How to fix missing dependency warning when using useEffect React Hook
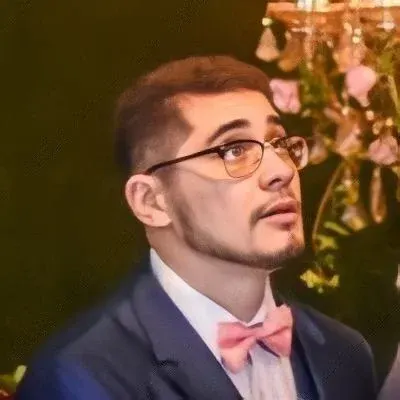
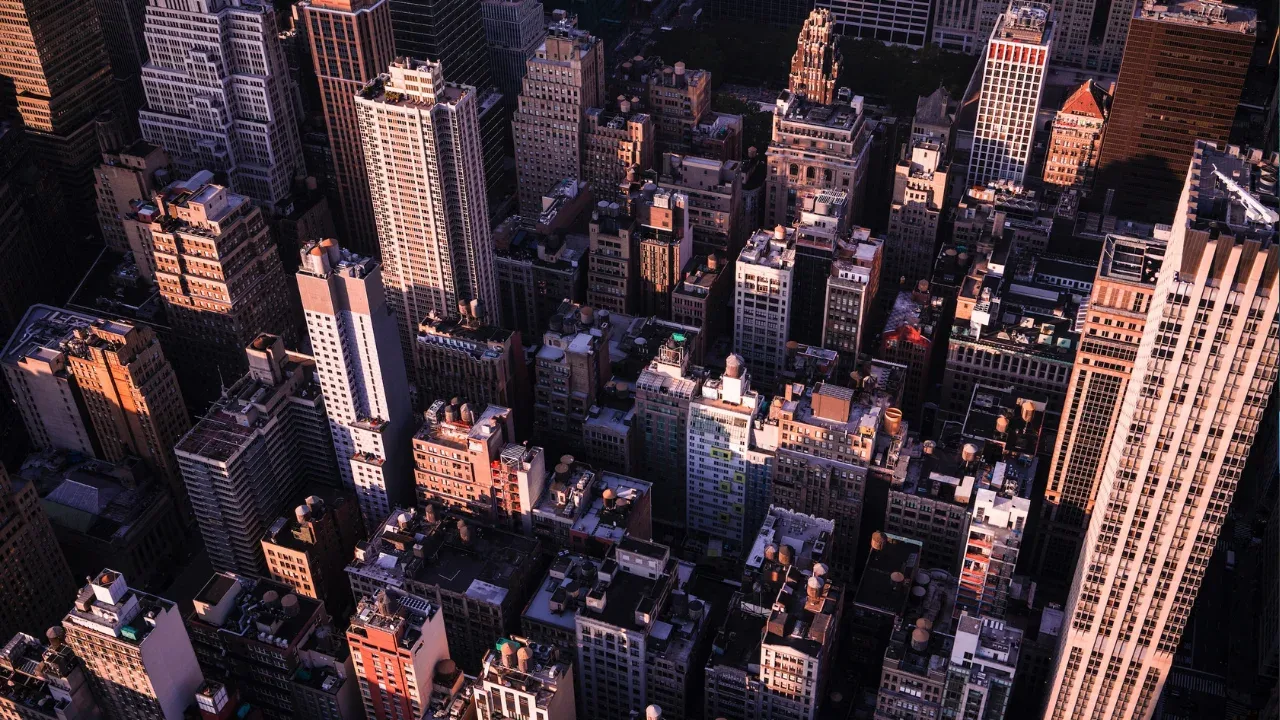
📝 [Tech Blog] How to Fix Missing Dependency Warning When Using useEffect React Hook
Are you stuck with a pesky missing dependency warning when using the useEffect React Hook? 🚫🔗 Don't worry, we've got you covered! In this blog post, we'll address common issues related to this warning, provide easy solutions, and help you say goodbye to those infinite loops once and for all! 🎉
The Issue at Hand 🤔
One frustrated developer reported encountering the following error message:
./src/components/BusinessesList.js
Line 51: React Hook useEffect has a missing dependency: 'fetchBusinesses'.
Either include it or remove the dependency array react-hooks/exhaustive-deps
This error often appears when trying to prevent an infinite loop on a fetch request. The user also expressed a desire to avoid using useReducer()
. After some research, they came across a suggested solution on a GitHub discussion thread, but it left them unsure about its implementation.
The Current Setup ⚙️
Currently, the user is utilizing the useEffect() hook like this:
useEffect(() => {
fetchBusinesses();
}, []);
Here, fetchBusinesses() is a function responsible for making the fetch request in order to retrieve businesses. However, the missing dependency warning emerged as a result of not including it in the dependency array.
The Easy Solution 💡
To fix the missing dependency warning, we need to include fetchBusinesses
as a dependency in the useEffect() hook. This ensures that the effect only runs when fetchBusinesses
changes.
useEffect(() => {
const fetchBusinesses = () => {
return fetch("theURL", { method: "GET" })
.then(res => normalizeResponseErrors(res))
.then(res => res.json())
.then(rcvdBusinesses => {
// handle received businesses
})
.catch(err => {
// handle errors
});
};
fetchBusinesses();
}, [fetchBusinesses]);
By adding fetchBusinesses
to the dependency array, we're specifying that the effect should re-run whenever fetchBusinesses
changes. This eliminates the missing dependency warning and prevents infinite loops.
Further Considerations 💡
While the above solution solves the immediate issue, it's important to mention another potential improvement. The user mentioned stumbling upon the useCallback()
function during their research.
useCallback()
is a React Hook that returns a memoized version of the callback function. It's useful in scenarios where the same callback is passed to child components, as it prevents unnecessary re-rendering. However, it's not required to resolve the missing dependency warning.
Conclusion and Next Steps 👏
Congratulations on fixing the missing dependency warning when using the useEffect React Hook! 🎉 Now you can run your fetch request without triggering infinite loops and enjoy smooth sailing in your React application.
If you want to dive deeper into the useCallback() function or explore other React Hooks, be sure to check out our related blog posts. And don't forget to share your success story with us! We'd love to hear how this solution worked for you. 💪💬
Keep coding, keep learning, and keep pushing the boundaries of your React applications! 🚀✨
Take Your Tech Career to the Next Level
Our application tracking tool helps you manage your job search effectively. Stay organized, track your progress, and land your dream tech job faster.
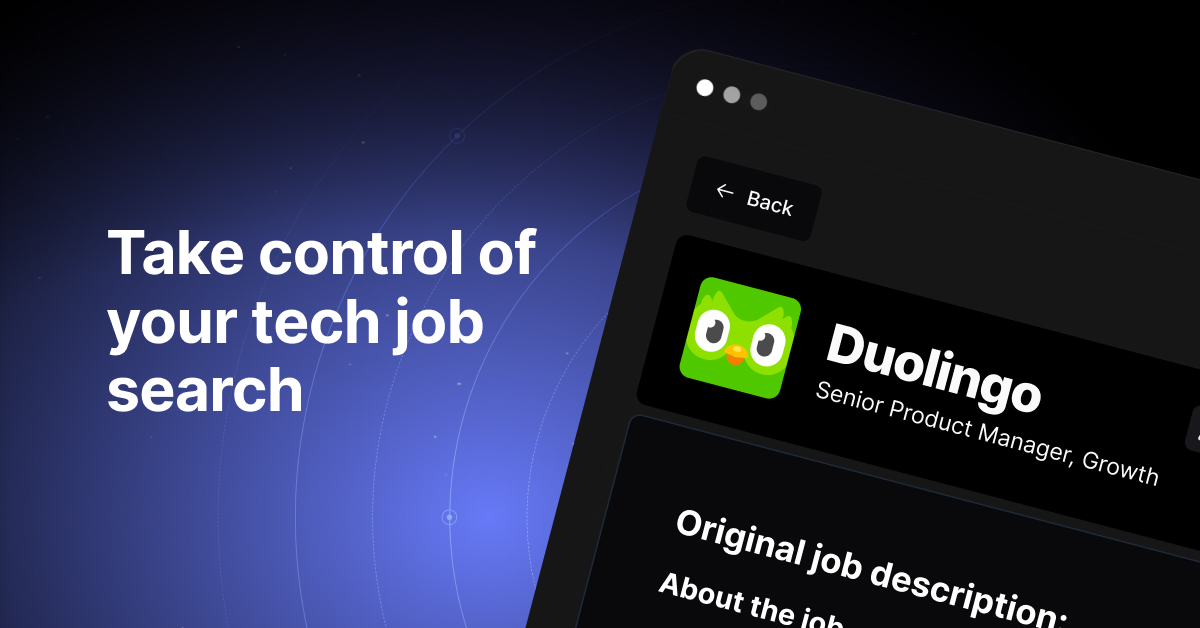