Standardize data columns in R
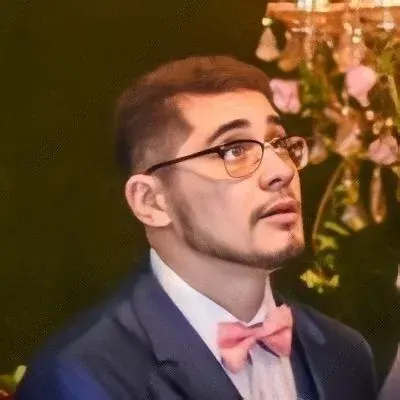
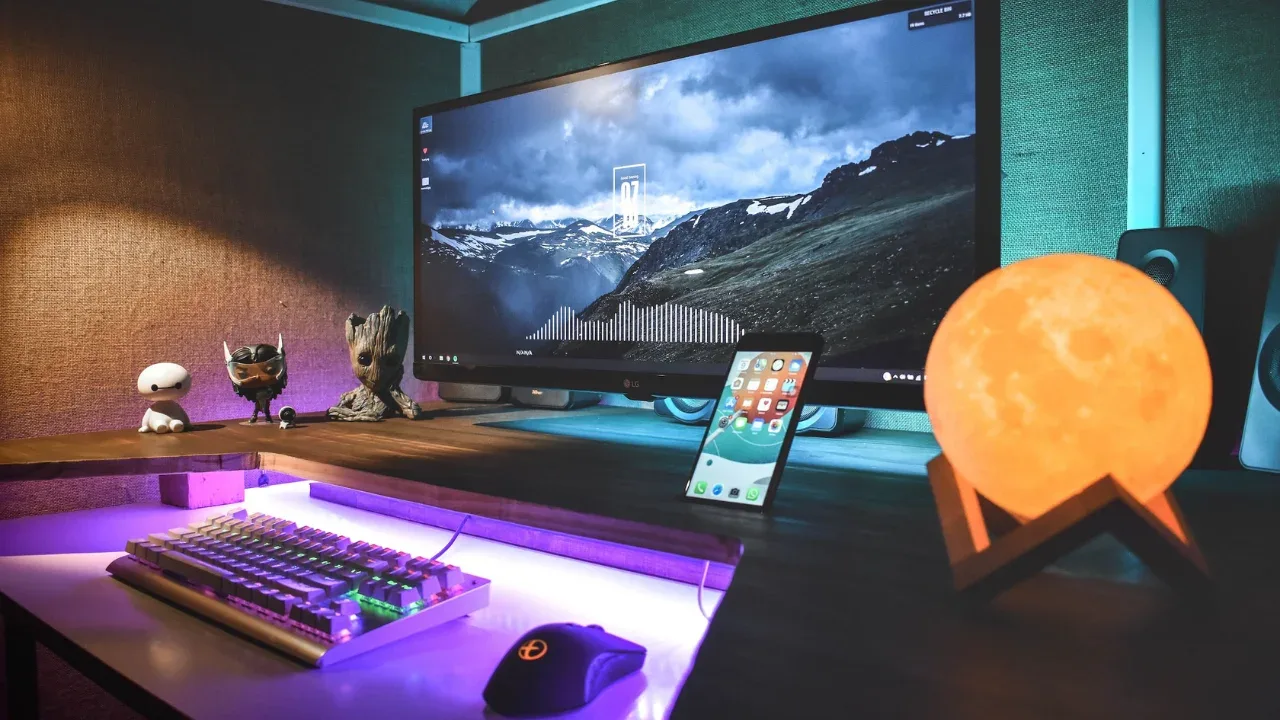
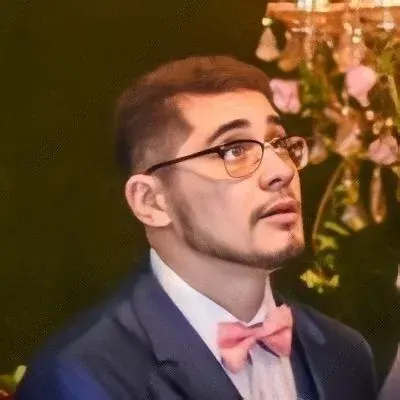
Standardize data columns in R: A Complete Guide 📊
So, you have a dataset called spam
with 58 columns and about 3500 rows of data related to spam messages. You want to perform some pre-processing and standardize the columns to have zero mean and unit variance before running linear regression. Smart move! 🧠
But you're not sure how to achieve this using R. Don't worry, I got you covered! In this guide, I'll walk you through the process of normalizing your data columns step by step. Let's get started! 🚀
1. Load the necessary packages 📦
Before we dive into the actual normalization process, let's make sure we have the required packages installed and loaded. In this case, we'll be using the dplyr
and caret
packages. If you don't have them yet, install them by running the following command:
install.packages(c("dplyr", "caret"))
Once installed, load the packages using the library()
function:
library(dplyr)
library(caret)
2. Pre-processing: Check for missing values 🔍
Before normalizing the data, it's always a good idea to check if there are any missing values in your dataset. Missing values can affect the accuracy of your normalization process. Use the following code to check for missing values:
# Assuming your dataset is stored in a variable called 'spam'
missing_values <- sum(is.na(spam))
missing_values
If the missing_values
variable is greater than 0, it means you have missing values to deal with. You can either remove those rows or impute the missing values with appropriate techniques. But that's a topic for another blog post! 😉
3. Normalize your data columns 📏
To standardize your data columns, we'll use the preProcess()
function from the caret
package. This function automatically performs various pre-processing steps, including normalization, on your dataset. Here's how you can do it:
# Assuming your dataset is stored in a variable called 'spam'
preprocessed_data <- preProcess(spam, method = c("center", "scale"))
# Apply the pre-processing transformation to your dataset
normalized_data <- predict(preprocessed_data, spam)
After executing these lines, you'll have a new dataset called normalized_data
, which contains the standardized columns. Each column will now have a mean of zero and a standard deviation of one.
4. Verify the transformation ✅
To make sure the transformation worked as expected, you can check the mean and standard deviation of each column in the normalized_data
dataset. Use the following code:
# Assuming your normalized dataset is stored in a variable called 'normalized_data'
column_stats <- data.frame(
Column = colnames(normalized_data),
Mean = colMeans(normalized_data),
Standard_Deviation = sqrt(colVars(normalized_data))
)
column_stats
Inspecting the column_stats
dataframe will give you a summary of the mean and standard deviation for each column. Ideally, you should see means close to zero and standard deviations close to one. If that's the case, congratulations, you have successfully standardized your data columns! 🎉
5. Engage with the community 🤝
I hope this guide helped you understand how to standardize data columns in R efficiently. But learning shouldn't stop here! Engaging with the R community can open doors to new insights and learning opportunities. Here are a few ways you can get involved:
Join R-related online forums and communities like Stack Overflow or RStudio Community. Ask questions, share your knowledge, and learn from others.
Follow prominent R bloggers and experts on platforms like Twitter or Medium. Their articles and insights can keep you updated on the latest trends and practices in the R ecosystem.
Contribute to open-source R projects on platforms such as GitHub. Collaborating with others will not only enhance your coding skills but also contribute to the growth of the R community.
Remember, learning is a journey, and the R community is here to support and guide you along the way! 🌟
I hope you found this guide helpful! Happy coding in R, and may your data analysis be as smooth as butter! 🧈💻
Is there anything else you'd like to learn about R or data analysis? Let me know in the comments below! 👇
Disclaimer: The example dataset and code snippets used in this guide are for illustrative purposes only. Make sure to adapt them to your specific dataset and requirements.
*[R]: R-Language *[API]: Application Programming Interface *[HTML]: HyperText Markup Language *[CSS]: Cascading Style Sheets