Reshaping data.frame from wide to long format
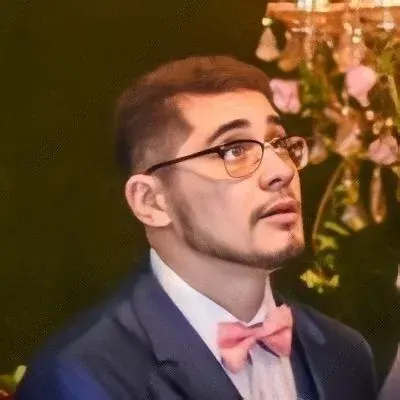
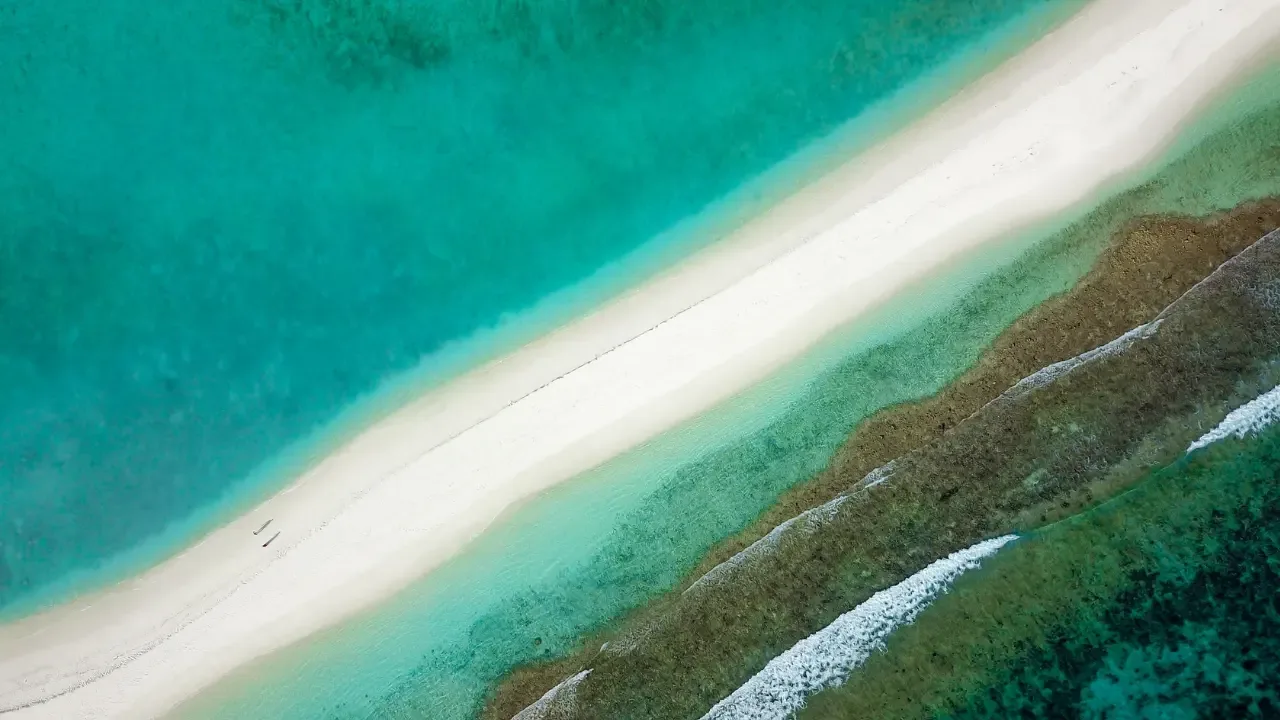
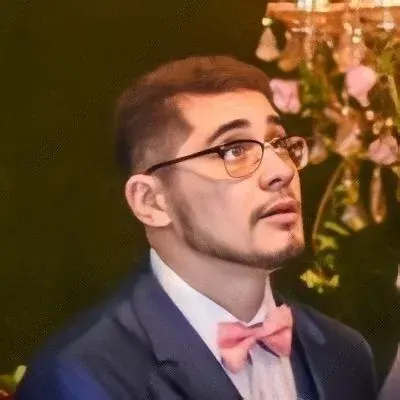
Reshaping a Dataframe from Wide to Long Format 🔄
So you have a dataframe that looks something like this:
Code Country 1950 1951 1952 1953 1954
AFG Afghanistan 20,249 21,352 22,532 23,557 24,555
ALB Albania 8,097 8,986 10,058 11,123 12,246
And you want to transform it into a long format like this:
Code Country Year Value
AFG Afghanistan 1950 20,249
AFG Afghanistan 1951 21,352
AFG Afghanistan 1952 22,532
AFG Afghanistan 1953 23,557
AFG Afghanistan 1954 24,555
ALB Albania 1950 8,097
ALB Albania 1951 8,986
ALB Albania 1952 10,058
ALB Albania 1953 11,123
ALB Albania 1954 12,246
In this blog post, we will explore how to reshape your dataframe using the reshape()
function, since you find it a bit more user-friendly. Let's dive right in!
The Problem: Convert Wide Dataframe to Long Format 😫
Converting a dataframe from wide to long format can be a bit tricky, especially if you're not familiar with the appropriate functions or methodologies. In this case, you want to convert a dataframe with columns representing the years into a format where each row represents an individual observation.
The Solution: Using the reshape()
Function 🚀
The reshape()
function in R is a powerful tool for reshaping dataframes. It allows you to transform your data from one shape to another, including converting from wide to long format.
Here's how you can use the reshape()
function to achieve your desired result:
# Assuming your original dataframe is called 'df'
long_df <- reshape(df,
idvar = c("Code", "Country"),
varying = c("1950", "1951", "1952", "1953", "1954"),
direction = "long",
v.names = "Value",
times = c(1950, 1951, 1952, 1953, 1954),
timevar = "Year")
Let's break down the parameters used in the reshape()
function:
idvar
: Specifies the identifier variables that uniquely identify each observation. In this case, "Code" and "Country" serve as the identifiers.varying
: Indicates the variable names containing the measurements to be reshaped. In this case, it's the years "1950" to "1954".direction
: Specifies the direction of the reshaping. In your case, you want to go from the wide format to the long format, so you'll set it to "long".v.names
: Specifies the name of the column that will contain the reshaped values. Here, we'll call it "Value".times
: Specifies the values to use as the new "Year" column. These will be the individual years within the columns you are reshaping.timevar
: Specifies the name of the new column that will store the times. We'll name it "Year".
By following this approach, you should be able to reshape your dataframe from wide to long format effectively.
Another Option: Using the tidyverse
Package 💡
If you prefer a more concise and intuitive way to reshape your dataframe, you can also use the tidyverse
package. Specifically, the pivot_longer()
function is a handy tool for this task.
library(tidyverse)
long_df <- your_dataframe %>%
pivot_longer(cols = starts_with("19"),
names_to = "Year",
values_to = "Value")
Using the pivot_longer()
function, you can specify the columns (cols
) to pivot based on a pattern, such as column names starting with "19" indicating the years. Then, you define the new column names using names_to
and store the corresponding values in values_to
. This approach provides a cleaner and more intuitive syntax for reshaping your dataframe.
Get Started and Reshape Your Data! 🎉
Now that you have learned two approaches to reshape your dataframe from wide to long format, you can choose the one that suits your preferences. Experiment with the reshape()
function or enjoy the simplicity of the tidyverse
package. Regardless of your choice, reshaping your data will allow you to perform more comprehensive analyses and gain deeper insights.
Give it a try with your dataset and let us know how it goes! Feel free to share your experiences, ask questions, or provide feedback in the comments section below. Happy data reshaping! 😄🔀
Note: Don't forget to modify the code according to your specific dataframe and column names.