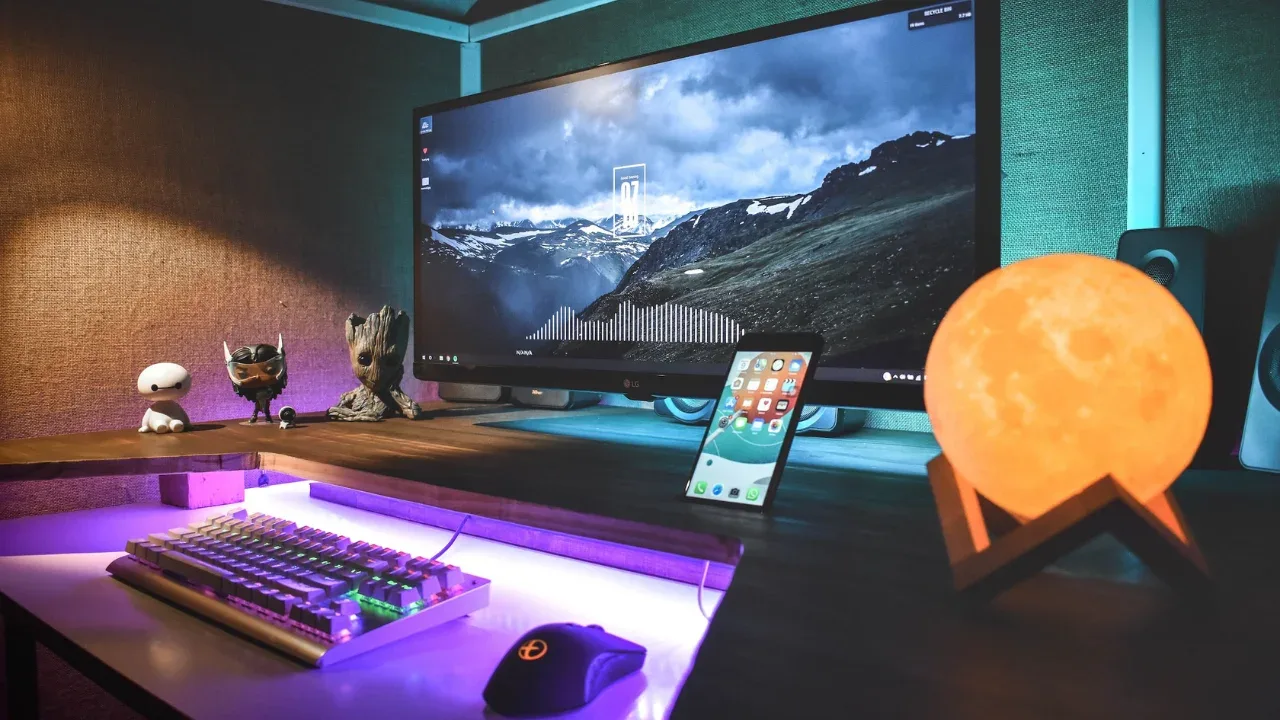
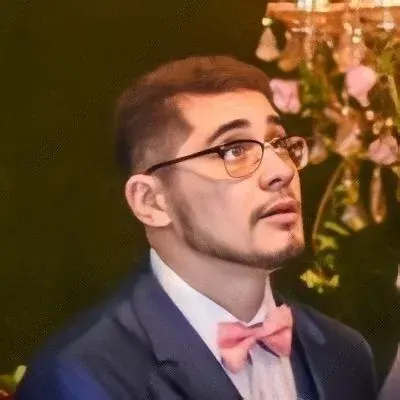
How to Pass Data from Child Component to Parent in ReactJS 💡
Hey there! 👋 Are you struggling with passing data from a child component to its parent in ReactJS? You're not alone! This can be a tricky task, but fear not – I'm here to help you out. Let's dive into the problem and find a solution together. 😊
The Problem 🤔
In the code you provided, you encountered the following error: "Uncaught TypeError: this.props.onSelectLanguage is not a function". This error occurs because the onSelectLanguage
prop is not defined or passed correctly to the child component.
The Solution 💡
To pass data from a child component to its parent in ReactJS, you can follow these steps:
Define a function in the parent component that will handle the data received from the child component. In your case, it's called
handleLanguageCode
.const ParentComponent = React.createClass({ // ... handleLanguageCode(langValue) { this.setState({ language: langValue }); }, // ... });
Pass the
handleLanguageCode
function as a prop to the child component.render() { return ( <div className="col-sm-9"> <SelectLanguage onSelectLanguage={this.handleLanguageCode} /> </div> ); }
Inside the child component, call the
onSelectLanguage
prop with the data you want to pass to the parent. Make sure to pass the data as an object to handle multiple values, like{ selectedLanguage: lang, selectedCode: code }
.handleLangChange(e) { const lang = this.state.selectedLanguage; const code = this.state.selectedCode; this.props.onSelectLanguage({ selectedLanguage: lang, selectedCode: code }); }
Finally, make sure the child component receives the
onSelectLanguage
prop correctly.render() { // ... <DropdownList // ... onChange={this.handleLangChange} /> // ... }
By following these steps, you should be able to pass data from the child component to its parent without encountering the "TypeError" error.
Handling JSON Data in the Child Component 📚
Now, let's address the second part of your question – handling JSON data in the child component. From the JSON example you provided, it looks like each language entry is an array with two values, such as ["aaa", "english"]
. To display both elements next to each other in the dropdown, you can modify the JSON structure slightly.
{
"languages": [
{
"code": "aaa",
"name": "english"
},
{
"code": "aab",
"name": "swedish"
}
// ...
]
}
Then, in your child component, you can use the map
function to extract both the code and the language name and display them together as an option in the dropdown.
render() {
const json = require("json!../languages.json");
const jsonArray = json.languages;
return (
<div>
<DropdownList
// ...
data={jsonArray.map((language) => `${language.code},${language.name}`)}
/>
</div>
);
}
By mapping each language entry in the JSON array, you can create options that show both the code and the language name as desired.
Wrapping Up 🎉
Congrats! You've learned how to pass data from a child component to its parent in ReactJS and how to handle JSON data in the child component. Feel free to apply these techniques in your own projects and share your success with others! 😄
If you have any more questions or need further assistance, don't hesitate to leave a comment below. Happy coding! 💻✨