How to compare oldValues and newValues on React Hooks useEffect?
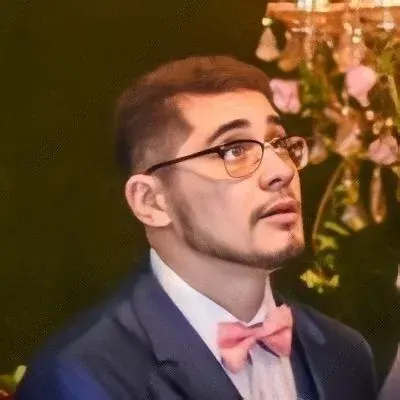
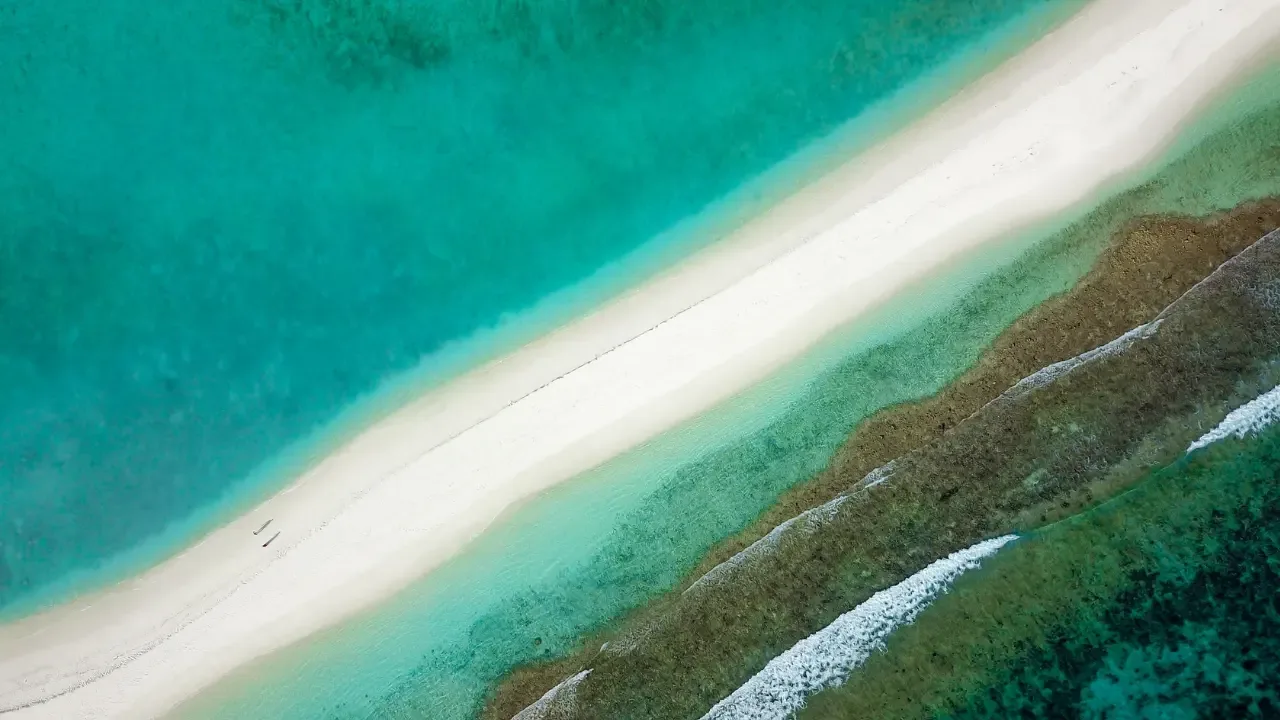
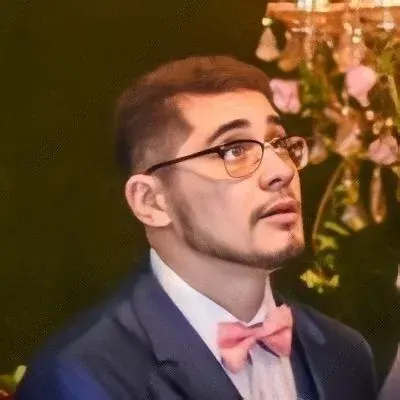
How to Compare oldValues and newValues on React Hooks useEffect?
š¤ Have you ever wondered if there's a way to compare oldValues
and newValues
in useEffect
on React Hooks, just like in the good old componentDidUpdate
days?
š Well, you're in luck! In this post, we'll explore an easy solution to this problem. We'll address the specific issue of comparing values in a useEffect
hook and provide a compelling call-to-action for you to engage with.
The Problem
Let's set the stage: imagine you have three inputs in your UI - rate
, sendAmount
, and receiveAmount
. You want to put these three inputs under the useEffect
hook to track any changes. Here are the rules to follow:
If
sendAmount
changes, calculatereceiveAmount = sendAmount * rate
.If
receiveAmount
changes, calculatesendAmount = receiveAmount / rate
.If
rate
changes and eithersendAmount > 0
orreceiveAmount > 0
, calculatereceiveAmount = sendAmount * rate
orsendAmount = receiveAmount / rate
, respectively.
š« Dealing with all these conditions in a single useEffect
hook seems challenging, right? But worry not, we've got you covered!
The Solution
To solve this problem, we'll introduce a custom hook called usePrevious
. This hook will allow us to keep track of the previous values of the inputs and compare them against the current values.
Here's a link to a helpful CodeSandbox that demonstrates the final solution.
How to Use usePrevious
Create a new file called
usePrevious.js
and add the following code:
import { useRef, useEffect } from 'react';
export default function usePrevious(value) {
const ref = useRef();
useEffect(() => {
ref.current = value;
});
return ref.current;
}
Import the
usePrevious
hook into your component:
import usePrevious from './usePrevious';
Inside your component, define the necessary state variables:
const [rate, setRate] = useState(0);
const [sendAmount, setSendAmount] = useState(0);
const [receiveAmount, setReceiveAmount] = useState(0);
Use the
usePrevious
hook to track the previous values of the state variables:
const prevRate = usePrevious(rate);
const prevSendAmount = usePrevious(sendAmount);
const prevReceiveAmount = usePrevious(receiveAmount);
Finally, inside the
useEffect
hook, compare the previous and current values to determine the necessary calculations:
useEffect(() => {
if (prevSendAmount !== sendAmount) {
const newReceiveAmount = sendAmount * rate;
setReceiveAmount(newReceiveAmount);
} else if (prevReceiveAmount !== receiveAmount) {
const newSendAmount = receiveAmount / rate;
setSendAmount(newSendAmount);
} else if (prevRate !== rate && (sendAmount > 0 || receiveAmount > 0)) {
const newReceiveAmount = sendAmount * rate;
const newSendAmount = receiveAmount / rate;
setReceiveAmount(newReceiveAmount);
setSendAmount(newSendAmount);
}
}, [prevSendAmount, prevReceiveAmount, prevRate, sendAmount, receiveAmount, rate]);
š And there you have it! With the help of the usePrevious
hook, you can now easily compare the oldValues
and newValues
in your useEffect
hook.
Conclusion
React Hooks make it easier than ever to manage state and side effects. However, comparing oldValues
and newValues
in a useEffect
hook can be a bit tricky. By using the usePrevious
hook, you can simplify this process and handle specific conditions effortlessly.
So, why not give it a try? Use the provided solution and see how it improves your code! And if you have any questions or doubts, feel free to leave a comment below. We'd love to help you out!
š Happy coding!