Replacing NAs with latest non-NA value
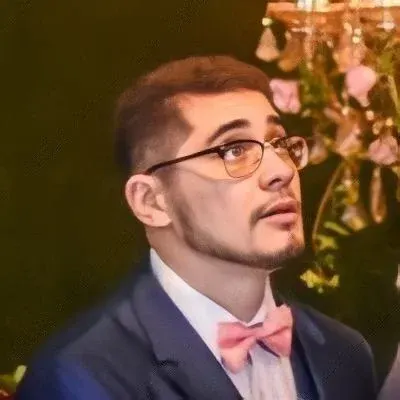
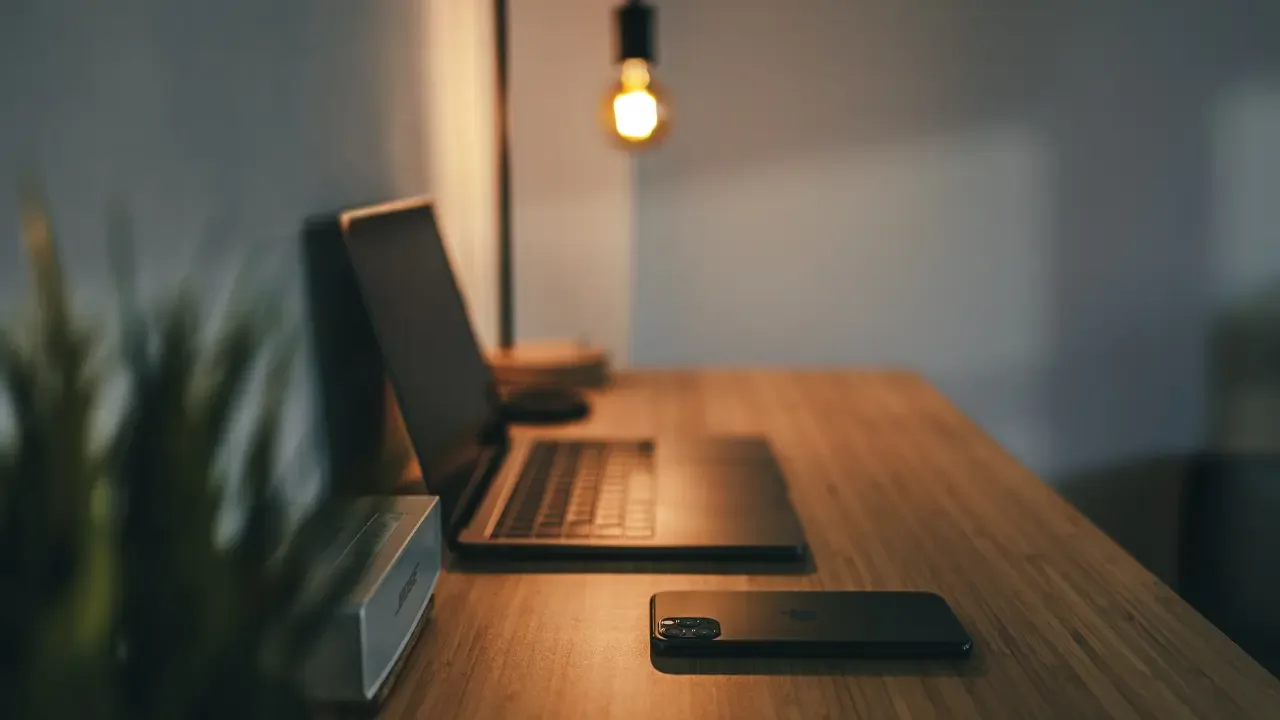
📝 Replacing NAs with latest non-NA value: A Complete Guide
Hey there! 👋 Are you facing the challenge of replacing NAs with the latest non-NA value in R? Don't worry, I got you covered! In this guide, I'll walk you through the common issues people encounter, provide easy solutions, and offer a compelling call-to-action at the end. Let's dive in, shall we? 💪
🧩 The Problem: Filling NAs with the closest previous non-NA value
Imagine you have a data frame or data table in R, and you want to "fill forward" NAs with the closest previous non-NA value. Here's a simple example using vectors:
y <- c(NA, 2, 2, NA, NA, 3, NA, 4, NA, NA)
You want to create a function fill.NAs()
that constructs yy
as follows:
yy
[1] NA NA NA 2 2 2 2 3 3 3 4 4
But here's the catch: you need to repeat this operation for many small-sized data frames (around 30-50 Mb in size) and handle rows where all entries are NAs. So, how can you approach this problem efficiently? Let's find out! 😎
🛠️ The Ugly Solution and Its Drawbacks
The code you provided aims to solve the problem, but you rightly pointed out its ugliness. Here's a snippet of the function fill.NAs()
you cooked up:
last <- function(x) {
x[length(x)]
}
fill.NAs <- function(isNA) {
# ... implementation details ...
}
While it may work, the solution can be hard to follow and lacks elegance. Don't worry; I have some better suggestions for you! 🙌
✨ A Better Approach: Using the zoo package
Instead of reinventing the wheel, we can leverage the power of existing packages. In this case, the zoo
package in R provides a straightforward way to fill NAs with the latest non-NA value. Here's how you can do it:
library(zoo)
y <- c(NA, 2, 2, NA, NA, 3, NA, 4, NA, NA)
yy <- na.locf(y)
And that's it! The na.locf()
function from the zoo
package replaces the NAs with the latest non-NA value, giving you the desired result:
yy
[1] NA NA NA 2 2 2 2 3 3 3 4 4
Now you might be wondering, how can you handle large data frames efficiently? Let's find out! 🚀
🚀 Efficiently Handling Large Data Frames
When dealing with large data frames (around 1 Tb in size), you need an approach that considers performance and memory usage. Here's a step-by-step guide to efficiently handle such cases:
Split your data frame into smaller chunks if possible. This way, you'll avoid overwhelming your system and maintain a higher level of efficiency.
Apply the
na.locf()
function from thezoo
package to each chunk separately.Merge the filled chunks back together into a single data frame using appropriate joining techniques based on your specific use case.
By following these steps, you can process large data frames efficiently and ensure smooth execution without overwhelming your system resources. 🎯
💡 Bonus Tips:
If you encounter rows where all entries are NAs, you can use additional techniques like the
complete.cases()
function to identify and handle those cases separately.Make sure to optimize your code by exploring parallel processing techniques or utilizing the power of distributed computing frameworks like Apache Spark or Hadoop if applicable to your situation.
📣 Your Turn: Share Your Experience and Suggestions!
Now that you have learned how to replace NAs with the latest non-NA value efficiently, I'd love to hear your thoughts! Have you faced any challenges while working with large data frames? Do you have any additional tips or suggestions? Don't hesitate to share your experiences and engage with the community in the comments section below! Let's learn and grow together! 😄
That's a wrap! 🎉 I hope this guide has been useful in helping you solve the problem of replacing NAs with the latest non-NA value in an easy and efficient manner. Remember, next time you encounter such an issue, give the zoo
package a try for quick and elegant solutions.
Until next time, happy coding! 👩💻👨💻
Take Your Tech Career to the Next Level
Our application tracking tool helps you manage your job search effectively. Stay organized, track your progress, and land your dream tech job faster.
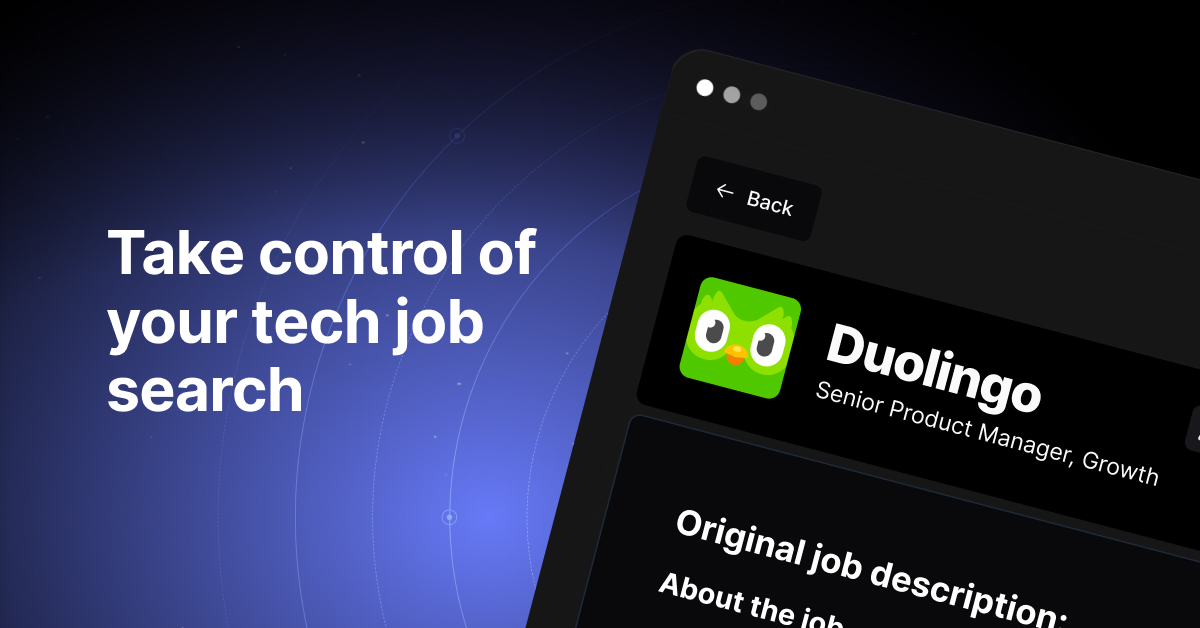