Why use purrr::map instead of lapply?
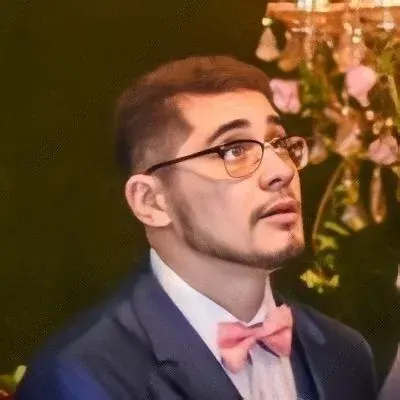
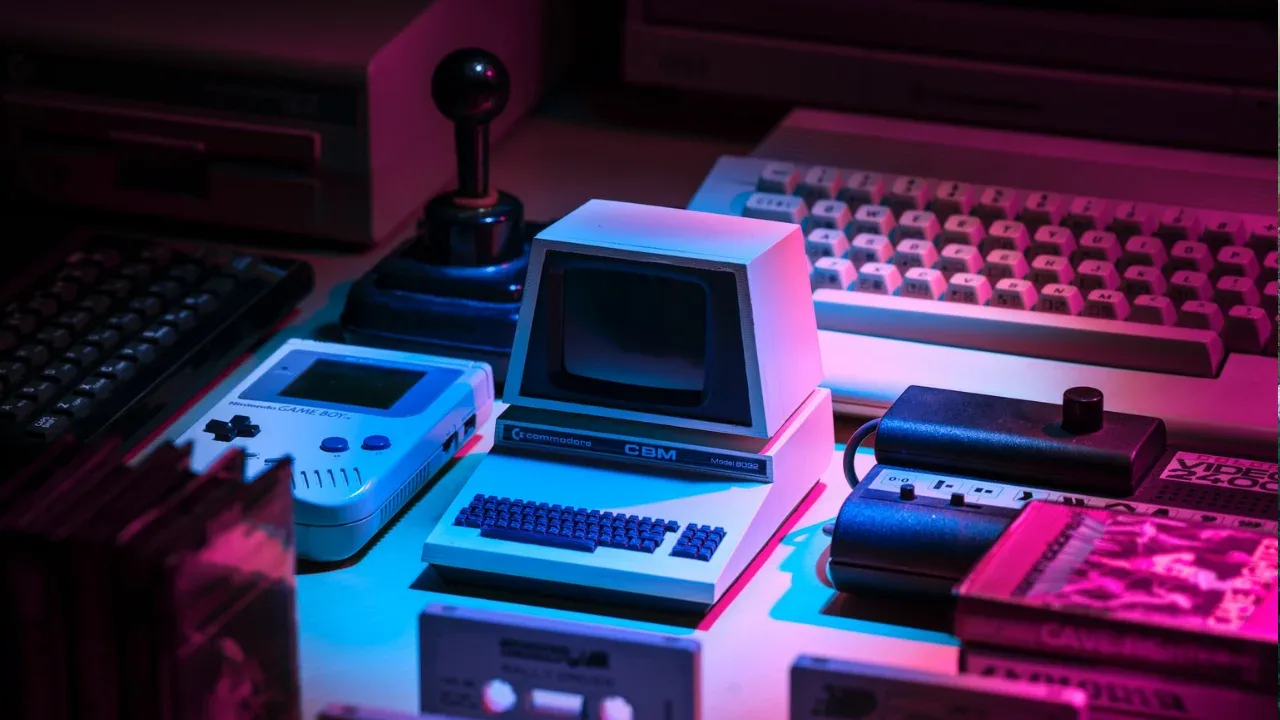
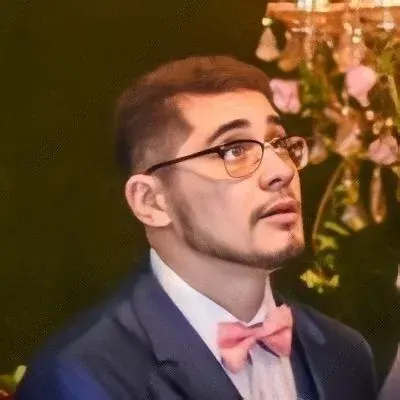
π The Power of purrr::map: A Game Changer in Functional Programming! π
Have you ever wondered about the difference between purrr::map
and lapply
? π If you're a fan of efficient code that's easy to read and maintain, then you're in the right place! π‘
πΊοΈ Understanding the Context
Let's start by exploring the context surrounding this burning question:
map(<list-like-object>, function(x) <do stuff>)
vs.
lapply(<list-like-object>, function(x) <do stuff>)
At first glance, it may seem like these two functions produce the same output, leaving you wondering why you should switch to purrr::map
. Additionally, benchmark tests might suggest that lapply
is slightly faster, which can make you skeptical. π€
However, let's delve deeper into this comparison and uncover the unique advantages of purrr::map
.
π The Power of Functional Programming
One of the cornerstones of purrr::map
lies in its ability to leverage the principles of functional programming. π―
π Consistent Output Dimensions
One major advantage of purrr::map
is its ability to return consistent output dimensions, even when faced with data complexities. For example, imagine you have a list-like object with both vectors and data frames as elements. purrr::map
will elegantly handle this, ensuring that the output remains consistent:
list_of_objs <- list(a = 1:3, b = data.frame(x = 1:3, y = 4:6))
map(list_of_objs, ~ as.character(.x))
By using map
, you'll get an output with matching dimensions, making further analysis and manipulation easier. π
π Simplifying Nested List Structures
Have you ever encountered deeply nested lists that require extensive loops and if conditionals? π« This is where purrr::map
truly shines! With its ability to traverse complex data structures, you can simplify your code using anonymous functions:
nested_list <- list(a = 1, b = list(c = 2, d = list(e = 3)))
map(nested_list, ~ if(is.list(.x)) modify_depth(.x, 2, log) else .x)
By utilizing modify_depth
within the anonymous function, you can modify the nested list structure only where needed, ensuring cleaner and more succinct code.
π₯ Igniting Performance and Exception Handling
Now, let's address performance factors and exceptional handling within the purrr::map
framework. β‘
β‘ Enhanced Performance with Future Evaluation
Although benchmark tests might suggest a slight advantage for lapply
, it's important to consider that purrr::map
utilizes future evaluation. This means it will evaluate all non-standard evaluation inputs as soon as possible, resulting in improved performance when working with large data sets. π
π§ Exception Handling Made Easy
purrr::map
also provides robust exception handling mechanisms, making it a reliable choice in uncertain scenarios. With the safely
function that comes bundled with purrr
, you can easily catch and handle exceptions. For example:
safe_division <- safely(function(x) 10 / x)
map(1:4, safe_division)
By utilizing safely
, you'll obtain a list containing both the result and an indication of whether the operation succeeded or failed. This way, you can effortlessly handle exceptions!
π Embrace the Power of purrr::map Today! π
In conclusion, while lapply
might offer similar outputs and slightly faster performance in some cases, purrr::map
introduces a wide range of advantages in functional programming. From handling nested lists effortlessly to improving performance with future evaluation and providing robust exception handling, purrr::map
empowers you to write clearer and more efficient code. πͺ
So, why not take the leap and embrace the power of purrr::map
in your next project? Start exploring its diverse functionalities and experience the joy of functional programming! π
Have you used purrr::map
before? How has it impacted your code? Share your thoughts and experiences in the comments below! π