Can I dispatch an action in reducer?
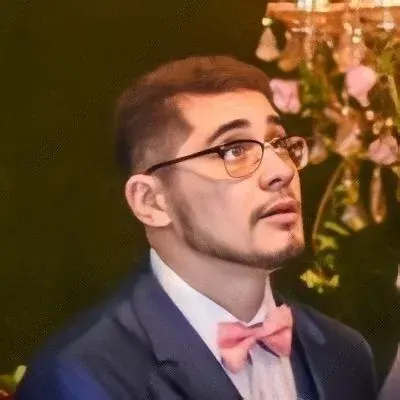
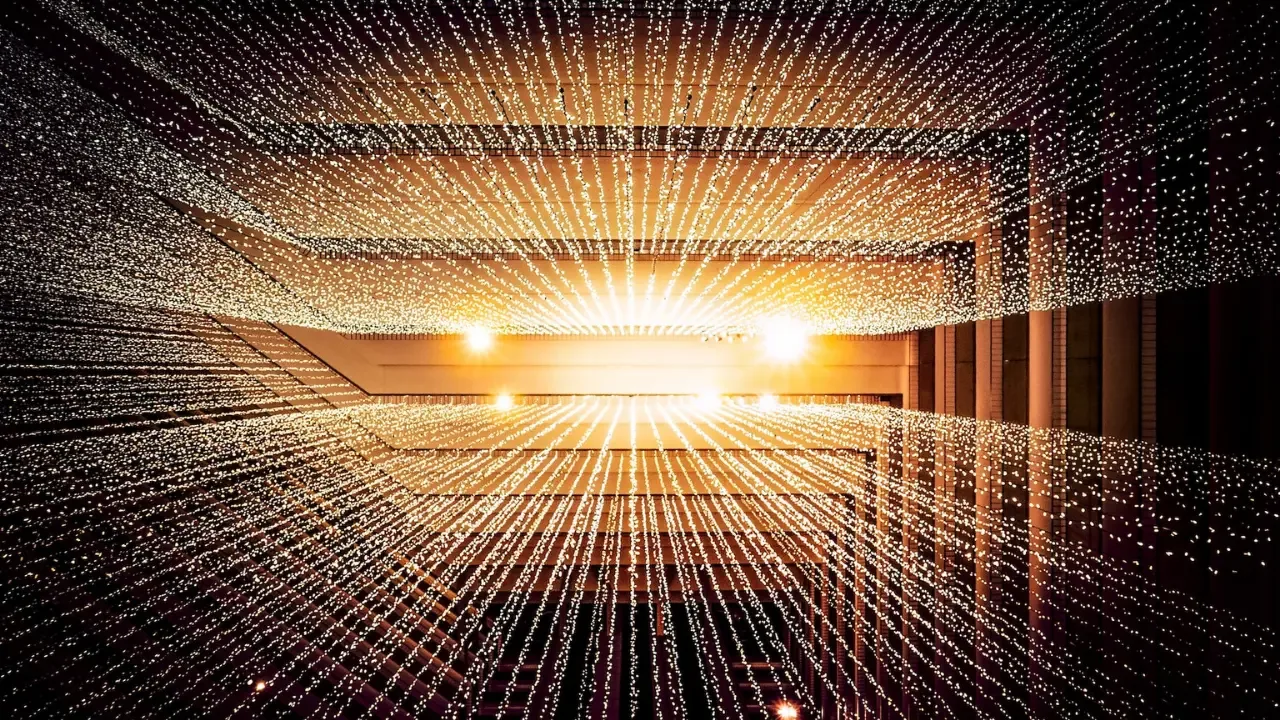
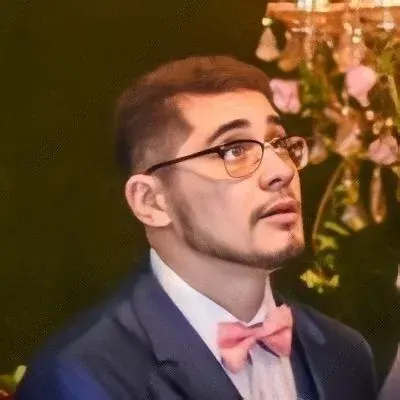
π₯πΆ Dispatching Actions in Reducer: A Complete Guide πΆπ₯
Do you have a burning desire to dispatch an action within a reducer? Need to update your progress bar dynamically while your audio element is playing? Well, you're in luck because we're about to dive deep into this topic! πββοΈπ¦
Understanding the Problem π€
Let's first understand the problem at hand. The goal here is to update the progress bar whenever the time is updated in the audio element. However, you might be scratching your head, wondering where to place your ontimeupdate
event handler or how to dispatch an action within its callback function. Fret not, my friend! We've got you covered! π―
The Solution: Middleware to the Rescue π¦ΈββοΈ
One approach to this problem is to leverage the power of middleware. Middleware is like a superhero that intercepts dispatched actions before they reach the reducer. By utilizing middleware, we can dispatch actions from event handlers or anywhere in our application. Pretty cool, huh? π
Step-by-Step Guide π
Ready to dispatch actions within your reducer? Follow these steps:
Step 1: Install Redux Thunk middleware
To use middleware, let's start by installing Redux Thunk, a popular middleware for Redux.
Open your terminal and run:
npm install redux-thunk
Step 2: Apply middleware in your store configuration
Next, go to your store configuration file and apply the Redux Thunk middleware. This step enables dispatching actions asynchronously within our application.
import { createStore, applyMiddleware } from 'redux';
import thunk from 'redux-thunk';
import rootReducer from './reducers';
const store = createStore(rootReducer, applyMiddleware(thunk));
export default store;
Step 3: Update your reducer
Now, let's update your reducer to dispatch the SET_PROGRESS_VALUE
action within the ontimeupdate
event handler. We'll use Redux Thunk to enable this dispatch.
import AudioElement from './AudioElement'; // Make sure you import AudioElement properly
const initialState = {
audioElement: new AudioElement('test.mp3'),
progress: 0.0,
};
const audio = (state = initialState, action) => {
switch (action.type) {
case 'SET_PROGRESS_VALUE':
return { ...state, progress: action.progress };
default:
return state;
}
};
export const updateProgressBar = () => (dispatch, getState) => {
const { audioElement } = getState().audio;
audioElement.audio.ontimeupdate = () => {
const progress = audioElement.currentTime / audioElement.duration;
dispatch({ type: 'SET_PROGRESS_VALUE', progress });
};
};
export default audio;
Step 4: Utilize the dispatched action
Now that we have set up the dispatch within the reducer, we need to utilize the dispatched action in our component. Here's an example of how you can do it:
import { useDispatch, useSelector } from 'react-redux';
import { updateProgressBar } from 'path/to/audioReducer';
const ProgressBar = () => {
const progress = useSelector((state) => state.audio.progress);
const dispatch = useDispatch();
React.useEffect(() => {
dispatch(updateProgressBar());
}, [dispatch]);
return <div>{progress}</div>;
};
Conclusion and Next Steps π
Congrats! π₯³ You've successfully dispatched an action within the reducer using Redux Thunk middleware. Now you can update your progress bar while your audio element is playing. How cool is that? Remember, middleware is a powerful tool, so feel free to explore other middleware options available in the Redux ecosystem.
If you found this guide helpful, share the knowledge with your fellow developers! Comment down below if you have any questions or thoughts. Keep coding and rock on! ππ»
Got any burning tech questions? Head over to our blog and leave a comment! Let's dive into the wonderful world of programming together! βοΈπ
Stay curious. Stay bold. Happy coding! ππ₯π