Use dynamic name for new column/variable in `dplyr`
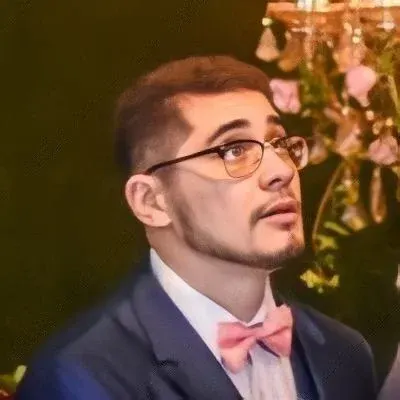
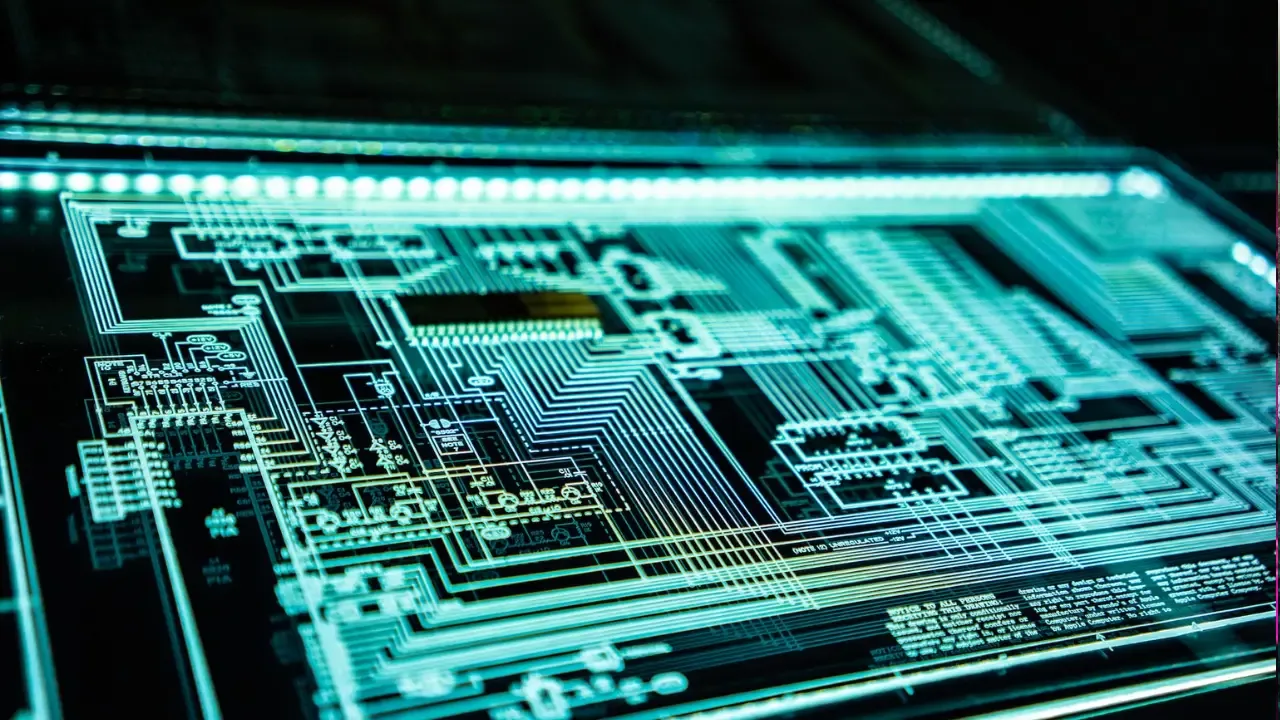
💡 Using Dynamic Names for New Columns in dplyr
Do you want to create multiple new columns in a data frame using the dplyr::mutate()
function with dynamically generated column names? If you're facing the issue of only getting one new variable instead of the desired multiple ones, fear not! We've got you covered with an easy solution.
💻 Let's take a look at an example using the iris
dataset:
library(dplyr)
iris <- as_tibble(iris)
📝 You've created a function named multipetal
to mutate new columns based on the Petal.Width
variable:
multipetal <- function(df, n) {
varname <- paste("petal", n, sep=".")
df <- mutate(df, varname = Petal.Width * n) ## problem arises here
df
}
🔄 Now, you want to loop through a range and create multiple new columns using this function:
for(i in 2:5) {
iris <- multipetal(df = iris, n = i)
}
🤔 However, when using mutate()
, it treats varname
as a literal variable name instead of dynamically generating the column names. As a result, only one new column called varname
is created instead of the desired four columns named petal.2
through petal.5
.
🔧 So, how can we get mutate()
to use dynamic names as column names? Here's the solution:
multipetal <- function(df, n) {
varname <- paste("petal", n, sep=".")
df <- mutate(df, !!varname := Petal.Width * n) ## solved!
df
}
By using the !!
operator before varname
, we tell mutate()
to interpret it as an evaluated variable instead of a literal name. This allows for the dynamic generation of column names.
🚀 With this updated code, you can run the loop again:
for(i in 2:5) {
iris <- multipetal(df = iris, n = i)
}
✨ Now, you will have four new columns named petal.2
through petal.5
in your iris
dataframe, with their contents calculated based on the Petal.Width
variable.
📣 Don't let the limitations of literal variable names hold you back from dynamically creating new columns! Try out this solution and unlock the full potential of dplyr::mutate()
.
What are some other tricks or challenges you've encountered with dplyr
? Share your experiences and solutions in the comments below! Let's keep the conversation going. 💬👇
Take Your Tech Career to the Next Level
Our application tracking tool helps you manage your job search effectively. Stay organized, track your progress, and land your dream tech job faster.
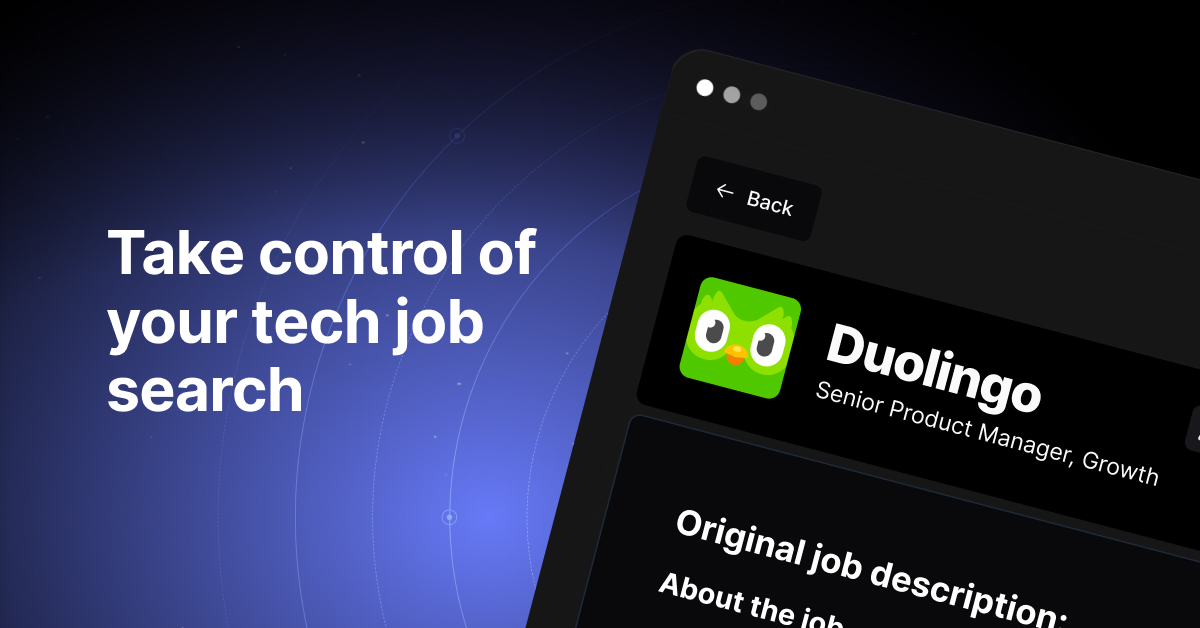