Understanding exactly when a data.table is a reference to (vs a copy of) another data.table
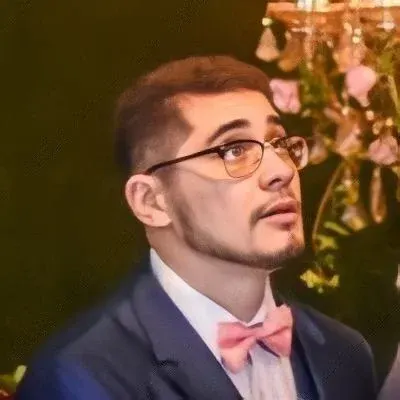

Understanding Pass-by-Reference in data.table: A Guide for Beginners 😎
So you're scratching your head trying to understand the whole pass-by-reference thing in data.table, huh? Don't worry, you're not alone! Many data.table users have faced the same confusion. 🤔
Let's dive in and unravel the mystery of when a data.table is a reference to another data.table and when it's a copy. We'll address common issues, provide easy solutions, and make sure you leave here with a clear understanding. 🚀
Exploring the Mystery 🕵️♀️
We'll start by looking at some code examples to demonstrate what's happening under the hood. Let's consider the following snippet:
library(data.table)
DT <- data.table(a=c(1,2), b=c(11,12))
print(DT)
# a b
# [1,] 1 11
# [2,] 2 12
newDT <- DT # reference, not copy
newDT[1, a := 100] # modify newDT
print(DT) # DT is modified too.
# a b
# [1,] 100 11
# [2,] 2 12
In this case, assigning DT
to newDT
creates a reference to the original data.table. So any modifications made to newDT
are reflected in DT
as well. This behavior is in line with what we expect from pass-by-reference. 😊
The Broken Reference Issue ☠️
But wait, things get interesting when we insert a non-:=
based modification between the assignment (DT <- data.table(...)
) and the :=
lines. Let's take a look:
DT = data.table(a=c(1,2), b=c(11,12))
newDT <- DT
newDT$b[2] <- 200 # new operation
newDT[1, a := 100]
print(DT)
# a b
# [1,] 1 11
# [2,] 2 12
In this scenario, the line newDT$b[2] <- 200
somehow "breaks" the reference, and DT
is no longer modified. 😱 What's going on here?
Understanding the Behavior 🧠
The reason newDT$b[2] <- 200
breaks the reference is that it triggers a copy of the data.table. When you perform an operation that requires modifying just a subset of the data, R automatically creates a copy to avoid unintentional changes to the original. This copy breaks the reference between newDT
and DT
.
The copy-on-modify behavior is R's way of protecting the integrity of your data. It ensures that any changes you make are explicit and don't unintentionally affect other variables. However, this behavior might not always be desired, especially when dealing with large datasets or memory constraints.
Workarounds and Solutions 💡
If you want to avoid breaking the reference and maintain pass-by-reference behavior, here are a couple of solutions:
Use the
set()
function: Theset()
function in data.table allows you to modify a data.table by reference. Here's an example:
DT <- data.table(a = c(1, 2), b = c(11, 12))
print(DT)
set(DT, i = 1, j = "a", value = 100)
print(DT)
# a b
# [1,] 100 11
# [2,] 2 12
With set()
, the modification happens directly on the data.table, preserving the reference.
Use the
copy()
function: If you want a copy of the data.table instead of a reference, you can explicitly create one using thecopy()
function. Here's an example:
DT <- data.table(a = c(1, 2), b = c(11, 12))
print(DT)
newDT <- copy(DT)
newDT[1, a := 100]
print(DT) # DT remains unchanged
# a b
# [1,] 1 11
# [2,] 2 12
By using copy()
, you create a separate copy of the data.table, ensuring that modifications made to the copy do not affect the original.
Understanding is Everything 👓
Understanding pass-by-reference in data.table is crucial to avoiding bugs and unexpected behavior in your code. By grasping the concepts discussed in this guide, you can confidently manipulate data.tables without worrying about unintentional modifications.
If you still have any doubts or want to explore more, feel free to connect with the awesome data.table community on Stack Overflow or data.table's official documentation. They'll be more than happy to assist you! 🌟
Now it's your turn! Share your experiences and let us know what other tips and tricks you have discovered 📢. Together, we can master data.table and make our data manipulations a breeze! 💪
Keep coding and stay curious! 🤓
Take Your Tech Career to the Next Level
Our application tracking tool helps you manage your job search effectively. Stay organized, track your progress, and land your dream tech job faster.
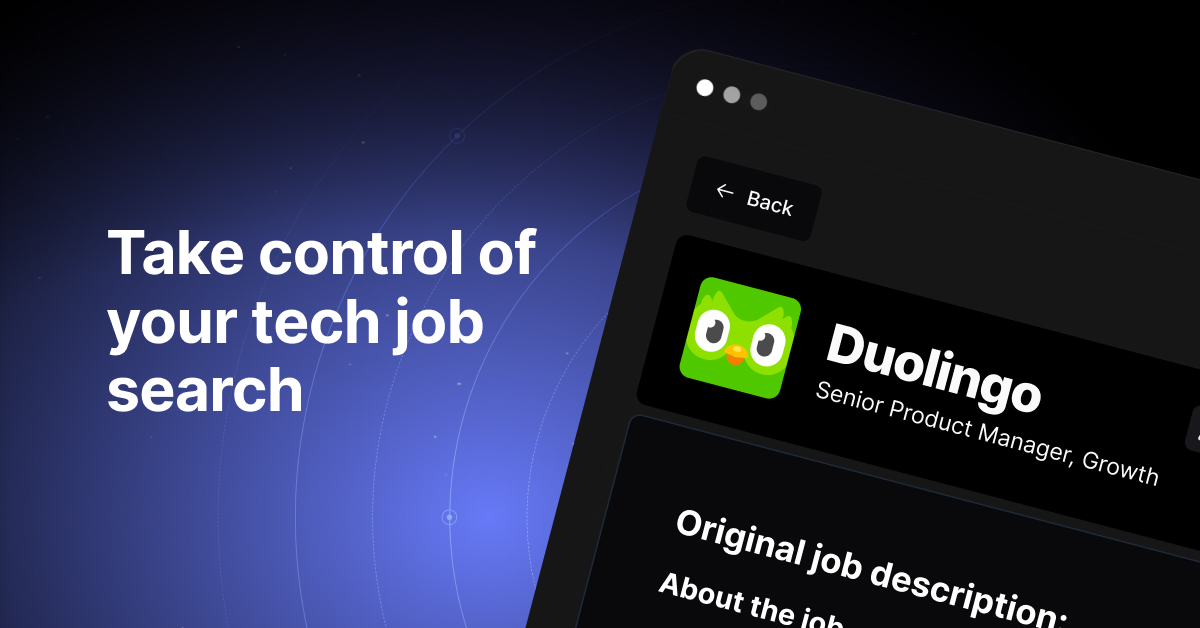