Adding an .env file to a React project
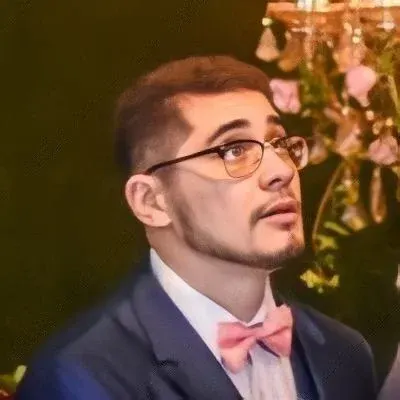
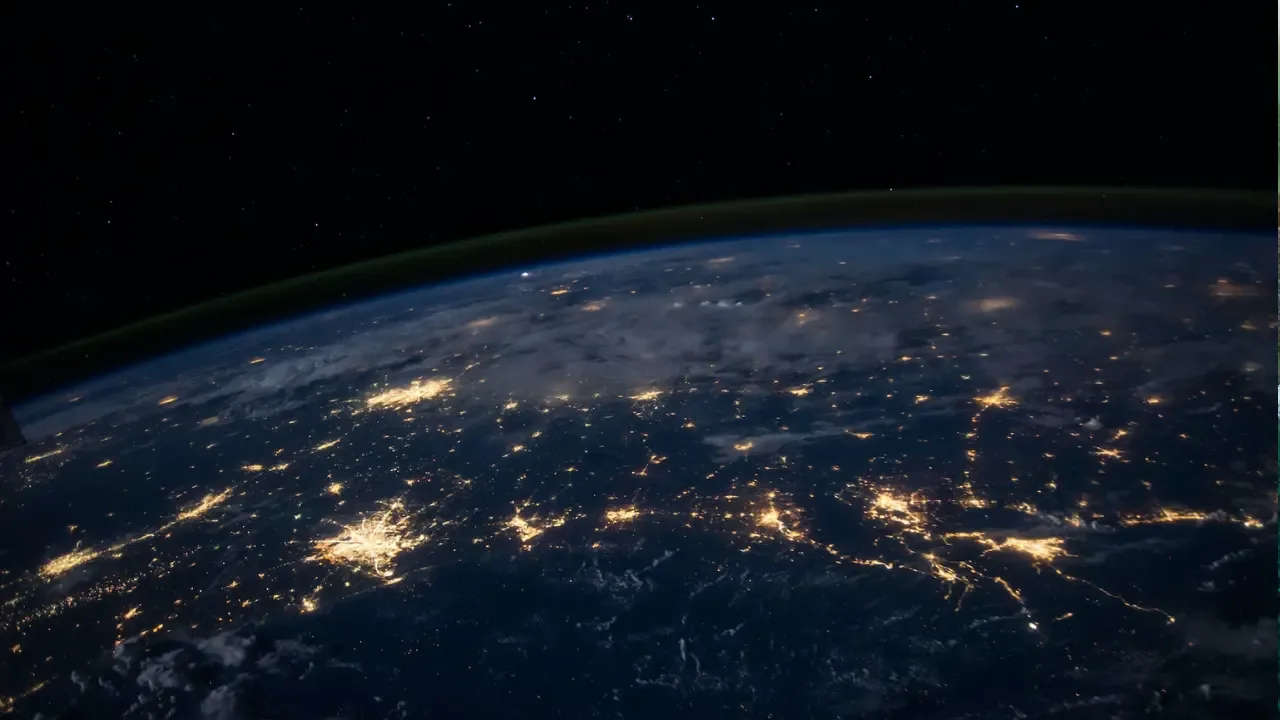
Adding an .env file to a React project: A Complete Guide đđ
Have you ever encountered the problem of wanting to hide your API key when committing your React project to GitHub? It can be a tricky situation, but fear not! We are here to guide you through it.
The Problem đ¤
One user on a forum had a similar issue and sought guidance on how to hide their API key in a Create React App. They followed the advice given in this Stack Overflow post, but unfortunately, their project failed to compile. Let's break down what might have gone wrong and find an easy solution.
Common Mistake: Misconfigured .env file đĨ
The user mentioned adding an .env
file to the root of their React project, but they named it process.env
. This is where the confusion arises. The correct name for the file is simply .env
without any prefixes or suffixes. It is essential to ensure that the file name is exactly .env
for React to recognize it.
Solution: Configuring the .env file âī¸
To resolve this issue and successfully hide your API key, let's follow these steps:
Create an
.env
file in the root directory of your React project.Open the
.env
file in your favorite code editor.Inside the
.env
file, add the following line:REACT_APP_API_KEY=my-secret-api-key
âšī¸ Make sure to replace
my-secret-api-key
with your actual API key.Save the changes to the
.env
file.
Next, let's update the fetch
request in your App.js
file to make use of the hidden API key.
performSearch = (query = 'germany') => {
fetch(`https://api.unsplash.com/search/photos?query=${query}&client_id=${process.env.REACT_APP_API_KEY}`)
.then(response => response.json())
.then(responseData => {
this.setState({
results: responseData.results,
loading: false
});
})
.catch(error => {
console.log('Error fetching and parsing data', error);
});
}
Make sure to replace REACT_APP_API_KEY
with process.env.REACT_APP_API_KEY
inside the fetch
request. By accessing the API key using process.env.REACT_APP_API_KEY
, React will automatically replace it with the value from your .env
file.
Congratulations! đ
You have successfully hidden your API key in your React project. Now you can commit your code to GitHub without worrying about exposing sensitive information.
If you encounter any issues or have further questions, please let us know in the comments section below. We are here to help you!
Don't forget to share this helpful guide with your fellow React developers who might be facing a similar problem. Spread the đ React love!
âĄī¸ Happy coding! đŠâđģđ¨âđģ
Take Your Tech Career to the Next Level
Our application tracking tool helps you manage your job search effectively. Stay organized, track your progress, and land your dream tech job faster.
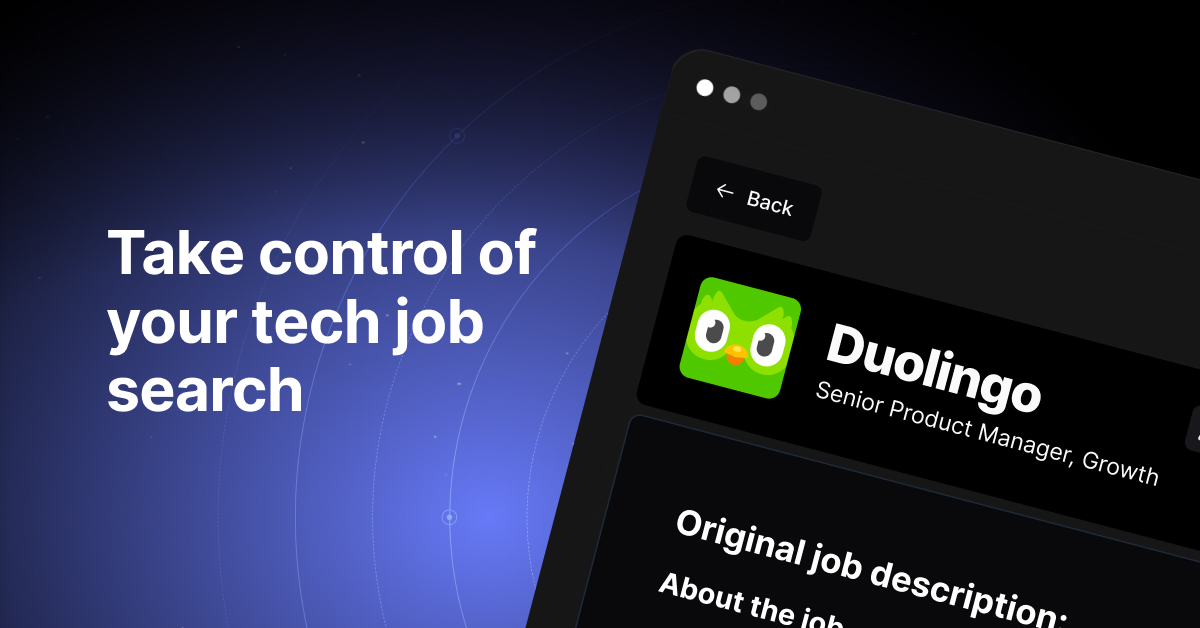