Having services in React application
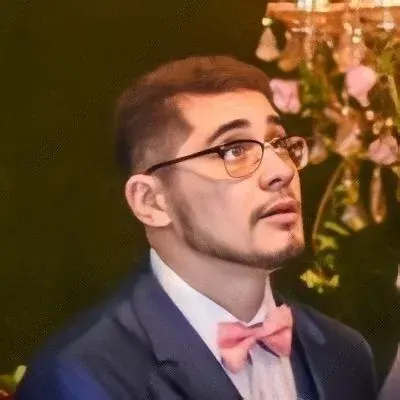
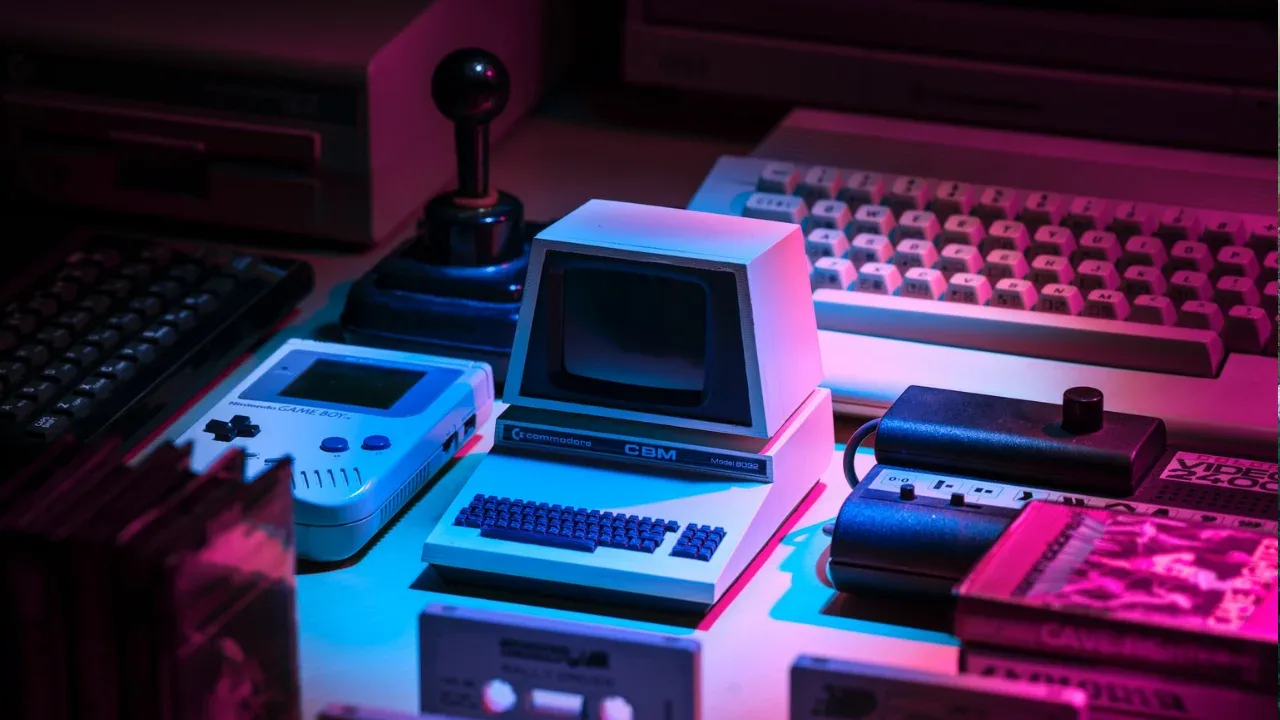
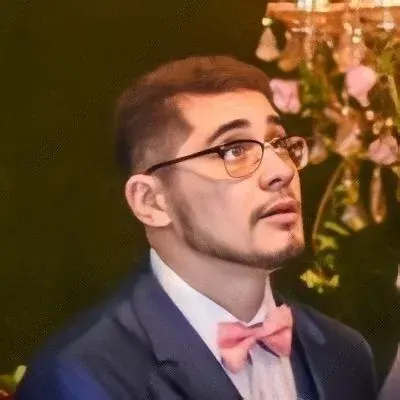
📝 How to Use Services in Your React Application
If you're familiar with Angular, you might be wondering how to extract logic to a service in your React application. In this blog post, we'll explore different options and provide easy solutions to achieve the same functionality. 🚀
💡 Understanding the Problem
Let's start by understanding the problem. Imagine you have a complex logic for validating a user's password strength in a React component. Writing this logic directly in the component can make it messy and difficult to maintain. Just like in Angular, you want to extract this logic and consume it elsewhere. But where should you write it in a React application? Let's find out! 😄
🎯 Solution 1: Using a Custom Hook
One popular way to extract logic in React is by using a custom hook. Custom hooks allow you to encapsulate reusable logic that can be shared across multiple components. Here's an example:
// usePasswordValidator.js
import { useState } from 'react';
const usePasswordValidator = () => {
const [isValid, setValidity] = useState(false);
const validatePassword = (password) => {
// Complex logic to validate password strength
setValidity(/* validity state based on logic */);
};
return [isValid, validatePassword];
};
export default usePasswordValidator;
Now, in your component, you can easily use this custom hook:
import React from 'react';
import usePasswordValidator from './usePasswordValidator';
const MyComponent = () => {
const [isValid, validatePassword] = usePasswordValidator();
const handlePasswordChange = (event) => {
const password = event.target.value;
validatePassword(password);
};
return (
<div>
<input type="password" onChange={handlePasswordChange} />
{isValid ? <p>Password is strong!</p> : <p>Password is weak.</p>}
</div>
);
};
export default MyComponent;
With this approach, you keep your component clean and reusable, while the complex password validation logic resides in a separate custom hook. 😎
🎯 Solution 2: Using a Third-Party Library
If you prefer not to reinvent the wheel, you can leverage third-party libraries that offer solutions for managing complex logic in React. One popular library is Redux, which uses the Flux architecture. Here's how you can achieve the same result using Redux:
Define an action creator to validate the password:
// passwordActions.js
export const validatePassword = (password) => {
// Complex logic to validate password strength
return {
type: 'VALIDATE_PASSWORD',
payload: /* validity state based on logic */,
};
};
Create a reducer to update the state based on the action:
// passwordReducer.js
const initialState = {
isValid: false,
};
const passwordReducer = (state = initialState, action) => {
switch (action.type) {
case 'VALIDATE_PASSWORD':
return {
...state,
isValid: action.payload,
};
default:
return state;
}
};
export default passwordReducer;
Connect your component to Redux and dispatch the action:
import React from 'react';
import { connect } from 'react-redux';
import { validatePassword } from './passwordActions';
const MyComponent = ({ isValid, validatePassword }) => {
const handlePasswordChange = (event) => {
const password = event.target.value;
validatePassword(password);
};
return (
<div>
<input type="password" onChange={handlePasswordChange} />
{isValid ? <p>Password is strong!</p> : <p>Password is weak.</p>}
</div>
);
};
const mapStateToProps = (state) => ({
isValid: state.isValid,
});
const mapDispatchToProps = {
validatePassword,
};
export default connect(mapStateToProps, mapDispatchToProps)(MyComponent);
Using Redux, you can centralize your app's state and separate logic from your components, just like Angular services. 😊
📣 Take Action: Share Your Thoughts!
Now that you know how to implement services in your React application, which method do you prefer? Have you tried any other approaches? Share your thoughts in the comments below! 👇
Remember, extracting logic to separate services or custom hooks enhances code reusability and maintainability. So don't hesitate to give it a try and level up your React development! 💪
Disclaimer: The examples provided in this blog post are for demonstration purposes only. Adjust the code to fit your specific needs and project structure.