Attach Authorization header for all axios requests
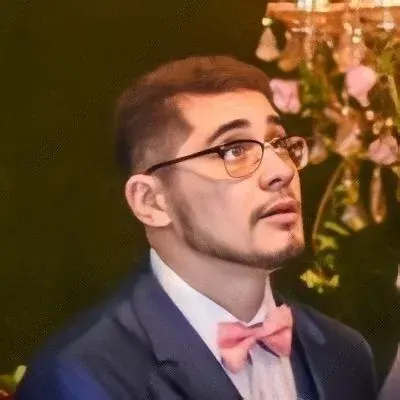
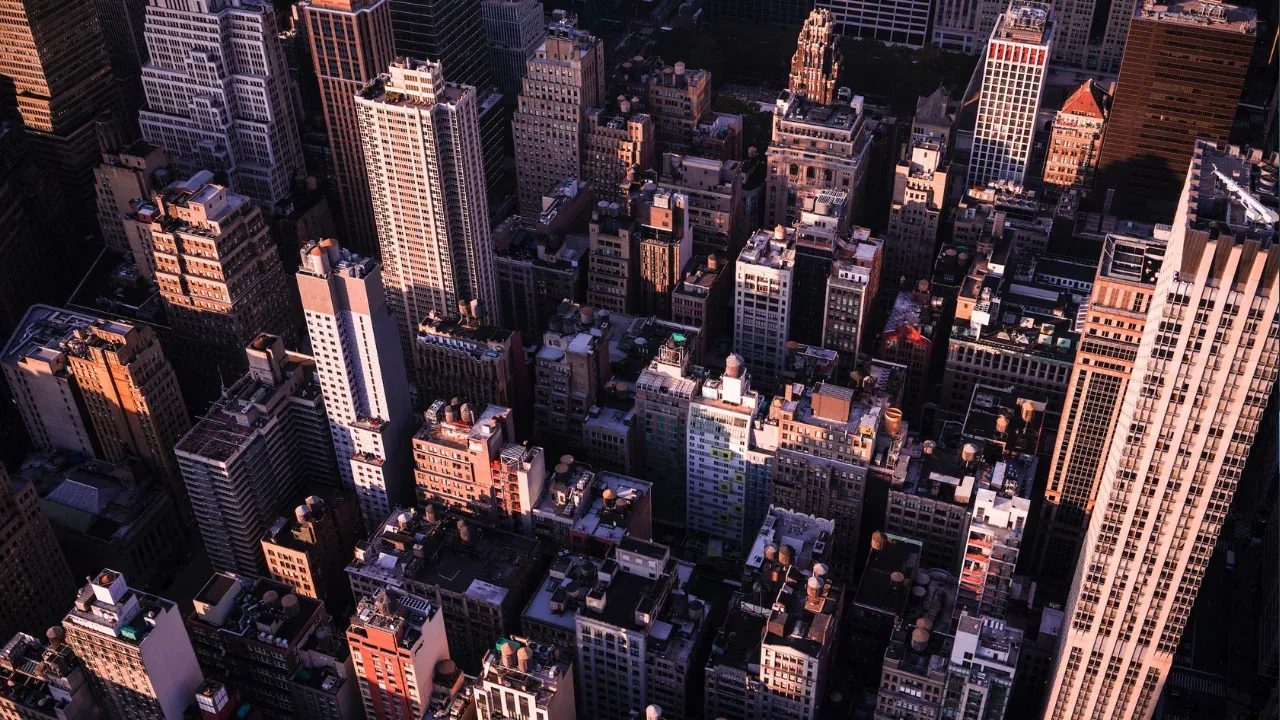
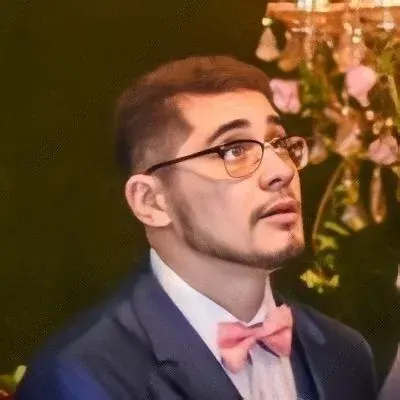
🚀 Easy 🚀 Axios Authorization Header for All Requests in React/Redux
So you want to attach an Authorization header to all Axios requests in your React/Redux application without manually adding it to each action? I got you covered! Let's dive into the best approach to solve this problem.
The Challenge
You're building a React/Redux application and after a user authenticates, you want to include an Authorization header in all Axios requests automatically. Adding the header manually to each request would be tedious and error-prone, not to mention inefficient.
The Solution
To automate the process of attaching the Authorization header, we'll create an Axios instance with default configuration options. This way, every request made with this instance will have the desired header. Here's how you can do it:
Import Axios and create an instance in your root directory, let's call it "api":
// api.js
import axios from 'axios';
const api = axios.create({
baseURL: 'https://api.mydomain.com',
});
export default api;
In your Redux actions, import the "api" instance you just created:
// actions.js
import api from './api';
export function loginUser(props) {
const url = '/login/';
const { email, password } = props;
const request = api.post(url, { email, password });
return {
type: LOGIN_USER,
payload: request
};
}
export function fetchPages() {
const url = '/pages/';
const request = api.get(url);
return {
type: FETCH_PAGES,
payload: request
};
}
Congratulations! 🎉 Now, every request made using the "api" instance will have the Authorization header attached automatically.
Bonus Tip: Attaching Token from Redux Store
To attach the token from your Redux store as the value for the Authorization header, you need to access it inside the Axios instance. One way to achieve this is by using Axios interceptors.
Modify the "api.js" file to include an interceptor:
// api.js
import axios from 'axios';
const api = axios.create({
baseURL: 'https://api.mydomain.com',
});
// Add an interceptor to modify requests
api.interceptors.request.use((config) => {
const token = store.getState().session.token;
if (token) {
config.headers.Authorization = `Bearer ${token}`;
}
return config;
}, (error) => {
return Promise.reject(error);
});
export default api;
Make sure to import the Redux store in the "api.js" file so you can access the token from the store. Let's assume your Redux store is stored in a file called "store.js":
// api.js
import axios from 'axios';
import store from './store';
// Rest of the code
That's it! Now, whenever a request is made with the "api" instance, the token from the Redux store will be automatically attached as the Authorization header.
Ready to Boost your Axios Requests?
You're all set now! 🚀 With this easy-to-implement solution, you can attach the Authorization header to all Axios requests effortlessly in your React/Redux application.
No more manual header attachments! Your code will be cleaner, more efficient, and less prone to errors.
If you have any feedback, ideas, or further questions, feel free to leave a comment below. Happy coding! 👩💻👨💻
P.S. Don't forget to share this awesome solution with your friends who may face similar challenges. Sharing is caring! 😊