Test if a vector contains a given element
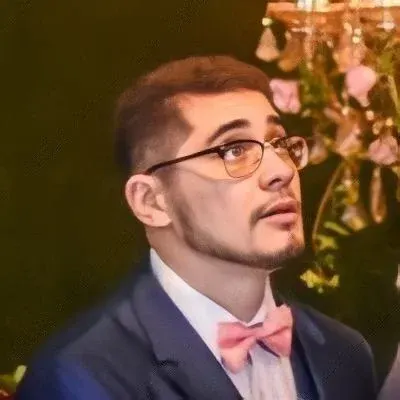
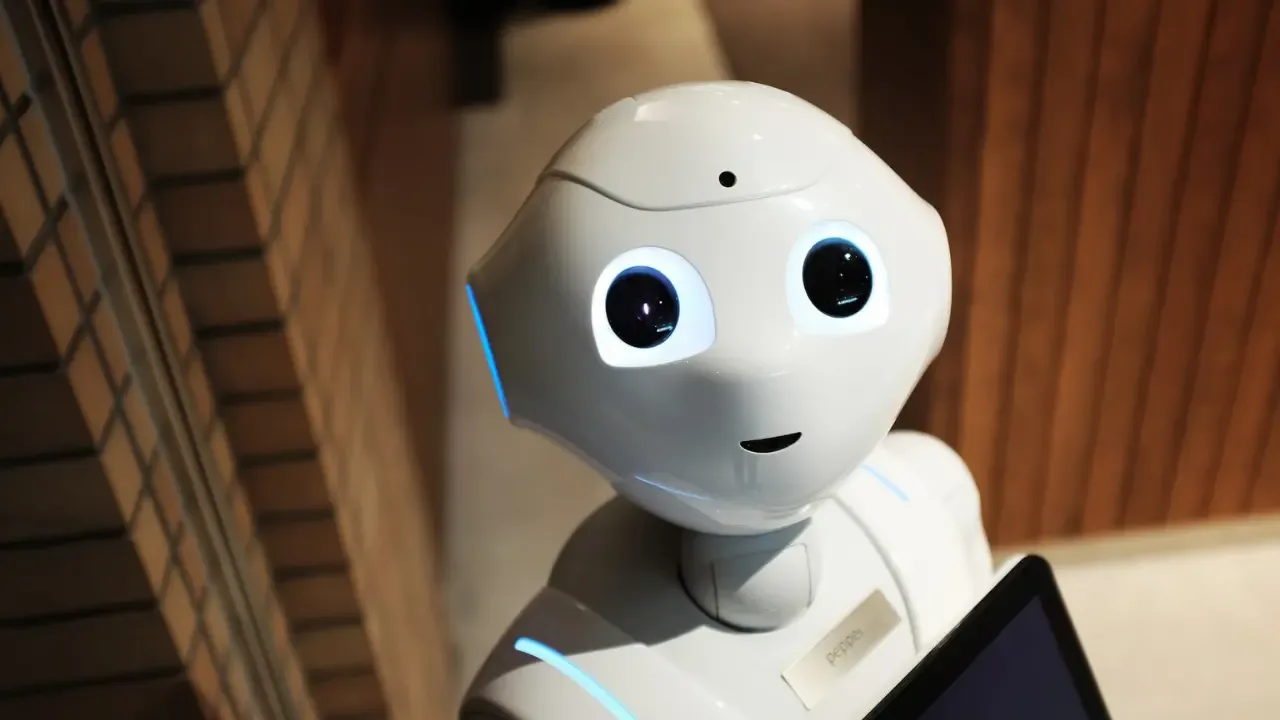
🧐 Is the Needle Hiding in the Haystack? Let's Find Out!
So, you've got a vector and you want to know if it contains a specific element. 🤔 Don't worry, we've got your back! We know how frustrating it can be to search for that elusive needle in the haystack. But fear not, because we have some super easy solutions to help you out!
🕵️ The Case of the Missing Element
Let's start by understanding the problem at hand. 📚 Imagine you have a vector – let's call it haystack
– and you want to check if it contains a certain value, which we'll call the needle
. The question is simple: is the needle
hiding somewhere within the haystack
?
⚙️ The Standard Approach
The most straightforward solution is to loop through the haystack
and compare each element with the needle
. 😅 This can be done easily with a for loop:
for (const element of haystack) {
if (element === needle) {
// Found it!
return true;
}
}
// Nope, the element is not here. 😢
return false;
In this example, we use a for...of loop to iterate over each element in the haystack
. We then compare each element with the needle
using the equality operator (===
). If we find a match, jackpot! We can return true
to indicate that the haystack
does contain the needle
. If we finish looping through the entire haystack
without finding a match, the needle
is not present, and we return false
.
🚀 Turbocharged Solution with Array.prototype.includes()
But wait, there's an even cooler solution! 😎 If you're using JavaScript, you can take advantage of the includes()
method provided by the Array
class. This method checks if an array (or in this case, a vector) includes a specific element, and it returns either true
or false
.
Here's how you can use includes()
to test if a vector contains a given element:
const containsNeedle = haystack.includes(needle);
if (containsNeedle) {
// We found it! 🎉
return true;
} else {
// Nope, not here. 😕
return false;
}
With just one line of code, haystack.includes(needle)
does all the work for you. It returns true
if the needle
is found in the haystack
, and false
otherwise. It's like having a magical search function 🧙♂️ at your fingertips!
📢 Your Turn to Engage!
Now that you've learned a couple of quick and simple ways to test if a vector contains a given element, it's your turn to try it out! 🤩 Experiment with these solutions in your own code and see the magic happen. Share your thoughts and experiences with us in the comments below! We'd love to hear from you. 👇
Happy coding! 💻✨
Take Your Tech Career to the Next Level
Our application tracking tool helps you manage your job search effectively. Stay organized, track your progress, and land your dream tech job faster.
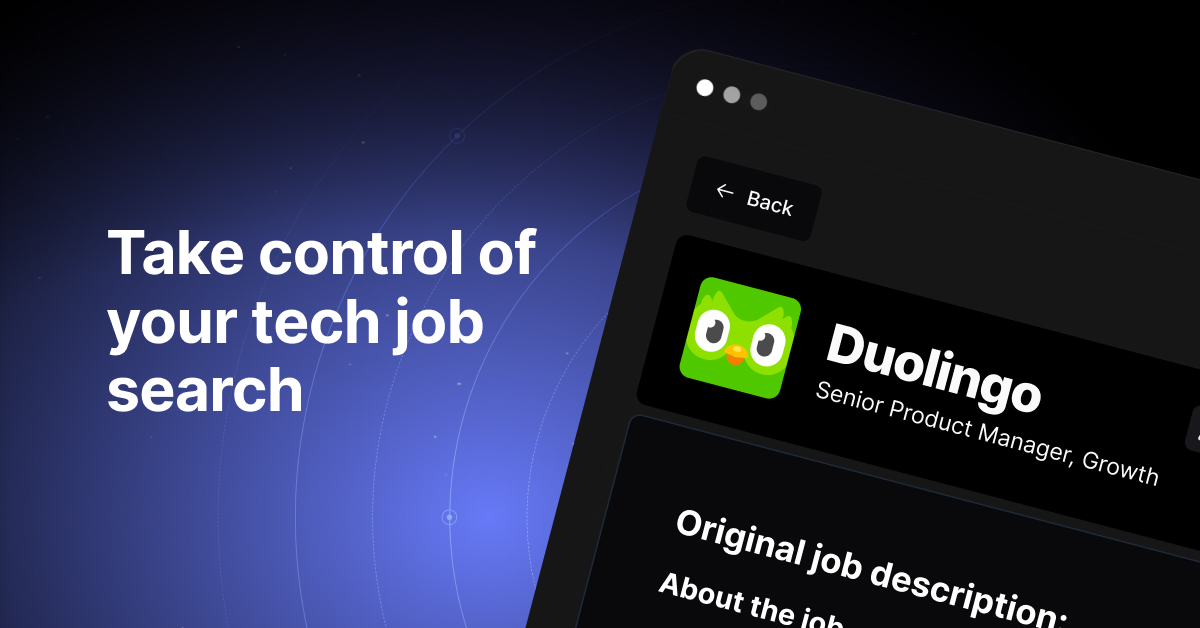