Hide keyboard in react-native
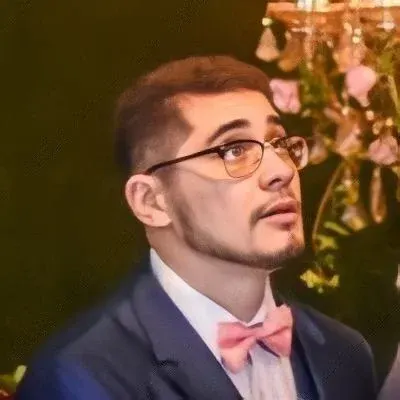
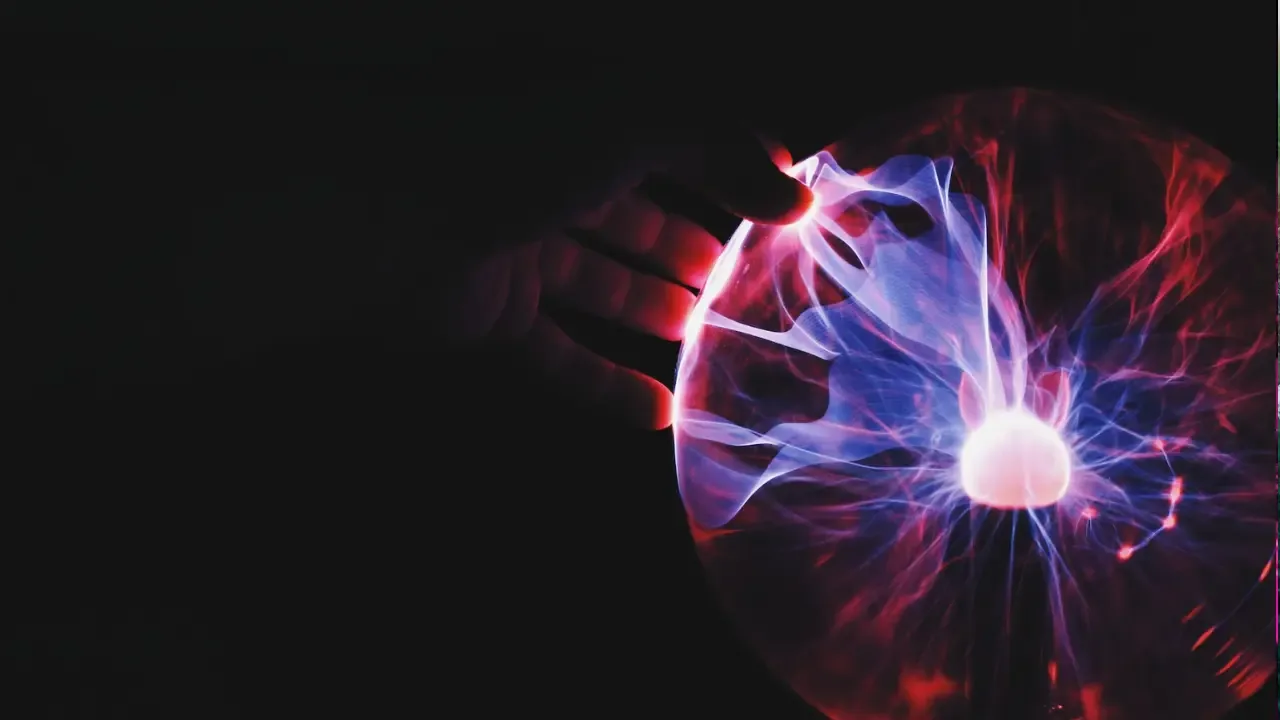
Hide Keyboard in React Native: A Simple Guide 📱
So you're building an awesome app with React Native, and one of the challenges you're facing is how to hide the keyboard when the user taps somewhere else on the screen. 🤔 Don't worry, we've got you covered! In this blog post, we'll address this common issue and provide easy solutions to help you out. Let's dive in! 💪
The Problem 🙇♂️
One of our readers was struggling with hiding the keyboard in React Native. Their specific requirement was to dismiss the keyboard when the user taps outside the text input, excluding the return key. Unfortunately, they couldn't find any useful information in the tutorials and blog posts they came across. 😔
Here's the code snippet they shared, just to give you some context:
<View style={styles.container}>
<Text style={styles.welcome}>
Welcome to React Native!
</Text>
<Text style={styles.instructions}>
To get started, edit index.ios.js
</Text>
<Text style={styles.instructions}>
Press Cmd+R to reload,{'\n'}
Cmd+D or shake for dev menu
</Text>
<TextInput
style={{height: 40, borderColor: 'gray', borderWidth: 1}}
onEndEditing={this.clearFocus}
/>
</View>
The Solution 🛠️
To fulfill the reader's requirement, we need to add a touchable area outside the text input that triggers the keyboard dismissal. Here's how to do it:
Import the
TouchableWithoutFeedback
component from React Native:
import { TouchableWithoutFeedback, Keyboard } from 'react-native';
Wrap the entire content inside the
TouchableWithoutFeedback
component, like this:
<TouchableWithoutFeedback onPress={Keyboard.dismiss} accessible={false}>
<View style={styles.container}>
{/* Your content goes here */}
</View>
</TouchableWithoutFeedback>
Done! Now, when the user taps anywhere outside the text input, the keyboard will be dismissed. 🎉
Example Implementation 🚀
Here's how you can integrate the solution into your code:
import React, { Component } from 'react';
import { View, Text, TextInput, TouchableWithoutFeedback, Keyboard } from 'react-native';
export default class App extends Component {
render() {
return (
<TouchableWithoutFeedback onPress={Keyboard.dismiss} accessible={false}>
<View style={styles.container}>
<Text style={styles.welcome}>
Welcome to React Native!
</Text>
<Text style={styles.instructions}>
To get started, edit index.ios.js
</Text>
<Text style={styles.instructions}>
Press Cmd+R to reload,{'\n'}
Cmd+D or shake for dev menu
</Text>
<TextInput
style={{ height: 40, borderColor: 'gray', borderWidth: 1 }}
onEndEditing={this.clearFocus}
/>
</View>
</TouchableWithoutFeedback>
);
}
}
const styles = {
container: {
flex: 1,
justifyContent: 'center',
alignItems: 'center',
backgroundColor: '#F5FCFF',
},
welcome: {
fontSize: 20,
textAlign: 'center',
margin: 10,
},
instructions: {
textAlign: 'center',
color: '#333333',
marginBottom: 5,
},
};
Conclusion 🎉
Hiding the keyboard when the user taps outside the text input is now a breeze, thanks to the TouchableWithoutFeedback
component and the Keyboard.dismiss
function provided by React Native. 🙌
We hope this guide helped you solve this common issue in your React Native app. If you have any questions, feel free to leave a comment below. Happy coding! 💻✨
Do you need more React Native tips and tricks? Stay tuned for our future blog posts where we'll discuss more helpful solutions. Don't forget to follow us for more amazing content! 📚🔥
Your Turn! 🚀
Have you encountered any other challenges while working with React Native? Share your experiences and let's find solutions together! 💪💡
Remember, sharing is caring! If you found this guide helpful, don't hesitate to share it with your developer friends who might be struggling with this issue too. Let's spread the knowledge! 🤝💙
Take Your Tech Career to the Next Level
Our application tracking tool helps you manage your job search effectively. Stay organized, track your progress, and land your dream tech job faster.
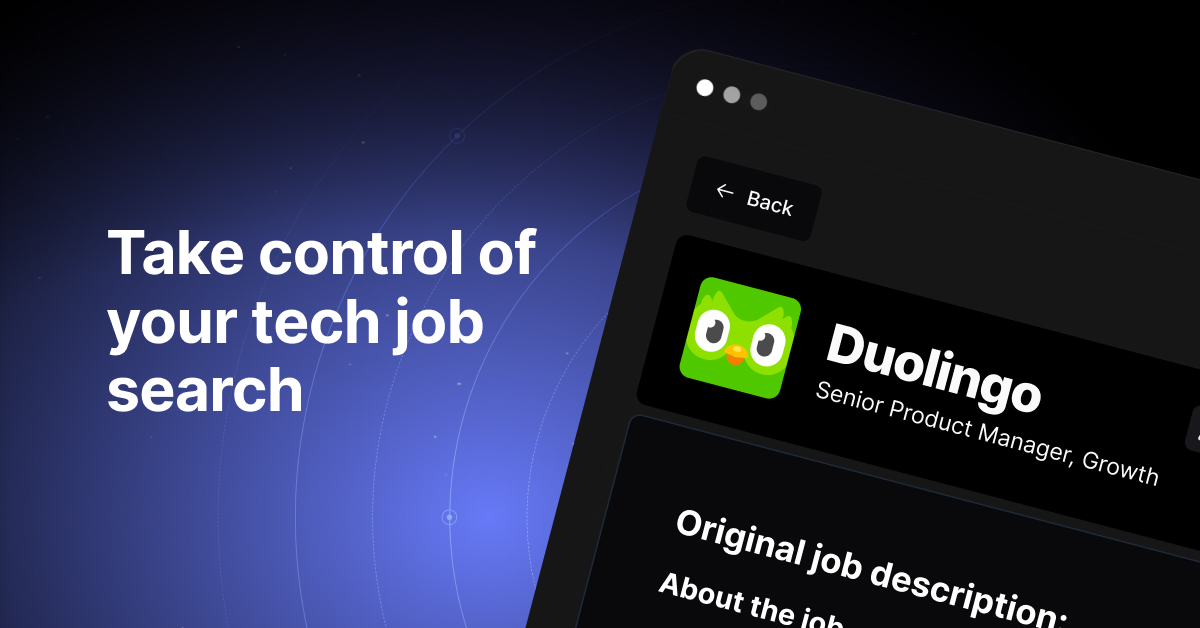