Get form data in React
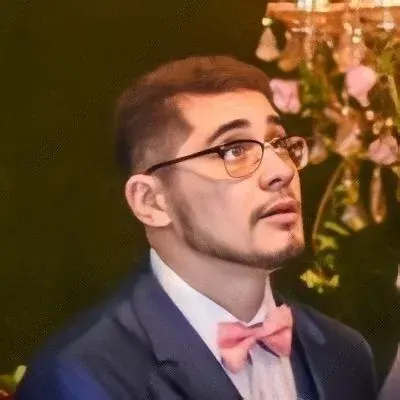
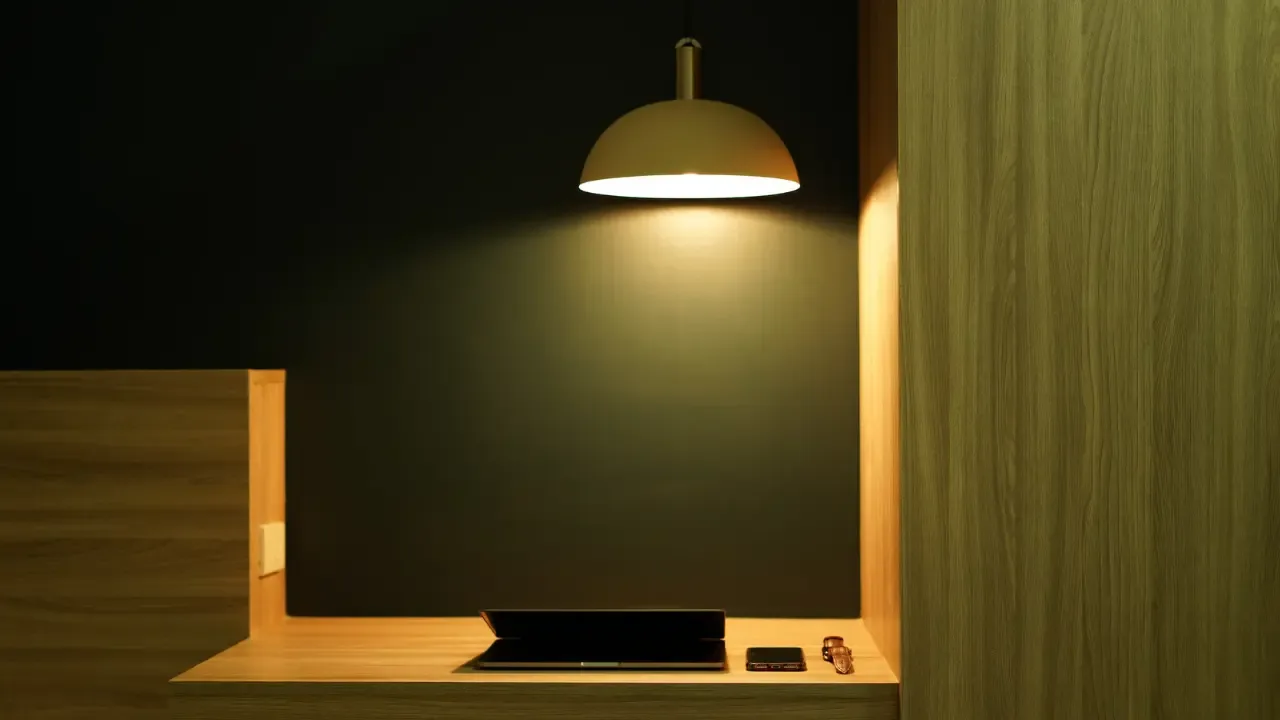
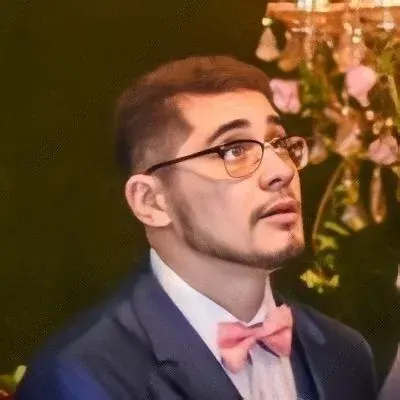
Get Form Data in React: A Simple Solution
So, you've built a form in your React app and now you're wondering how to access the data entered by the user in your handleLogin
function. 🤔 Don't worry, we've got you covered! In this blog post, we'll explore a simple solution to this common problem and help you get that data in no time. Let's dive in! 💪
Understanding the Problem
First, let's take a closer look at the code snippet you provided. In your render
function, you have a form with an email input, a password input, and a login button. You want to access the email and password entered by the user in your handleLogin
function.
The Solution
To solve this problem, we can leverage React's concept of controlled components. A controlled component is an input element whose value is controlled by React, rather than by the DOM. By managing the form data with React's state, we can easily access it in our handleLogin
function. Let's see how it's done.
In your component's state, define properties for email
and password
and initialize them with empty strings.
getInitialState: function() {
return {
email: '',
password: '',
};
},
Next, update your input elements by binding their values to the corresponding state properties and adding onChange
event handlers to update the state whenever the user enters or modifies data.
<input
type="text"
name="email"
placeholder="Email"
value={this.state.email}
onChange={this.handleInputChange}
/>
<input
type="password"
name="password"
placeholder="Password"
value={this.state.password}
onChange={this.handleInputChange}
/>
Now, let's implement the handleInputChange
function to update the state based on user input.
handleInputChange: function(event) {
const target = event.target;
const name = target.name;
const value = target.value;
this.setState({
[name]: value,
});
},
Great! Now, whenever the user enters or modifies data in the input fields, the state will be updated accordingly. 🙌
Accessing Form Data
Finally, let's tackle the main question: how can we access the email and password fields in our handleLogin
function? The answer is simple: we can directly access the values stored in the state.
handleLogin: function() {
const email = this.state.email;
const password = this.state.password;
// Do something with email and password...
},
With the form data stored in the state, you can now easily access it in your handleLogin
function and perform any necessary actions, such as making API calls or validating the data. 🚀
Conclusion
Congratulations! 🎉 You've successfully learned how to get form data in React by leveraging controlled components and managing component state. By following these steps, you can access user input in your event handlers and handle it according to your app's needs.
If you have any further questions or need additional assistance, don't hesitate to reach out in the comments section below. Happy coding! 💻
Share Your Experience!
Have you encountered any challenges while getting form data in React? How did you overcome them? Share your story and tips in the comments section! Let's learn from each other and make React development a breeze for everyone. 😊