Plotting two variables as lines using ggplot2 on the same graph
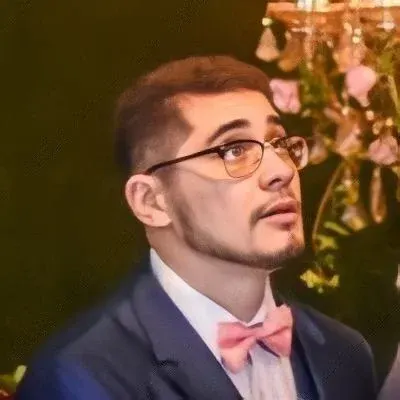

Plotting Two Variables as Lines Using ggplot2: A Complete Guide
š Have you ever wondered how to plot two variables as lines on the same graph using ggplot2? š¤ Don't worry, you're not alone! Many people find this task a bit challenging at first, but once you understand the process, it's a piece of cake! š° In this guide, we'll walk through the steps to plot the time series variables var0
and var1
with the date
on the x-axis, using ggplot2. š
The Data
š Before we dive into the code, let's take a quick look at the data provided:
test_data <- data.frame(
var0 = 100 + c(0, cumsum(runif(49, -20, 20))),
var1 = 150 + c(0, cumsum(runif(49, -10, 10))),
date = seq(as.Date("2002-01-01"), by = "1 month", length.out = 100)
)
š
The test_data
object contains two variables, var0
and var1
, representing time series data, and a date
column. Our goal is to plot var0
and var1
as lines on the same graph, with the x-axis representing the dates.
The Solution
š§ Now, let's jump into the solution using ggplot2! š”
Here's the code to achieve our desired plot:
library(ggplot2)
ggplot(test_data, aes(x = date)) +
geom_line(aes(y = var0, color = "var0")) +
geom_line(aes(y = var1, color = "var1")) +
labs(x = "Date", y = "Value") +
scale_color_manual("Legend",
values = c(var0 = "blue", var1 = "red"),
labels = c(var0 = "Variable 0", var1 = "Variable 1")) +
theme_minimal()
Let's break down what's happening in the code step-by-step:
We first load the ggplot2 package, which is essential for creating the plot.
Using the
ggplot()
function, we specify the data frametest_data
and set the x-axis to thedate
variable.We add two lines to the plot using the
geom_line()
function. Each line is associated with a different variable:var0
andvar1
. We also use thecolor
aesthetic to differentiate the lines.Next, we add axis labels for the x and y axes using the
labs()
function.To create a legend, we use the
scale_color_manual()
function. We specify the colors for each variable and provide labels for the legend entries.Finally, we apply a minimalistic theme using the
theme_minimal()
function to enhance the visual appeal of the plot.
š That's it! You've successfully plotted var0
and var1
as lines on the same graph using ggplot2! š
Troubleshooting and Tips
š ļø If you encounter any issues or want to further customize the plot, here are a few troubleshooting tips:
Colors: If you want to change the colors of the lines, modify the
values
argument in thescale_color_manual()
function. You can use standard color names or HEX color codes.Line Style: You can add additional arguments to the
geom_line()
function to control the line style, such aslinetype
orsize
.Date Formatting: If you want to change the date format on the x-axis, you can use the
scale_x_date()
function. This function allows you to specify the format of the dates using thedate_labels
argument.
Share Your Results!
š£ We'd love to see the plots you create with this guide! Feel free to share your results in the comments section below or on social media using the hashtag #ggplot2Lines. š
If you found this guide helpful or have any further questions, don't hesitate to leave a comment. Let's keep the conversation going! š¤©
Happy plotting! šØš
Take Your Tech Career to the Next Level
Our application tracking tool helps you manage your job search effectively. Stay organized, track your progress, and land your dream tech job faster.
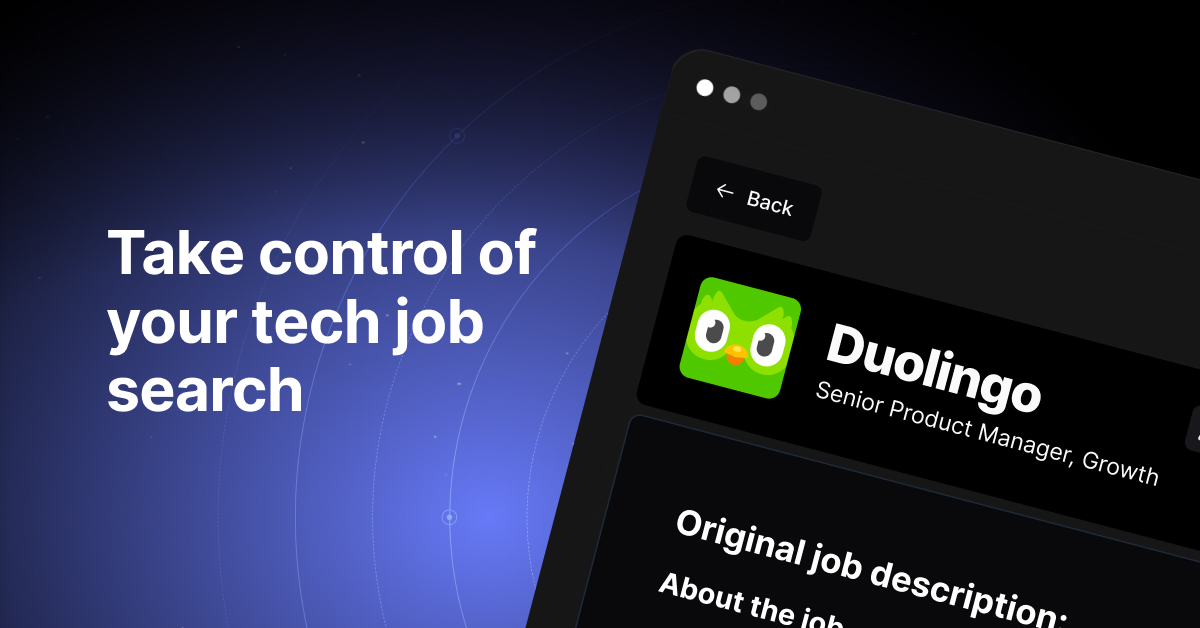