Select rows from one data.frame that are not present in a second data.frame
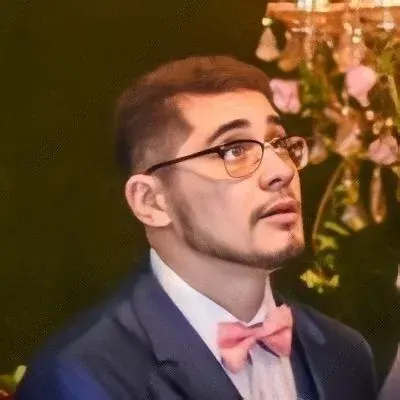
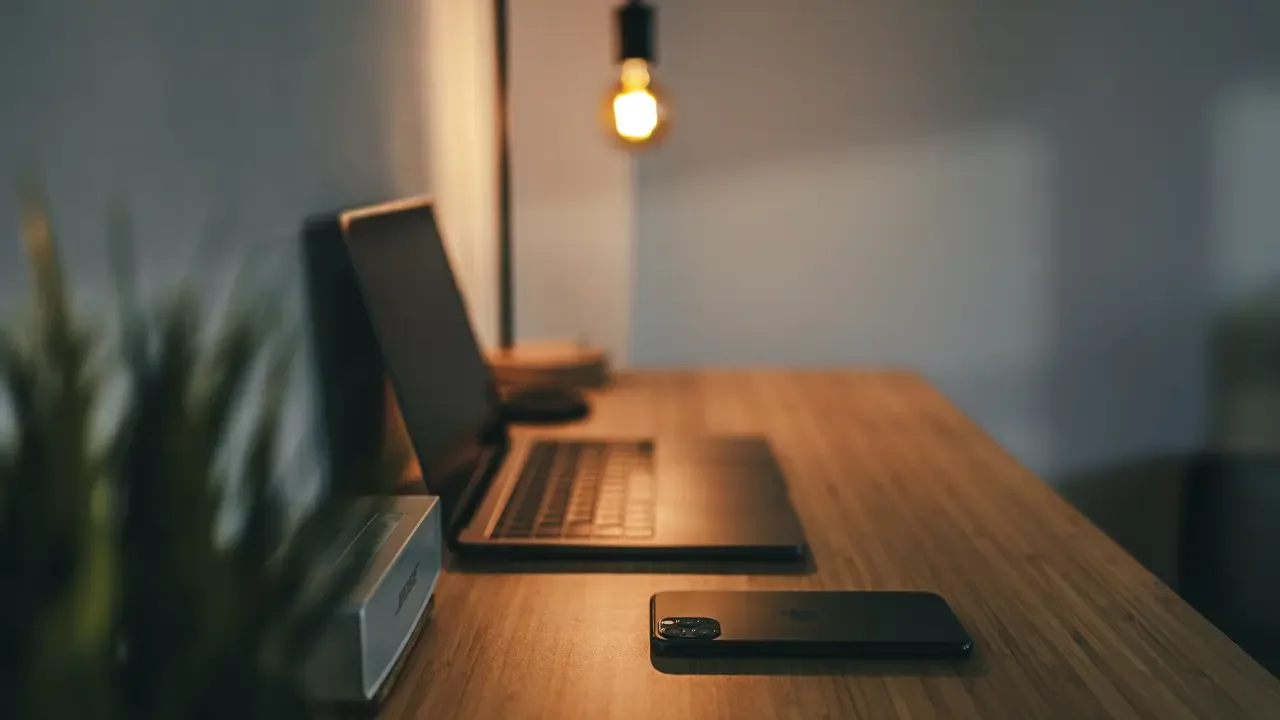
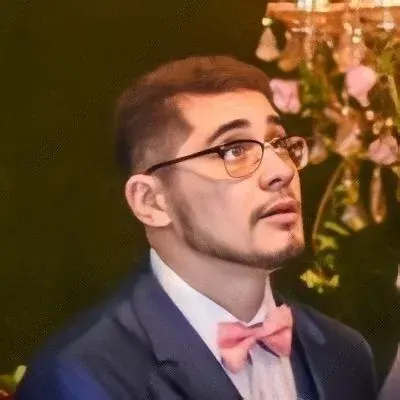
📝 Easily Select Rows from One Data.frame Not Present in Another 📝
Are you stuck with the problem of selecting rows from one data.frame that are not present in another? Don't worry, we've got you covered! In this blog post, we will address this common issue, provide easy solutions, and even share a more crafted code to help you out. So let's dive in! 💪
The Problem 🤔
Imagine you have two data.frames, a1
and a2
:
a1 <- data.frame(a = 1:5, b = letters[1:5])
a2 <- data.frame(a = 1:3, b = letters[1:3])
You want to find the rows in a1
that are not present in a2
. Basically, you're looking for a simple way to compare two data.frames and filter out the rows that don't match.
The Solution 💡
Now, the question arises: Is there a built-in function for this type of operation? While there isn't a direct function, we can use R's powerful capabilities to solve this problem easily.
Here's a simple and efficient solution using a custom function:
rows.in.a1.that.are.not.in.a2 <- function(a1, a2) {
a1.vec <- apply(a1, 1, paste, collapse = "")
a2.vec <- apply(a2, 1, paste, collapse = "")
a1.without.a2.rows <- a1[!a1.vec %in% a2.vec, ]
return(a1.without.a2.rows)
}
Simply call the function rows.in.a1.that.are.not.in.a2
and pass your data.frames a1
and a2
as arguments. The function will return the desired rows from a1
that are not present in a2
. 👌
rows.in.a1.that.are.not.in.a2(a1, a2)
A More Crafted Code in Action ✨
While the solution we provided above is simple and effective, you mentioned being curious if someone had already crafted a more refined code. So here's an alternative implementation that achieves the same result:
library(dplyr)
a1.without.a2.rows <- anti_join(a1, a2, by = c("a", "b"))
The anti_join
function from the popular dplyr
package does the trick here. It performs a left join on the common columns between a1
and a2
and returns only the rows from a1
that have no match in a2
.
Engage with Us! 👇
We hope this blog post helped you tackle the problem of selecting rows from one data.frame that are not present in another. Now, it's your turn to engage with us!
🔸 Have you encountered any other data.frame comparison challenges? Let us know in the comments below! 👇
🔸 Do you have a different approach or a more refined code for solving this problem? Share it with us! 🙌
Remember, together we can unlock the full potential of R in data manipulation and analysis! Keep exploring and stay tuned for more exciting tech tips and tricks. 🚀
Happy coding! 😊