Attempted import error: "useHistory" is not exported from "react-router-dom"
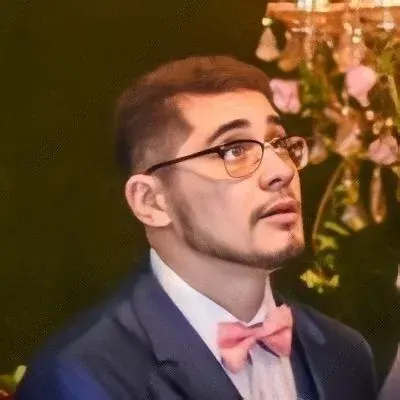
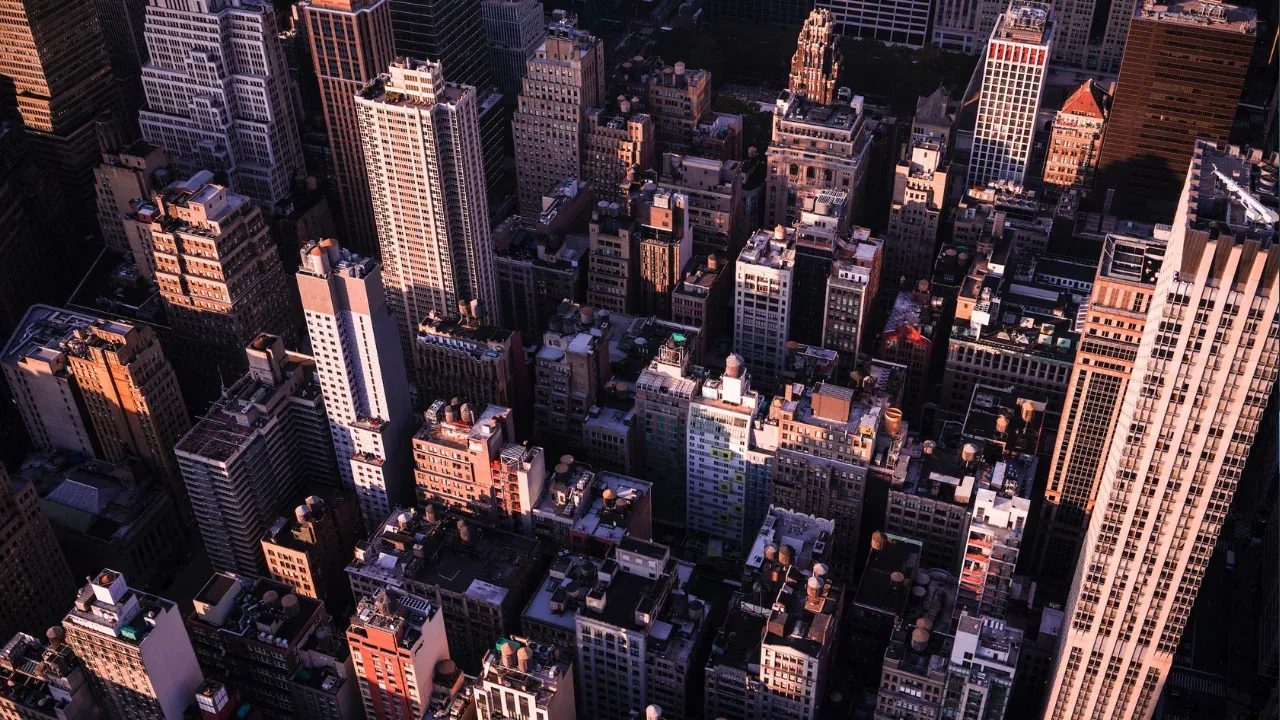
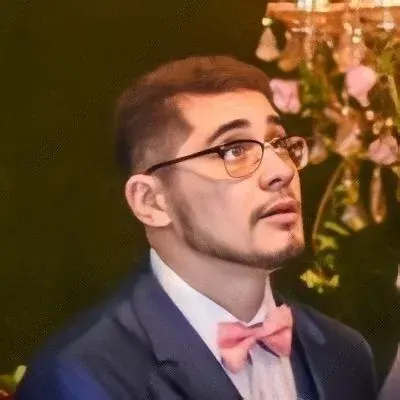
ππ€ Having trouble with 'useHistory' import error in react-router-dom? Here are some easy solutions! π
So, you're working on your πawesome React project and suddenly you encounter an error: Attempted import error: 'useHistory' is not exported from 'react-router-dom'
. π± Don't worry, we've got you covered! In this blog post, we'll dive into this common issue, provide easy solutions, and get you back on track. Let's get started! π₯
Understanding the Problem π΅οΈββοΈ
The error message is pretty straightforward. It's telling you that the 'useHistory' is not exported from 'react-router-dom'. But why is this happening? π€
The problem lies in the specific version of 'react-router-dom' you are using. In this case, you mentioned using version 4.3.1. π
Solutions π
1οΈβ£ Upgrade react-router-dom version: The 'useHistory' hook was introduced in a later version of 'react-router-dom'. To use it, you need to have a version equal to or newer than version 5.
If you want to upgrade your version, you can use the following command:
```
npm install react-router-dom@latest
```
After running this command, make sure to restart your development server for the changes to take effect.
2οΈβ£ Alternative solution: If you are not able or do not want to upgrade your 'react-router-dom' version, you can use a different hook called 'useNavigate'. This hook provides similar functionality to 'useHistory' and can be used as a replacement.
To use 'useNavigate', you can modify your import statement as follows:
```
import { useNavigate } from 'react-router-dom';
```
Then, replace `const history = useHistory();` with `const navigate = useNavigate();`.
Don't forget to refactor your code where 'history' was being used. Replace it with 'navigate' accordingly.
Example Solution π
Let's apply the alternative solution mentioned above to your code snippet:
import React, { useState } from 'react';
import { Grid } from '@material-ui/core';
import { useNavigate } from 'react-router-dom';
function UserForm() {
const [step, setStep] = useState(1);
const navigate = useNavigate();
const nextStep = (e) => {
e.preventDefault();
setStep(prevState => prevState + 1);
};
const previousStep = (e) => {
e.preventDefault();
setStep(prevState => prevState - 1);
};
const reGenerate = (e) => {
e.preventDefault();
};
const confirm = (e) => {
navigate('/');
};
return (
<div>
<h1>Hello</h1>
{/* The rest of your code */}
</div>
);
}
export default UserForm;
By making these changes, you should now be able to navigate between steps using 'useNavigate' instead of 'useHistory'. π
Conclusion and Call-to-Action πͺ
We hope this guide helped you overcome the 'useHistory' import error in 'react-router-dom'. Remember, if you're facing any other issues or have any questions, feel free to leave a comment below. π
Now, go ahead and implement the solution that works best for you! Happy coding! πβ¨